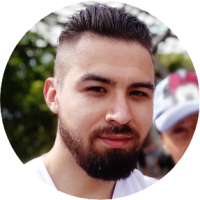
Topics
Replies
whis.gg
02 Feb 2016, 15:04
Build this cAlgo and run on 1 minute timeframe. It should send you testing email each time candle opens with opening time in the body of the email.
using System; using cAlgo.API; namespace cAlgo { [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class TestMail : Indicator { [Parameter("Address", DefaultValue = "your@email.com")] public string Address { get; set; } private int lastCounted; public override void Calculate(int index) { if (!IsLastBar) { lastCounted = MarketSeries.Close.Count; return; } bool isNewBar = MarketSeries.Close.Count > lastCounted; if (isNewBar) { var time = DateTime.UtcNow; string body = "New candle was opened in " + time.ToString("MMMM dd, yyyy") + " at " + time.ToString("HH:mm" + " (UTC)."); Notifications.SendEmail(Address, Address, "TestMail cAlgo", body); lastCounted = MarketSeries.Close.Count; } } } }
If it won't work, then there is a problem with email settings and not the indicator.
@whis.gg
whis.gg
02 Feb 2016, 14:33
RE:
Does that work?
LineStyle lineStyle; Colors color; if (DateTime.UtcNow.DayOfWeek == DayOfWeek.Monday) { lineStyle = LineStyle.Solid; color = Colors.Red; } else { lineStyle = LineStyle.Lines; color = Colors.Blue; }
Or short version.
bool isMonday = DateTime.UtcNow.DayOfWeek == DayOfWeek.Monday; var lineStyle = isMonday ? LineStyle.Solid : LineStyle.Lines; var color = isMonday ? Colors.Red : Colors.Blue;
@whis.gg
whis.gg
31 Jan 2016, 17:14
Use this code instead.
using cAlgo.API; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class Note : Indicator { [Parameter("Note", DefaultValue = "Template name")] public string Text { get; set; } protected override void Initialize() { ChartObjects.DrawText("note", Text, StaticPosition.TopRight, Colors.Gray); } public override void Calculate(int index) { return; } } }
@whis.gg
whis.gg
31 Jan 2016, 17:10
( Updated at: 21 Dec 2023, 09:20 )
RE:
Is there any way to mark templates so when I apply it it shows name on chart ?
You can make simple indicator which would show a text on the chart. Then you can put it into each template and save it.
using cAlgo.API; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class Note : Indicator { [Parameter("Note", DefaultValue = "Template name")] public string Text { get; set; } protected override void Initialize() { ChartObjects.DrawText("note", Text, StaticPosition.TopRight, Colors.Gray); } public override void Calculate(int index) { return; } } }
How can I delete custom indicators from scrolling list , not from chart? I downloaded many and the list is just too long .
There are 2 options.
- Delete it from cAlgo platform.
- Delete cAlgo from Indicators folder. %userprofile%\Documents\cAlgo\Sources\Indicators
@whis.gg
whis.gg
30 Jan 2016, 15:18
( Updated at: 21 Dec 2023, 09:20 )
You would need to add outputs on Heiken Ashi (OHLC values) and make NonlagDot able to get custom data source (HA values). Then when you are loading indicator you choose HA as source data.
Sample of using Moving Average on Renko Chart.
@whis.gg
whis.gg
27 Jan 2016, 22:12
Hey MaVe,
Take a look at this, I think it does what you want. :)
using System; using cAlgo.API; using cAlgo.API.Internals; namespace cAlgo.Indicators { [Indicator(IsOverlay = true, AutoRescale = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class DailyHighLow : Indicator { [Parameter("Days To Show", DefaultValue = 3)] public int DaysToShow { get; set; } [Parameter("Extend (Days)", DefaultValue = 2)] public int ExtendInDays { get; set; } private MarketSeries daily; protected override void Initialize() { daily = MarketData.GetSeries(TimeFrame.Daily); } public override void Calculate(int index) { DateTime today = DateTime.UtcNow.Date; for (int i = 0; i < DaysToShow; i++) { DateTime startOfDay = today.AddDays(-i); DateTime endOfDay = today.AddDays(1 - i); var high = daily.High.Last(i); var low = daily.Low.Last(i); ChartObjects.DrawLine("DailyHigh" + i, startOfDay, high, endOfDay, high, Colors.Yellow, 1); ChartObjects.DrawLine("DailyLow" + i, startOfDay, low, endOfDay, low, Colors.Yellow, 1); ChartObjects.DrawLine("DailyHighExtended" + i, endOfDay, high, endOfDay.AddDays(ExtendInDays), high, Colors.Green, 1, LineStyle.Lines); ChartObjects.DrawLine("DailyLowExtended" + i, endOfDay, low, endOfDay.AddDays(ExtendInDays), low, Colors.Red, 1, LineStyle.Lines); } } } }
@whis.gg
whis.gg
23 Jan 2016, 10:59
Hi!
What about something like that. It's just pseudo-code but you should get the idea. :)
private bool isNewCandle = true; private TimeSeries openTime; private TimeSpan timeFrame = MarketSeries.TimeFrame.ToTimeSpan(); // This method doesn't exit. You need to write one. public override void Calculate(int index) { if (isNewCandle) { SetValuesToZero(); // Sets your values to zero. openTime = MarketSeries.OpenTime.LastValue; isNewCandle = false; } if (Time > openTime + timeFrame) { isNewCandle = true; } }
@whis.gg
whis.gg
20 Jan 2016, 15:56
Could you provide your method where you use SendEmail?
Make sure you use correct settings before trying to send an email notification. You can do that in Preferences -> Email Settings.
Example how to use it correctly:
string fromAddress = "belochjiri@hotmail.com"; //Sender's Address string toAdress = "belochjiri@hotmail.com"; // Recipient's Address string subject = "Testing email"; //Email Subject string text = "This email was sent from cAlgo."; //Email Body protected override void OnStart() { SendEmail(fromAdress, toAddress, subject, text); }
@whis.gg
whis.gg
19 Jan 2016, 17:05
RE:
This documentation might help.
If you want to implement it in your cAlgo, it's built-in already.
Example:
private LinearRegressionForecast lrForecast; protected override void OnStart() { lrForecast = Indicators.LinearRegressionForecast(Source, Period); } protected override void OnTick() { Print("LRF Last Value = {0}", lrForecast.Result.LastValue); }
I hope it helped.
@whis.gg
whis.gg
02 Feb 2016, 15:09
RE:
MaVe said:
It's not. I need the exact formula of calculations in order to achieve same values.
@whis.gg