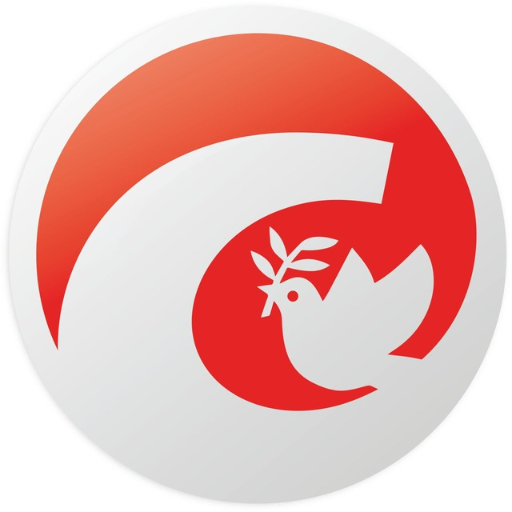
Topics
Replies
Spotware
15 Jan 2014, 16:21
using cAlgo.API; namespace cAlgo.Robots { [Robot] public class SampleBreakEven:Robot { private TradeType _tradeType; [Parameter("Trigger (pips)", DefaultValue = 20)] public int Trigger { get; set; } [Parameter("Buy", DefaultValue = true)] public bool Buy { get; set; } [Parameter("Volume", DefaultValue = 10000, MinValue = 10000)] public int Volume { get; set; } [Parameter(DefaultValue = 10)] public double TakeProfitPips { get; set; } [Parameter(DefaultValue = 10)] public double StopLossPips { get; set; } [Parameter(DefaultValue = "Break Even cBot")] public string MyLabel { get; set; } protected override void OnStart() { _tradeType = Buy ? TradeType.Buy : TradeType.Sell; var result = ExecuteMarketOrder(_tradeType, Symbol, Volume, MyLabel, StopLossPips, TakeProfitPips); if(!result.IsSuccessful) { Print("Stopping cBot due to failure executing market order"); Stop(); } } protected override void OnTick() { var position = Positions.Find(MyLabel, Symbol, _tradeType); if(position == null) { Print("Position not found. Stopping cBot"); Stop(); return; } var entryPrice = position.EntryPrice; var distance = _tradeType == TradeType.Buy ? Symbol.Bid - entryPrice : entryPrice - Symbol.Ask; if (distance >= Trigger*Symbol.PipSize) { ModifyPosition(position, entryPrice, position.TakeProfit); Print("Stop Loss to Break Even set for position {0}", position.Id); Stop(); } } } }
@Spotware
Spotware
15 Jan 2014, 12:37
// ------------------------------------------------------------------------------------------------- // // The Labels Robot demonstrates the use of Label to differentiate positions opened by a robot // // ------------------------------------------------------------------------------------------------- using cAlgo.API; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC)] public class LabelsSample : Robot { [Parameter("Buy", DefaultValue = true)] public bool TradeTypeBuy { get; set; } [Parameter(DefaultValue = "My Label 1")] public string MyLabel { get; set; } [Parameter(DefaultValue = 100000)] public int Volume { get; set; } [Parameter("Stop Loss (pips)", DefaultValue = 100)] public int StopLoss { get; set; } [Parameter("Take Profit (pips)", DefaultValue = 100)] public int TakeProfit { get; set; } [Parameter(DefaultValue = 2)] public int MaxPositions { get; set; } protected TradeType cBotTradeType { get { return TradeTypeBuy ? TradeType.Buy : TradeType.Sell; } } protected override void OnStart() { Positions.Opened += PositionsOnOpened; } private void PositionsOnOpened(PositionOpenedEventArgs args) { var position = args.Position; Print( position.Label == MyLabel ? "Position opened by LabelsSample cBot at {0}" : "Position opened manually at {0}", position.EntryPrice); } protected override void OnBar() { if (Positions.Count < MaxPositions) { ExecuteMarketOrder(cBotTradeType, Symbol, Volume, MyLabel, StopLoss, TakeProfit); } } } }
@Spotware
Spotware
15 Jan 2014, 12:19
// ------------------------------------------------------------------------------------------------- // // This code is a demonstration of the different syntax that can be used to print messages to the log // // ------------------------------------------------------------------------------------------------- using cAlgo.API; namespace cAlgo.Indicators { [Indicator] public class PrintToLog:Indicator { protected override void Initialize() { Print("Initializing PrintToLog Indicator"); } public override void Calculate(int index) { Print("index {0}", index); var close = MarketSeries.Close.LastValue; var high = MarketSeries.High.LastValue; var low = MarketSeries.Low.LastValue; Print("Current close {0}, high {1}, low {2}", close, high, low); Print("Account info:"); Print(Account.Balance); Print(Account.Equity); Print(Account.Margin); Print("Open Positions: " + Positions.Count + "position(s)"); Print("Pending Orders: " + PendingOrders.Count + "order(s)"); } } }
@Spotware
Spotware
15 Jan 2014, 12:10
// --------------------------------------------------------------------------------------- // // This cBot is intended to demonstrate the use of Notifications // // The "Sell Order Notification Robot" will execute a sell market order upon start up with SL and TP. // On the next tick after each position is closed it will execute another and send a notification. // // --------------------------------------------------------------------------------------- using cAlgo.API; namespace cAlgo.Robots { [Robot] public class SellOrderNotification : Robot { [Parameter(DefaultValue = "C:/Windows/Media/notify.wav")] public string SoundFile { get; set; } [Parameter(DefaultValue = 10000, MinValue = 1000)] public int Volume { get; set; } [Parameter(DefaultValue = "Sell Order Robot")] public string cBotLabel { get; set; } [Parameter("Stop Loss", DefaultValue = 10, MinValue = 1)] public int StopLossPips { get; set; } [Parameter("Take Profit", DefaultValue = 10, MinValue = 1)] public int TakeProfitPips { get; set; } protected override void OnStart() { var result = ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, cBotLabel, StopLossPips, TakeProfitPips); if(result.IsSuccessful) { var position = result.Position; Print("Position {0} opened at {1}", position.Label, position.EntryPrice); SendNotifications(position); } } private void SendNotifications(Position position) { var emailBody = string.Format("{0} Order Created at {1}", position.TradeType, position.EntryPrice); Notifications.SendEmail("from@somewhere.com", "to@somewhere.com", "Order Created", emailBody); Notifications.PlaySound(SoundFile); } protected override void OnTick() { var position = Positions.Find(cBotLabel); if (position != null) return; var result = ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, cBotLabel, StopLossPips, TakeProfitPips); if (result.IsSuccessful) { position = result.Position; SendNotifications(position); } } } }
@Spotware
Spotware
15 Jan 2014, 11:49
// --------------------------------------------------------------------------------------- // // This code demonstrates use of ChartObjects // It shows the maximum of a DataSeries for a period. // // --------------------------------------------------------------------------------------- using System; using cAlgo.API; namespace cAlgo.Indicators { [Indicator(IsOverlay = true)] public class ChartObjectsSample : Indicator { [Parameter("Source")] public DataSeries Source { get; set; } [Parameter("Period", DefaultValue = 30, MinValue = 3)] public int Period { get; set; } [Parameter("Text Color", DefaultValue = "Yellow")] public string TextColor { get; set; } [Parameter("Line Color", DefaultValue = "White")] public string LineColor { get; set; } private Colors _colorText; private Colors _colorLine; protected override void Initialize() { // Parse color from string if (!Enum.TryParse(TextColor, out _colorText)) { ChartObjects.DrawText("errorMsg1", "Invalid Color for Text", StaticPosition.TopLeft, Colors.Red); _colorText = Colors.Yellow; } if (!Enum.TryParse(LineColor, out _colorLine)) { ChartObjects.DrawText("errorMsg2", "\nInvalid Color for Line", StaticPosition.TopLeft, Colors.Red); _colorLine = Colors.White; } } public override void Calculate(int index) { if (!IsLastBar) return; ChartObjects.DrawVerticalLine("vLine", index - Period, _colorLine); int maxIndex=index; double max = Source[index]; for (int i = index - Period; i <= index; i++) { if (max >= Source[i]) continue; max = Source[i]; maxIndex = i; } var text = "max " + max.ToString("0.0000"); var top = VerticalAlignment.Top; var center = HorizontalAlignment.Center; ChartObjects.DrawText("max", text, maxIndex, max, top, center, _colorText); ChartObjects.DrawLine("line", maxIndex, max, index, Source[index], _colorLine); } } }
@Spotware
Spotware
14 Jan 2014, 16:06
// ------------------------------------------------------------------------------------------------- // // The Starter cBot will execute a buy order on start // On each tick it will check the net profit and if it reached a predefined value // it will close the position and stop. // // ------------------------------------------------------------------------------------------------- using System; using cAlgo.API; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC)] public class Starter_cBot : Robot { [Parameter(DefaultValue = 10000)] public int Volume { get; set; } [Parameter(DefaultValue = 10)] public double Profit { get; set; } [Parameter(DefaultValue = 10)] public double StopLossPips { get; set; } [Parameter(DefaultValue = "My Robot")] public string MyLabel { get; set; } protected override void OnStart() { ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, MyLabel, StopLossPips, null); } protected override void OnTick() { var position = Positions.Find(MyLabel, Symbol, TradeType.Buy); if(position == null) { Stop(); return; } if (position.NetProfit > Profit) { ClosePosition(position); Stop(); } } } }
@Spotware
Spotware
14 Jan 2014, 14:40
// --------------------------------------------------------------------------------------- // // In "LimitOrder" cBot places a limit order on start, with a label // representing the robot, symbol and timeframe of the instance. // On each tick it checks if the order has been filled. // // --------------------------------------------------------------------------------------- using cAlgo.API; namespace cAlgo.Robots { [Robot] public class LimitOrder : Robot { private bool _filled; [Parameter(DefaultValue = "LimitOrder cBot")] public string RobotLabel { get; set; } [Parameter(DefaultValue = 10)] public int Target { get; set; } [Parameter(DefaultValue = 10000, MinValue = 1000)] public int Volume { get; set; } [Parameter(DefaultValue = true)] public bool Buy { get; set; } [Parameter(DefaultValue = 10)] public double TakeProfitPips { get; set; } [Parameter(DefaultValue = 10)] public double StopLossPips { get; set; } protected string MyLabel { get { return string.Format("{0} {1} {2}", RobotLabel, Symbol.Code, TimeFrame); } } protected TradeType TradeType { get { return Buy ? TradeType.Buy : TradeType.Sell; } } protected override void OnStart() { double targetPrice = TradeType == TradeType.Buy ? Symbol.Ask - Target*Symbol.PipSize : Symbol.Bid + Target*Symbol.PipSize; PlaceLimitOrder(TradeType, Symbol, Volume, targetPrice, MyLabel, StopLossPips, TakeProfitPips); } protected override void OnTick() { if (_filled) return; var position = Positions.Find(MyLabel); if (position == null) return; _filled = true; Print("Limit Order Filled: ID = {0}, Entry Price = {1}, Label = {2}", position.Id, position.EntryPrice, position.Label); } } }
@Spotware
Spotware
14 Jan 2014, 12:59
// The cBot when started will execute an order, setting Take Profit and Stop Loss. // In the event that the position opens it prints a message to the log. // In the event that the position closes it prints a message to the log and executes another order. using System; using cAlgo.API; namespace cAlgo.Robots { [Robot()] public class SampleBuyOrder : Robot { [Parameter("Buy", DefaultValue = true)] public bool TradeTypeBuy { get; set; } [Parameter(DefaultValue = 10000)] public int Volume { get; set; } [Parameter(DefaultValue = "myLabel")] public string cBotLabel { get; set; } [Parameter(DefaultValue = 10)] public double TakeProfitPips { get; set; } [Parameter(DefaultValue = 10)] public double StopLossPips { get; set; } protected TradeType cBotTradeType { get { return TradeTypeBuy ? TradeType.Buy : TradeType.Sell; } } protected override void OnStart() { Positions.Opened += OnPositionsOpened; Positions.Closed += OnPositionsClosed; ExecuteMarketOrder(cBotTradeType, Symbol, Volume, cBotLabel, StopLossPips, TakeProfitPips); } protected void OnPositionsOpened(PositionOpenedEventArgs args) { var position = args.Position; if (position.Label == cBotLabel) Print("Position opened by cBot"); } private void OnPositionsClosed(PositionClosedEventArgs args) { var position = args.Position; if (position.Label == cBotLabel) { Print("Position closed by cBot"); ExecuteMarketOrder(cBotTradeType, Symbol, Volume, cBotLabel, StopLossPips, TakeProfitPips); } } } }
@Spotware
Spotware
14 Jan 2014, 12:55
( Updated at: 18 Mar 2019, 09:05 )
using System; using cAlgo.API; using cAlgo.API.Internals; namespace cAlgo.Indicators { [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC)] public class AccessMarketData : Indicator { public override void Calculate(int index) { // Last (current) high price double high = MarketSeries.High[index]; // Last (current) Close price double close = MarketSeries.Close.LastValue; // previous low price double low = MarketSeries.Low[index - 1]; // open price 2 bars ago double open = MarketSeries.Open[index - 2]; // Last (current) typical price double typical = MarketSeries.Typical.Last(0); // Previous weighted close price var weightedClose = MarketSeries.WeightedClose.Last(1); // Print the median price of the last 5 bars for(int i = 0; i < 5; i++) { double median = MarketSeries.Median.Last(i); Print("Median Price {0} bars ago is {1}", i, median); } // Check if it is Sunday DateTime openTime = MarketSeries.OpenTime.LastValue; if(openTime.DayOfWeek == DayOfWeek.Sunday) { // it is Sunday } // First bar of the day DateTime currentDate = MarketSeries.OpenTime.LastValue.Date; var firstBarIndex = MarketSeries.OpenTime.GetIndexByTime(currentDate); // access the date part var date = openTime.Date; // access the time part var time = openTime.TimeOfDay; // Daylight Saving Time var isDST = openTime.IsDaylightSavingTime(); // convert to UTC var utcTime = openTime.ToUniversalTime(); // total count of tick Volume series int count = MarketSeries.TickVolume.Count; // Last tick Volume double tickVolume = MarketSeries.TickVolume[count - 1]; // symbol code of the current series string symbol = MarketSeries.SymbolCode; // timeframe of the current series TimeFrame timeframe = MarketSeries.TimeFrame; // access to different symbol Symbol symbol2 = MarketData.GetSymbol("AUDUSD"); // access to different series var dailySeries = MarketData.GetSeries(TimeFrame.Daily); } } }
@Spotware
Spotware
13 Jan 2014, 14:39
Using the methods of the new API will require different syntax altogether.
Please see the examples here: /forum/whats-new/1937
Also, you can download the Trading API Guide.
One way of implementing the above would be:
protected override void OnStart() { ExecuteMarketOrderAsync(TradeType.Buy, Symbol, 10000, "My Label", OnExecuted); } private void OnExecuted(TradeResult result) { double TakeProfitPrice; if (result.IsSuccessful && Positions.Count == 1) { var position = result.Position; if (position.TradeType == TradeType.Buy) TakeProfitPrice = position.EntryPrice + TakeProfit * Symbol.TickSize; if (position.TradeType == TradeType.Sell) TakeProfitPrice = position.EntryPrice - TakeProfit * Symbol.TickSize; //... } else { Print("Failed to create position"); } //... }
@Spotware
Spotware
15 Jan 2014, 17:31 ( Updated at: 21 Dec 2023, 09:20 )
RE:
hgorski said:
You can start with the following sample: /forum/calgo-reference-samples/2247
If you like to set it manually, you may click on the security button next to the settings to the left of a position in the positions tab.
@Spotware