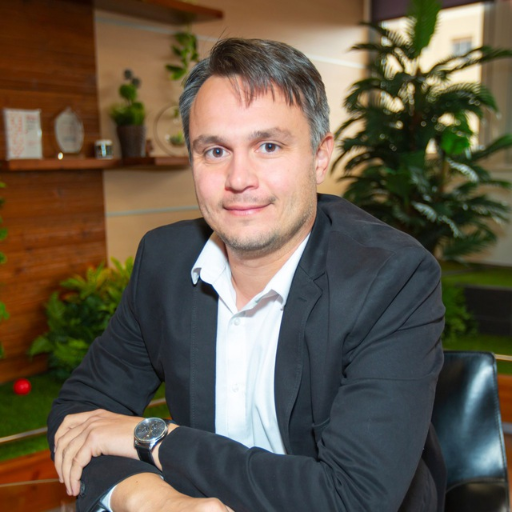
Topics
Replies
PanagiotisCharalampous
12 Mar 2019, 12:07
Hi erikvb,
There are several issues with your cBot. I made some changes so that you can build it but you need to check if this is what you want. See the code below
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class HMABOT : Robot { [Parameter(DefaultValue = 0.0)] public double Parameter { get; set; } [Parameter("Label", DefaultValue = "HMA")] public string Label { get; set; } [Parameter("Volume", DefaultValue = 1000)] public int Volume { get; set; } [Parameter("Source")] public DataSeries Source { get; set; } [Parameter("Periods", DefaultValue = 14)] public int Periods { get; set; } internal HMASignals HmaSignals { get; set; } public bool IsBullish = false; public bool IsBearish = false; private MarketSeries HmaDaySeries; private HMASignals _hmaSignal; protected override void OnStart() { // Put your initialization logic here HmaDaySeries = MarketData.GetSeries(TimeFrame.Daily); _hmaSignal = Indicators.GetIndicator<HMASignals>(Periods,Source,false,false,1,false,1); } protected override void OnTick() { // Put your core logic here // BEARISH if (_hmaSignal.IsBearish && Positions.FindAll(Label, Symbol, TradeType.Buy).Length == 0) { IsBearish = true; IsBullish = false; close(TradeType.Sell); trade(TradeType.Buy); } // BULLISH if (_hmaSignal.IsBullish && Positions.FindAll(Label, Symbol, TradeType.Buy).Length == 0) { IsBearish = false; IsBullish = true; close(TradeType.Buy); trade(TradeType.Sell); } } private void close(TradeType tradeType) { foreach (var position in Positions.FindAll(Label, Symbol, tradeType)) ClosePosition(position); } private void trade(TradeType tradetype) { ExecuteMarketOrder(tradetype, Symbol, Volume, Label); } protected override void OnStop() { // Put your deinitialization logic here } } }
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 11:33
Hi Kafeldom,
There is no such feature at the moment. It will be added in a future update.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 11:32
Hi Ziyad,
If you need professional assistance, you can post a Job or contact a Consultant.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 11:30
Hi James,
As the message clearly states, you need to end your region somewhere. You start your region here
#region Indicator declarations private DSSBressert _DSS { get; set; }
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 11:25
Hi Afrim,
If you need professional assistance, you can post a Job or contact a Consultant.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 11:23
Hi AAZero,
There is no such option at the moment. We will consider this for a future update.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Mar 2019, 17:27
Hi Ben,
There in no equivalent feature on cTrader at the moment.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Mar 2019, 15:32
Dear amsman,
There is no such thing as a single “root” memory issue with cTrader that needs to be addressed. First of all, most of the memory issues are caused by poorly programmed custom cBots and indicators.No matter how efficiently we program cTrader, if the custom cBot/Indicator uses a lof of memory, there is nothing we can do about it. This is why we always ask if a trader is running a custom cBot/Indicator and if yes, we ask for the code to investigate further.
In the rare cases a memory issue is caused by cTrader itself, we ask for the cooperation of traders to address it. I completely disagree with your statement “There is no support offered in these forums to eleviate the problem.... Other than post me you code and we'll have a look...”. We always ask for detailed troubleshooting information to reproduce the problem and we even proceed with TeamViewer sessions to investigate issues on trader machines. As soon as we identify the cause of each issue, we aim to resolve it with the next update. Many members of the community can confirm that they have received such treatment and that their issues have been handled and resolved in a reasonable time.
Also please note that cTrader is an evolving platform that constantly changes as new features and functionalities are introduced.Therefore there is no once and for all solution for everything unless we stop developing the platform. Issues will appear and will be resolved constantly as we release new versions of the platform. This is one of the main reasons we are releasing beta versions before releasing to brokers. So that you can test it and report to us any issues you might have. Therefore if you still face memory issues with cTrader, let us know how to reproduce and we will be happy to have a look.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Mar 2019, 15:29
Hi procumulative@gmail.com,
It seems you are using a custom indicator. Can you please share it with us?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Mar 2019, 11:47
Hi lec0456,
Sorry, my mistake, it does not work for non interactive objects. But we will add this feature in an upcoming update.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Mar 2019, 11:02
Hi Patrick,
Which cTrader are you referring to? I can see targets in Spotware Beta.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Mar 2019, 10:17
Hi lec0456,
ObjectHoverChanged works for non interactive objects as well. See below how to get the index of the hovered line
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class NewcBot : Robot { [Parameter(DefaultValue = 0.0)] public double Parameter { get; set; } protected override void OnStart() { Chart.ObjectHoverChanged += OnChartObjectHoverChanged; var divLine = Chart.DrawVerticalLine("Dayend", Server.Time, Color.Orange, 1, LineStyle.DotsRare); } void OnChartObjectHoverChanged(ChartObjectHoverChangedEventArgs obj) { if (obj.IsObjectHovered) { if (Chart != null) Chart.DrawStaticText("hoverText", obj.ChartObject.Name, VerticalAlignment.Top, HorizontalAlignment.Left, Chart.ColorSettings.ForegroundColor); if (obj.ChartObject is ChartVerticalLine) Print("Index " + (obj.ChartObject as ChartVerticalLine).Time); } else Chart.RemoveObject("hoverText"); } protected override void OnTick() { // Put your core logic here } protected override void OnStop() { // Put your deinitialization logic here } } }
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Mar 2019, 09:53
Hi dmn,
We have investigated this issue and it seems you are using a custom indicator (I-Sessions) which causes the memory issue. Can you try removing the indicator and letting us know if it resolves the issue?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Mar 2019, 09:32
Hi daniel.agg,
Thanks for posting in our forum. I downloaded the cBot but there is no source code. Can you please send it with source code? Also you can just post the code here, no need to send files.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
07 Mar 2019, 17:59
Hi ctid1032297,
Thanks for posting in our forum. Renko and Range bars are not available yet.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
07 Mar 2019, 17:55
Hi Sasha,
Yes it is a bug and we are aware about it. I just wanted to make sure it is the one we know. It happens on optimization when cBots get data form other timeframes than the one they execute on.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
07 Mar 2019, 16:59
Hi Sasha,
I have checked your cBot and you are using data from another timeframe
[Parameter("S & R TimeFrame", DefaultValue = "Daily")] public TimeFrame SRTimframe { get; set; } . . . seriesSR = MarketData.GetSeries(SRTimframe); . . . .
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
07 Mar 2019, 16:20
RE: IsOverlay not work as previous version did.
uvwxyz said:
Hi,
Previously one could use in the Indicators opened chart's tool : f / Custom and overlay a custom indicator B ( built with declaration: "IsOverlay = true;) over another custom indicator A (which is already displayed in a panel), by selecting (in the f / Custom dialouge box) B as the 'Indicator' and selecting an output series of indicator A as the input series (source) for indicator B.
Now doing so draws the Indicator B on the main chart which is useless, and also takes away a lot of power of cTrader custom indicators. Previously it drew on the panel of Indicator A.
Can you please, make this previously available funtionality available again.
Cheers.
Hi abs,
This is a bug and we will fix it.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
07 Mar 2019, 16:01
Hi Ben,
There is no other feature at the moment.You can try double clicking on the Trend Line and choose Show Angle. This feature will help you drawing a straight line.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 12:08
RE: Backtesting Options with custom timeframes
eivaremir said:
Hi eivaremir,
Yes this is in our plans. It will be added in one of the upcoming updates.
Best Regards,
Panagiotis
@PanagiotisCharalampous