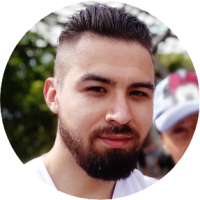
Topics
Replies
whis.gg
07 Oct 2016, 14:27
Hi, you need to put the class in same namespace. Then you should send the algo as parameter in which it should be printed. See sample below.
using cAlgo.API; using cAlgo.API.Internals; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class NewcBot : Robot { [Parameter(DefaultValue = 0.0)] public double Parameter { get; set; } private TestClass testClass; protected override void OnStart() { testClass = new TestClass(); } protected override void OnTick() { testClass.Test(this); } } public class TestClass { private int x = 4; public void Test(Algo algo) { algo.Print(x); } } }
If you need any further help, feel free to contact me via email.
@whis.gg
whis.gg
14 Sep 2016, 04:50
using System; using cAlgo.API; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class CustomTF : Indicator { [Parameter("Period", DefaultValue = 1, MinValue = 1)] public int Period { get; set; } [Parameter("Wick Thickness", DefaultValue = 1, MinValue = 1)] public int WickThickness { get; set; } [Parameter("Body Thickness", DefaultValue = 3, MinValue = 1)] public int BodyThickness { get; set; } [Parameter("Bullish Color", DefaultValue = "SeaGreen")] public string ColorBull { get; set; } [Parameter("Bearish Color", DefaultValue = "Tomato")] public string ColorBear { get; set; } private Colors colorBull, colorBear; protected override void Initialize() { Enum.TryParse<Colors>(ColorBull, out colorBull); Enum.TryParse<Colors>(ColorBear, out colorBear); } public override void Calculate(int index) { int idx1 = index - (index % (Period)); int idx2 = idx1 + (index - idx1) / 2; int x = idx2 - idx1; double open = (MarketSeries.Open[idx1] + MarketSeries.Close[idx1 - 1]) / 2; double high = Functions.Maximum(MarketSeries.High, index - idx1 + 1); double low = Functions.Minimum(MarketSeries.Low, index - idx1 + 1); double close = MarketSeries.Close[index]; var color = close > open ? colorBull : colorBear; ChartObjects.DrawLine("Wick" + idx1, idx2, high, idx2, low, color, WickThickness, LineStyle.Solid); ChartObjects.DrawLine("Body" + idx1, idx2, open, idx2, close, color, BodyThickness, LineStyle.Solid); } } }
@whis.gg
whis.gg
14 Sep 2016, 04:49
using System; using cAlgo.API; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class CustomTF : Indicator { [Parameter("Period", DefaultValue = 1, MinValue = 1)] public int Period { get; set; } [Parameter("Wick Thickness", DefaultValue = 1, MinValue = 1)] public int WickThickness { get; set; } [Parameter("Body Thickness", DefaultValue = 3, MinValue = 1)] public int BodyThickness { get; set; } [Parameter("Bullish Color", DefaultValue = "SeaGreen")] public string ColorBull { get; set; } [Parameter("Bearish Color", DefaultValue = "Tomato")] public string ColorBear { get; set; } private Colors colorBull, colorBear; protected override void Initialize() { Enum.TryParse<Colors>(ColorBull, out colorBull); Enum.TryParse<Colors>(ColorBear, out colorBear); } public override void Calculate(int index) { int idx1 = index - (index % (Period)); int idx2 = idx1 + (index - idx1) / 2; int x = idx2 - idx1; double open = (MarketSeries.Open[idx1] + MarketSeries.Close[idx1 - 1]) / 2; double high = Functions.Maximum(MarketSeries.High, index - idx1 + 1); double low = Functions.Minimum(MarketSeries.Low, index - idx1 + 1); double close = MarketSeries.Close[index]; var color = close > open ? colorBull : colorBear; ChartObjects.DrawLine("Wick" + idx1, idx2, high, idx2, low, color, WickThickness, LineStyle.Solid); ChartObjects.DrawLine("Body" + idx1, idx2, open, idx2, close, color, BodyThickness, LineStyle.Solid); } } }
This indicator plots chart X times bigger than original. Therefore if you attach it on t34 chart with period of 5, you will get t170 chart.
I hope it helps.
@whis.gg
whis.gg
31 Jul 2016, 21:56
RE:
ChasBrownTH said:
re.Romanov Capital 'this can be done from within an indicator or cBot easily.' Can you please explain how?
I need to store data at specific times and it would be wonderful to just click a chart then have my Bot write to my database at that exact moment.
Making robot which would do the action and then stop is not an option? You would attach it to chart and whenever you hit play button it does the action.
@whis.gg
whis.gg
31 Jul 2016, 13:20
RE:
tmc. said:
You can download cAlgo platform, make new cBot, leave its logic empty, make new instance and run backtest for desired period of time. That way you get chart for selected period of time and can do analysis there.
Additionally, once the chart is loaded you can attach your indicators to the chart, if you use any.
@whis.gg
whis.gg
23 Jun 2016, 12:47
It's not a bug, stop loss and take profit haven't supported decimal places, so it always rounds to whole number. However, there is a workaround - see the sample code below.
using System; using cAlgo.API; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class Sample : Robot { [Parameter(DefaultValue = 5.5)] public double StopLossPips { get; set; } [Parameter(DefaultValue = 8.1)] public double TakeProfitPips { get; set; } protected override void OnStart() { ExecuteMarketOrderAsync(TradeType.Sell, Symbol, Symbol.VolumeMin, "label"); Positions.Opened += OnPositionsOpened; } private void OnPositionsOpened(PositionOpenedEventArgs obj) { var position = obj.Position; if (position.Label == "label") { int direction = position.TradeType == TradeType.Buy ? 1 : -1; double stopLoss = position.EntryPrice - StopLossPips * Symbol.PipSize * direction; double takeProfit = position.EntryPrice + TakeProfitPips * Symbol.PipSize * direction; ModifyPosition(position, stopLoss, takeProfit); } } } }
@whis.gg
whis.gg
22 Jun 2016, 21:39
Add this anywhere in the class.
protected override void OnTick() { foreach (var position in Positions.FindAll("SampleRSI", Symbol)) { if (position.StopLoss != position.EntryPrice) { if (position.Pips >= 50) { ModifyPosition(position, position.EntryPrice, position.TakeProfit); } } } }
@whis.gg
whis.gg
21 Jun 2016, 17:46
Welcome in cTrader community. Here you go: /algos/indicators/show/1326
@whis.gg
whis.gg
21 Jun 2016, 14:44
Hi, this method returns you how many bars back was the highest or lowest bar within a period.
private int HighestBar(DataSeries series, int period) { for (int i = 0; i <= period; i++) { if (series[series.Count - 1 - i] == series.Maximum(period)) { return i; } } return -1; } private int LowestBar(DataSeries series, int period) { for (int i = 0; i <= period; i++) { if (series[series.Count - 1 - i] == series.Minimum(period)) { return i; } } return -1; }
@whis.gg
whis.gg
18 Jun 2016, 17:23
RE:
kaliszczak1991 said:
Is there an option to set that robot to works only in some hours, lets say during London session?
Have a look at this thread: /forum/calgo-reference-samples/543
@whis.gg
whis.gg
06 Nov 2016, 22:25
Hi Mikro,
You could use Linq to check if collection of current opened positions contain desired position. See the sample below.
@whis.gg