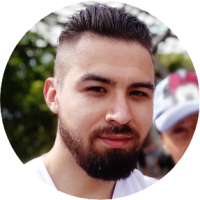
Topics
Replies
whis.gg
25 Feb 2017, 09:58
( Updated at: 15 Jan 2024, 14:51 )
@Stopware Team
It's been nearly 3 weeks when this serious bug was reported first time. How long is it going to take to fix it? Why did you made the new parameter window when the old one was working alright? Instead you could invest the time into something that traders have voted for.
Here are all reports of the bug, please fix it already.
/forum/indicator-support/11162
/forum/indicator-support/11263
[/forum/ctrader-support/11261]
@whis.gg
whis.gg
18 Feb 2017, 13:56
Hi,
See this thread: /forum/whats-new/1463
If you want someone to code the indicator you should write into Jobs section or contact Consultants directly.
I am available to code it, contact me via email if you want a quote.
Kind Regards,
Jiri
@whis.gg
whis.gg
16 Feb 2017, 20:30
This should do, haven't tested though.
using cAlgo.API; using cAlgo.API.Internals; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleCloseAllPositionsAsync : Robot { [Parameter(DefaultValue = 100, MinValue = 1)] public int NumberOfPositions { get; set; } protected override void OnStart() { for (int i = 0; i < NumberOfPositions; i++) { ExecuteMarketOrderAsync(TradeType.Buy, Symbol, Symbol.VolumeMin); } } protected override void OnBar() { CloseAllPositionsAsync(); Stop(); } private void CloseAllPositionsAsync() { int lastIndex = Positions.Count - 1; for (int index = 0; index <= lastIndex; index++) { if (index != lastIndex) { ClosePositionAsync(Positions[index]); } else { TradeResult result = ClosePosition(Positions[index]); if (result.IsSuccessful) { if (Positions.Count > 0) { CloseAllPositionsAsync(); } } else { if (result.Error == ErrorCode.MarketClosed) { // market closed } else if (result.Error == ErrorCode.Disconnected) { // disconnected } else { // other error } } } } } protected override void OnStop() { Print(Positions.Count); } } }
@whis.gg
whis.gg
16 Feb 2017, 20:13
Not sure if it's best solution but it's working. It might get looped if market is closed or you lost the internet connection - you should add a check if there is an error and break the loop.
using cAlgo.API; using cAlgo.API.Internals; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleCloseAllPositionsAsync : Robot { [Parameter(DefaultValue = 100, MinValue = 1)] public int NumberOfPositions { get; set; } protected override void OnStart() { for (int i = 0; i < NumberOfPositions; i++) { ExecuteMarketOrderAsync(TradeType.Buy, Symbol, Symbol.VolumeMin); } } protected override void OnBar() { CloseAllPositionsAsync(); Stop(); } private void CloseAllPositionsAsync() { int lastIndex = Positions.Count - 1; for (int index = 0; index <= lastIndex; index++) { if (index != lastIndex) { ClosePositionAsync(Positions[index]); } else { ClosePosition(Positions[index]); CheckIfAllPositionsAreClosed(); } } } private void CheckIfAllPositionsAreClosed() { if (Positions.Count > 0) { CloseAllPositionsAsync(); } } protected override void OnStop() { Print(Positions.Count); } } }
@whis.gg
whis.gg
16 Feb 2017, 15:54
int index = MarketSeries.Open.Count - 1; double candleSize = MarketSeries.High[index] - MarketSeries.Low[index]; double bodySize = Math.Abs(MarketSeries.Open[index] - MarketSeries.Close[index]); double percent = bodySize * 100 / candleSize; if (percent > 50) { // the body size is greater than 50% of the candle }
You mean like that?
@whis.gg
whis.gg
07 Feb 2017, 11:57
RE:
Spotware said:
Hi there,
Thanks for your post in our forum. I am sorry that you find this situation frustrating.
Allow us to explain and perhaps you may understand and respect our position. We have a team dedicated to support brokers, because we are a B2B company and they are our clients. Our current team can handle up to 100 brokers (100 entities). Our team cannot handle 300,000 traders (300,000 entities). The fees we charge brokers reflect the requirement to support a single entity only, not their thousands upon thousands of clients.
In a typical supply chain situation we would be considered a wholesaler or even manufacturer. Our fees to brokers are a fraction of what they are to end users, becuase they have large overheads - such as large support teams.
Brokers are responsible for supporting their clients (you) and Spotware (us) are responsible for supporting them.
PS - we screen calls and have a white list of number who are our clients.
I hope this explanation is well received.
Regards,
Spotware Team
Are you saying that Spotware Team is focused into cBroker development and support only? You should consider changing your signature then, "TRADERS FIRST™ Vote for your favorite features: http://vote.spotware.com/" is little bit outdated (over 3 years already), don't you think?
@whis.gg
whis.gg
01 Feb 2017, 23:34
Let me make few things clear. the Calculate(int index) method that you override in the indicator is called on each bar (index) starting from zero - first bar on the chart (historical) up to current bar and then it's called on each price change during live market, therefore it's most likely going to be called multiple times with same index and you might call it repainting at latest bar.
When you are getting series from different timeframe the index values are not equal to your chart timeframe. Therefore you always need to find associated index between both series. That's the reason why indicator acts slightly different on historical and live data. The built-in function GetIndexBy(Exact)Time will know "future" close of that candle, because it's already closed when retrieved in the history. During live market it's going to return you current close value, but this value might change until new bar opens.
The variable called lastIdx is just holding last index of custom market series and checks for new bar (higher index) - it's true only when new bar opens.
The loops I used are both different. In first solution it's repainting historical values of the indicator Result in order to duplicate historical chart behaviour. The second solution is just setting NaN values of all previous candles that are not equal to close time of custom timeframe so it doesn't plot flat line anymore but connects points only.
Essentially you want to make the source indicator not to be repainting at all or delegate an event which would hold indexes that were repainted as args and subscribe to it in the indicator that's using it. Then you can force the indicator to recalculate certain indexes as well.
@whis.gg
whis.gg
01 Feb 2017, 14:36
This is essentially what I’m after, or what I wish to accomplish: creating lines using the other timeframe data points.
You should make a method that would do the work rather than complicating it by using moving average. See the solution below.
using cAlgo.API; using cAlgo.API.Internals; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class PricePoints : Indicator { [Parameter("Timeframe", DefaultValue = "Minute3")] public TimeFrame CustomTimeFrame { get; set; } [Output("Main")] public IndicatorDataSeries Result { get; set; } private MarketSeries ms; private int lastIdx = 0; protected override void Initialize() { ms = MarketData.GetSeries(CustomTimeFrame); } public override void Calculate(int index) { var idx = ms.OpenTime.GetIndexByExactTime(MarketSeries.OpenTime[index]); if (idx > lastIdx) { Result[index - 1] = ms.Close[idx - 1]; lastIdx = idx; } //else //{ // Result[index] = MarketSeries.Close[index]; // for (int i = MarketSeries.OpenTime.GetIndexByExactTime(ms.OpenTime[lastIdx]); i < index; i++) // { // Result[i] = double.NaN; // } //} } } }
Commented area is plotting last bar that is still in the progress.
@whis.gg
whis.gg
01 Feb 2017, 13:53
You can't force other indicators referencing to this to repaint as well but here is the solution the indicator itself.
using cAlgo.API; using cAlgo.API.Internals; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class PricePoints : Indicator { [Parameter("Timeframe", DefaultValue = "Minute3")] public TimeFrame CustomTimeFrame { get; set; } [Output("Main")] public IndicatorDataSeries Result { get; set; } private MarketSeries ms; private int lastIdx; protected override void Initialize() { ms = MarketData.GetSeries(CustomTimeFrame); } public override void Calculate(int index) { var idx = ms.OpenTime.GetIndexByTime(MarketSeries.OpenTime[index]); if (idx != lastIdx) { Result[index] = ms.Close[idx]; lastIdx = idx; } else { for (int i = MarketSeries.OpenTime.GetIndexByExactTime(ms.OpenTime[idx]); i <= index; i++) { Result[i] = ms.Close[lastIdx]; } } } } }
@whis.gg
whis.gg
01 Feb 2017, 10:08
Hi John, you are reading close value of 3-minute chart every minute. Therefore it returns you close value of a bar that is still in the progress. You would need to repaint previous values of all indexes that are within one 3-minute chart bar in order to achieve flat lines as in the history where you can read only OHLC values of closed bars. I hope it makes any sense.
@whis.gg
whis.gg
18 Dec 2016, 17:32
Hi Simon.
Calculate(int index) is called on each price change (each tick). The method that you have written to call OnBarClosed(int index) is called only at opening of new bar (new index) and passes previous index into the method. Therefore the indicator has always unassigned last value because its waiting for the close (opening of new bar).
I suppose you are trying to access last value of the indicator, that's why you get the NaN value.
@whis.gg
whis.gg
15 Dec 2016, 19:41
RE:
But i debugged the code and when i call MarketData.GetSeries for the current bot timeframe (i know the current data is also in .MarketSeries, but the bot is still under construction, so far away from release :) ) it returns the same marketdata like in backtesting, that is in this example, 125 hour-bars before the starttime-period. But when i call MarketData.GetSeries for any other timeframe than the current one i get zero data which is not really good because i need also past data from other timeframes.
Yes, I was talking about series obtained via GetSeries() method. The series of current chart timeframe are always loaded with historical data.
Thank you very much, that is really nice of you. Unfortunately i am developing this bot already a long time and it consists of 4 different projects, so i hope you understand that i do not want to give my "baby away" ;). But like i said, that is very very nice of you. Thanks.
I understand. I am not here to steal your robot or anything similar to this. It would just make helping you much easier.
@whis.gg
whis.gg
27 Feb 2017, 02:34
Hi, Time Series Moving Average is equal to Linear Regression Forecast. The cAlgo platform calculates it by multiplying Linear Regression Slope with Period plus Linear Regression Intercept. Here's is better solution that gives you same result.
@whis.gg