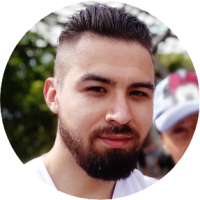
Topics
Replies
whis.gg
22 Nov 2017, 12:59
The calculation method in the refenreced indicator won't be invoked unless you read its output value or force it to calculate.
Add one of these lines into OnTick method inside your cBot.
newIndicator.Calculate(MarketSeries.Open.Count - 1);
var unused = newIndicator.Result.LastValue;
Both samples will make the indicator to calculate at current index.
@whis.gg
whis.gg
20 Nov 2017, 01:16
( Updated at: 15 Jan 2024, 14:51 )
Hi! The server time is always returning current time of the server, you are trying to calculate historical data with it. You should be using MarketSeries.OpenTime instead. See [this indicator ]which is pretty much the same using different approach.
@whis.gg
whis.gg
04 Jul 2017, 15:15
Public: The type or member can be accessed by any other code in the same assembly or another assembly that references it.
Private: The type or member can only be accessed by code in the same class or struct.
Const is a keyword that indicates a constant. It describes an entity that cannot be changed at program runtime.
@whis.gg
whis.gg
29 Jun 2017, 15:15
Hi GammaQuant, sorry for late response I was very busy lately. I have posted the solution on your thread. /forum/cbot-support/11619?page=1#4
@whis.gg
whis.gg
29 Jun 2017, 15:13
Here is a sample of grouping across all instances using static list of custom class called Order. The cBot executes a market order on each new bar and adds to the list. Each time price changes it updates position's State. The cBot also prints number of positions for each State using LINQ queries.
Be aware I haven't tested it so there might be some bugs.
using System.Collections.Generic; using System.Linq; using cAlgo.API; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class GroupsSample : Robot { public enum State { Gaining, BreakingEven, Loosing } public class Order { public Position Position { get; set; } public State State { get; set; } } private static List<Order> _orders; protected override void OnStart() { // creates new list if doesn't exist if (_orders == null) { _orders = new List<Order>(); } // subscribes to Positions.Closed event Positions.Closed += OnPositionClosed; } /// <summary> /// Method called on position closed /// </summary> private static void OnPositionClosed(PositionClosedEventArgs obj) { // finds matching order var matchingOrder = _orders.FirstOrDefault(x => x.Position == obj.Position); // if found if (matchingOrder != null) { // removes order from our list _orders.Remove(matchingOrder); } } /// <summary> /// Method called on each new bar /// </summary> protected override void OnBar() { // executes market order and calls back OnPositionOpened method when filled ExecuteMarketOrderAsync(MarketSeries.Open.Count % 2 == 0 ? TradeType.Buy : TradeType.Sell, Symbol, 1000, OnPositionOpened); // counts how many positions have certain state var gaining = _orders.Count(x => x.State == State.Gaining); var breakingEven = _orders.Count(x => x.State == State.BreakingEven); var loosing = _orders.Count(x => x.State == State.Loosing); // prints number of positions that are gaining, breaking even, loosing Print("Gaining = {0}, BreakingEven = {1}, Loosing = {2}", gaining, breakingEven, loosing); } /// <summary> /// Method called when position opened /// </summary> private void OnPositionOpened(TradeResult result) { // prints error and skips rest of the iteration if result wasn't successful if (!result.IsSuccessful) { Print(result.Error); return; } // adds new order into our list _orders.Add(new Order { Position = result.Position }); } /// <summary> /// Method called on each price change /// </summary> protected override void OnTick() { // loops through all orders in our list and sets their state foreach (var order in _orders) { if (order.Position.NetProfit > 0) { order.State = State.Gaining; } else if (order.Position.NetProfit < 0) { order.State = State.Loosing; } else { order.State = State.BreakingEven; } } } } }
@whis.gg
whis.gg
23 Jun 2017, 11:47
You could write an enum with all three states, then make public class with position and state. Then instead of list of positions you would make a list of your custom class containing positions and state. You could use LINQ queries afterwards.
Do you want to group trades opened by the instance only or across all instances?
@whis.gg
whis.gg
18 Jun 2017, 11:34
private void PrintDistanceForEachPendingOrder() { var pendingOrders = PendingOrders; foreach (var pendingOrder in pendingOrders) { var distancePips = Math.Round(Math.Abs(pendingOrder.TargetPrice - (pendingOrder.TradeType == TradeType.Buy ? Symbol.Ask : Symbol.Bid)) / Symbol.PipSize, 1); Print(distancePips); } }
@whis.gg
whis.gg
16 Jun 2017, 11:13
Difference between current price and target price of pending order, divided by pip size gives you number of pips away from your order.
/api/reference/pendingorder/targetprice
@whis.gg
whis.gg
26 Jan 2018, 00:42
Is it going to be fixed any time soon?
@whis.gg