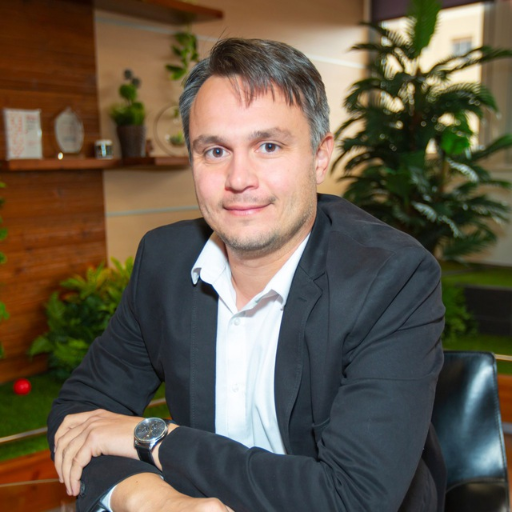
Topics
Replies
PanagiotisCharalampous
02 Oct 2020, 11:59
( Updated at: 21 Dec 2023, 09:22 )
Hi there,
It seems fine to me. You need to provide more information to be able to reproduce the problem.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 10:29
Hi jayteasuk,
That's seconds, around one month.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 09:45
Hi jayteasuk,
If you just want to use the application for your own purposes, you can generate a token using your application's playground and use it in your application.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 09:38
Hi noppanon,
LoadMoreHistory() is not supported in Backtesting at the moment
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 09:19
Hi SuassunaFX,
Can you please explain your issue in English and provide some more information e.g. screenshots?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 09:18
Hi there,
Can you please provide more information about this issue?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 09:03
Hi pietdup59,
cTrader is no different than any other Windows application. You can drag it wherever you want, on any screen you like.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 09:00
Hi firemyst,
cBots/Indicators are typical C# projects so there is nothing specific about. You will use it as you would use it in any C# other project. Commissions are available in Open API.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 08:55
Hi Jay3cast,
This is not available in the mobile applications at the moment.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 08:52
Hi tnormtrade,
You need to contact FxPro regarding this issue.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 08:52
Hi kebbo,
My code shows you how to detect a bat change as soon as it happens. If you want to use it for other timeframes you need to adjust it accordingly. If the code does not enter the if statement, it means the bar has not been created yet and there is nothing you can do about it. Your code is a completely wrong way to solve the problem it and I suggest to forget about it since it does not take into consideration that bars from other symbols might have not been completed yet.
Regarding your questions
But then the previous price is taken (from the candle before, which has to be index 2) and not the one that just closed the candle. Why? Is the bot faster than updating every chart?
The fact that the candle closed in one symbol does not mean that it is closed for the rest, hence you should avoid this approach
how many lines of code can be "processed" per tick? If the market makes a jump of about 5 ticks, will the onTick method be called 5x completely? Even if the market is faster than the bot?
This depends on your pc. If it does not manage to process incoming ticks before the next one arrives, then they will be ignored.
but from the second candle/call it jumps very fast to the needed 10 ticks although there was no price movement at all, why is that?
OnTick() is called whenever a bir or ask price is changed for any of the subscribed symbols. Hence it will not correspond to the movement of the chart.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 08:24
Hi there,
You can try something like this to count the number of consecutive losses.
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class NMewBot : Robot
{
int _consecutiveLosses;
protected override void OnStart()
{
Positions.Closed += Positions_Closed;
}
private void Positions_Closed(PositionClosedEventArgs obj)
{
if (obj.Position.NetProfit < 0)
_consecutiveLosses++;
else
_consecutiveLosses = 0;
}
protected override void OnBar()
{
}
protected override void OnTick()
{
}
}
}
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 08:17
Hi herofus,
This change needs to take place in the indicator, not in the cBot. The code you have written makes no sense at all.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 08:14
Hi Luca,
You can use a flag that will stop more positions from being placed until the conditions are reset again.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
02 Oct 2020, 08:11
Hi firemyst,
You can use Open API to retrieve the symbol information.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
30 Sep 2020, 16:23
Hi Luca,
Please post this in the correct topic.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
30 Sep 2020, 15:59
Hi herofus,
This indicator does not expose this information. The information is stored in the following private variables
private IndicatorDataSeries _highZigZags;
private IndicatorDataSeries _lowZigZags;
You need to make them public and read the information from there.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
30 Sep 2020, 12:33
Hi herofus,
It would be helpful if you could share the zig zag indicator you are using.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
30 Sep 2020, 11:11
Hi xabbu,
You problem is here
IndicatorArea.DrawStaticText("Symbols", _text, VerticalAlignment.Top, HorizontalAlignment.Right, _color);
When the indicator is referenced, the indicator area is not initialized. You can fix it by adding this condition
if (IndicatorArea != null)
IndicatorArea.DrawStaticText("Symbols", _text, VerticalAlignment.Top, HorizontalAlignment.Right, _color);
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
05 Oct 2020, 07:56
Hi gioele.buonadonna,
Here you go
Best Regards,
Panagiotis
Join us on Telegram
@PanagiotisCharalampous