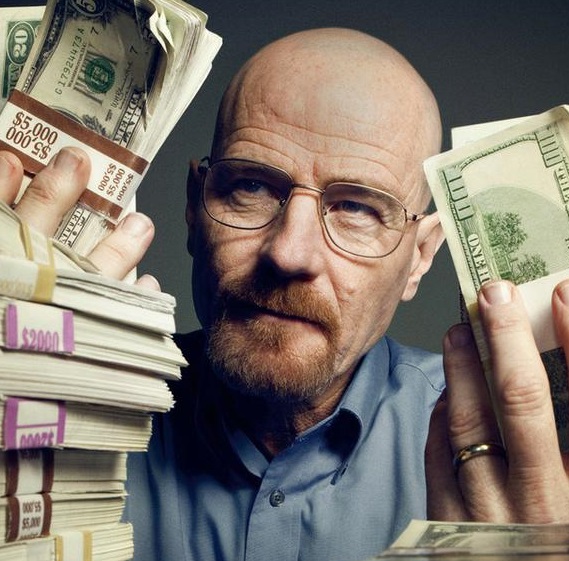
Topics
Replies
moneybiz
03 Oct 2015, 13:32
CPU is the primary player in backtesting.
Also if you can reduce the parameters needed to be optimized will also help a lot or at least increments should not be very small.
The more combinations you have the more calculations needed thus more time. Try fixing some parameters at reasonable values and optimize the remaining.
But how much you can rely on optimization since it's doing a curve fitting on past prices?
@moneybiz
moneybiz
02 Oct 2015, 19:17
protected override void OnBar() { // The new bar's index is 0, the closed bar's index is 1. var prevBarOpenPrice = MarketSeries.Open.Last(1); var prevBarClosePrice = MarketSeries.Close.Last(1); var prevBarHighPrice = MarketSeries.High.Last(1); var prevBarLowPrice = MarketSeries.Low.Last(1); if (prevBarHighPrice - prevBarOpenPrice < prevBarHighPrice - prevBarClosePrice) { // Open Sell. } }
Add the lines above to your bot.
@moneybiz
moneybiz
02 Oct 2015, 14:32
IsRising: Checks if the last value in a dataseries is greater than the previous.
IsFalling: Checks if the last value in a dataseries is less than the previous
If the data series is constructed on Close price then the last value will always fluctuate since the last price (close price) is changing. The prices before that last one will be settled.
In your situation when you're checking for IsRising the tick data (current close price) is rising but just before the second condition is tested for IsFalling a new tick data is received asynchronously and the condition is tested with that fresh data which is lower than the close price of the closed bar (previous bar).
That's why you're receiving two different results inside OnBar event. This should be a very rare case, since it takes few milliseconds or less to calculate the conditions.
You can avoid this by adding ELSE statement in your code to guarantee single execution.
if (MaXX.Result.IsRising()) { // Open BUY. } else if (MaXX.Result.IsFalling()) { // Open SELL. }
@moneybiz
moneybiz
30 Sep 2015, 18:39
RE:
Spotware said:
Dear Trader,
Please use the following code snippet:
[Parameter("RSI Indicator Time Frame", DefaultValue = "Minute15")] public TimeFrame RelativeStrengthIndexIndicatorTimeFrame { get; set; }
Thank you, it works.
It would be nice to be able to set a default TimeFrame for the robot itself addition to the TimeZone and AccessRights parameters.
@moneybiz
moneybiz
21 Sep 2015, 21:29
RE:
cerana said:
using System; using System.IO; using System.Collections.Generic; using System.Linq; using System.Collections; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; using cAlgo.Indicators; using cAlgo.API.Requests; using System.Threading; using System.Timers; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class profit : Robot { [Parameter(DefaultValue = 100.0)] public double Parameter { get; set; } protected override void OnTick() { int a = 0; int b = 0; foreach (var position in Positions) a++; foreach (var position in Positions) if (position.Pips > Parameter) b++; if (a == b) foreach (var position in Positions) { ClosePosition(position); } } } }
Why you don't close every position at once like this?
protected override void OnTick() { foreach (var position in Positions.Where(p=> p.NetProfit > Parameter).ToArray()) { ClosePosition(position); } }
@moneybiz
moneybiz
17 Sep 2015, 11:35
To download something from internet you need to use WebClient.
Add the following using statement to the top, which includes the WebClient class:
using System.Net;
Then inside your OnStart() method you can download the content of the file as follows:
var client = new WebClient(); var content1 = client.DownloadString("http://www.pauna.biz/8052756.txt"); var content2 = client.DownloadString("http://www.pauna.biz/8052756.txt"); if (content1.Contains("8052756")) { // Do something. }
That's it.
@moneybiz
moneybiz
16 Sep 2015, 12:11
RE:
Spotware said:
Dear Trader,
We kindly ask you to perform the following steps:
Uninstall cAlgo
Go ‘My Documents’ delete any cAlgo folders
Enter the below in the SEARCH bar of your PC’s start menu: “%LOCALAPPDATA%”
Open folder “LOCAL”, then “APPS” and delete the “2.0” folder
Uninstall .NET framework from your computer (restart may be required)
Install the latest .NET framework and restart your computer
- Try to install cAlgo again.
Doesn't work.
You can't uninstall .NET 4.6 framework which comes builtin with Windows 10.
The other steps I tried doesn't help.
Faulting module name: clr.dll, version: 4.6.96.0, time stamp: 0x55b96716
From the above line I think you need to compile cAlgo with .NET 4.6 installed or target the 4.6 directly.
@moneybiz
moneybiz
15 Sep 2015, 22:45
RE:
9718853 said:
int totalPips = (int)(Positions[y].Pips - Positions[y].EntryPrice);
and then using totalPips to delay when a martingale strategy is called with this
if ( totalPips <= MartingaleTrigger * Symbol.PipSize)
Positions[y].Pips is already gibing you the profit or loss in pips.
Subtracting Positions[y].EntryPrice from pips is not logical. One is a value like 10 (pips) and the other is a value like 1.12345.
int totalPips = Positions[y].Pips is enough.
What does the MartingaleTrigger represent, pips or price? If pips then totalPips <= MartingaleTrigger is enough.
You use multiplication with Symbol.PipSize only when you want to add/subtract some pips from a price value.
Say you want to set a take profit price position as 10 pips from the entry price.
var takeProfit = Positions[y].EntryPrice + (TakeProfitPips * Symbol.PipSize);
var takeProfit = 1.12345 + (10 * 0.0001) = 1.12445
@moneybiz
moneybiz
15 Sep 2015, 14:04
Reinstalling doesn't help.
Here is more detailed info from event viewer.
--- Application: cAlgo.exe Framework Version: v4.0.30319 Description: The process was terminated due to an internal error in the .NET Runtime at IP 00007FFB1998E16E (00007FFB19980000) with exit code 80131506. --- Faulting application name: cAlgo.exe, version: 1.30.58489.34659, time stamp: 0x55d8d9fd Faulting module name: clr.dll, version: 4.6.96.0, time stamp: 0x55b96716 Exception code: 0xc0000005 Fault offset: 0x000000000000e16e Faulting process id: 0x500 Faulting application start time: 0x01d0efa5a95fc016 Faulting application path: C:\Users\User01\AppData\Local\Apps\2.0\G0PQRP9W.DW0\J1VMY7HW.TKT\calgo_2b1d787f64b7eb41_0001.001e_649829480e6aefdd\cAlgo.exe Faulting module path: C:\Windows\Microsoft.NET\Framework64\v4.0.30319\clr.dll Report Id: 70d923e1-6367-4e73-bf84-8a35fd15db9d Faulting package full name: Faulting package-relative application ID: --- Fault bucket 120318424629, type 4 Event Name: APPCRASH Response: Not available Cab Id: 0 Problem signature: P1: cAlgo.exe P2: 1.30.58489.34659 P3: 55d8d9fd P4: clr.dll P5: 4.6.96.0 P6: 55b96716 P7: c0000005 P8: 000000000000e16e P9: P10: Attached files: C:\Users\User01\AppData\Local\Temp\WER943.tmp.WERInternalMetadata.xml C:\Users\User01\AppData\Local\Temp\WER11BF.tmp.appcompat.txt These files may be available here: C:\ProgramData\Microsoft\Windows\WER\ReportArchive\AppCrash_cAlgo.exe_2ab9e13edb4f7c2e2faf4e765855bc2b4798d37_b126fcd0_1e831316 Analysis symbol: Rechecking for solution: 0 Report Id: 70d923e1-6367-4e73-bf84-8a35fd15db9d Report Status: 1 Hashed bucket: 8f0a548ae781a48ac72d08502c83e275 ---
@moneybiz
moneybiz
14 Sep 2015, 12:49
( Updated at: 19 Mar 2025, 08:57 )
RE:
Spotware said:
Dear Trader,
Could you please send us your brokers name and the environment you use (live or demo) at support@ctrader.com
It's backtesting should not be depended on live or demo account.
Anyway, I sent an email to the above address.
@moneybiz
moneybiz
13 Sep 2015, 20:03
RE:
1906442735 said:
I would like to know, why i can not set a stop loss like 0.5, 0.23 or 0,9 at time to create an ExecuteOrder.
ExecuteMarketOrder/Async converts entered pips to integer values. That's why you can't set real numbers like 0.53, 0.23, 0.9.
Set them according to the code above, that's how I do it for the same reason as yours.
@moneybiz
moneybiz
13 Sep 2015, 19:58
RE:
1906442735 said:
I would like to know, why i can not set a stop loss like 0.5, 0.23 or 0,9 at time to create an ExecuteOrder.
In my case, I am just opening positions like this:
ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, Label, 0.53, 0);
Because I really need to set a stoploss with that kind of size.
Sometimes cAlgo create the position and with that stoploss and sometimes it does not.
I do not know why, but I would like to know if cAlgo have an specific small limit for stoploss.
Thank you for your answer as soon as posible.
Place the order without stop loss/take profit then when the order result is returned add the stop loss/take profit pips to the entry price of the returned order and use ModifyPosition/Async to update to the exact positions you want.
I do it this way:
public void EnsurePositionRangesSet(Position position, double? stopLossPips, double? takeProfitPips, double pipSize) { var update = false; double? stopLossPrice = null; double? takeProfitPrice = null; if (stopLossPips.HasValue && !position.StopLoss.HasValue) { stopLossPrice = position.TradeType == TradeType.Buy ? position.EntryPrice - stopLossPips.Value*pipSize : position.EntryPrice + stopLossPips.Value*pipSize; update = true; } if (takeProfitPips.HasValue && !position.TakeProfit.HasValue) { takeProfitPrice = position.TradeType == TradeType.Buy ? position.EntryPrice + takeProfitPips.Value*pipSize : position.EntryPrice - takeProfitPips.Value*pipSize; update = true; } if (!update) { return; } if (!IsBacktesting) { LogEvent(TraceEventType.Verbose, 0, "Updating position ranges. EP: {0}, SL: {1}, TP: {2}", position.EntryPrice, stopLossPrice, takeProfitPrice); } UpdatePositionSafeAsync(position, stopLossPrice, takeProfitPrice, null, UpdatePositionRetryErrorCodes); }
@moneybiz
moneybiz
13 Sep 2015, 19:50
( Updated at: 23 Jan 2024, 13:16 )
RE:
tradermatrix said:
Hello
I already asked this question..et the answer here:[/forum/calgo-support/6054]
cordially
It has been about 2 months and there is no solution yet.
If you look at the chart above it is not a tick data problem. It's internal bug in backtest engine.
If it was a tick data problem the bar would look different since I believe the bar is constructed from tick data itself.
There is probably some approximation error which calculates the tick data to be at different price.
@moneybiz
moneybiz
11 Sep 2015, 01:23
RE:
When doing backtests I noticed that on 28 Jun 2015 the EUR/USD the market opened with a huge gap of ~165 pips which was draining my equity.
Then I decided to close all the open positions on Friday 30 mins before market closes. But how?
I noticed that the Robot class has Time property which gives you the price's date and time as the ticks are coming. But these values are controlled by the TimeZone setting which is specified as an attribute property.
At GMT+3 the market closes at 00:00, so when I set TimeZone = TimeZones.GTBStandardTime I was able to compare whether Robot.Time is greater than 23:30 to be able to close the open positions.
This is when the TimeZone comes to play. It gives you the price date and time according to the setting specified.
@moneybiz
moneybiz
01 Sep 2015, 19:16
RE:
Paul_Hayes said:
take a look at the logic in the Trend Lines Indicator and draw these characters ate the start and end points:-
▼▲
Thank you. It's interesting to do things that way but works.
@moneybiz
moneybiz
01 Sep 2015, 03:20
RE:
Spotware said:
Dear Trader,
Please have a look at the Position events section of our API Programmers Guides and at OnBar vs OnTick section of our cAlgo support site.
Thank you bu I don't ask for the usage of the events.
I'm asking whether they are fired async or sync. It seems to me that the position data comes together packed with tick data and first OnTick() event is fired then Position.Closed event (if there is one in the received packet from the server).
If they are independent of each other and there is no ordering guaranteed I will implement a locking mechanism, that's why I'm asking this question.
I want to know whether they are independent of each other or you're putting them in any order to prevent users to dive into async coding?
@moneybiz
moneybiz
03 Oct 2015, 19:50
>> SslStream is not guaranteed to be thread safe.
This means if you want to access the object from different threads it's up to you to implement the concurrency handling mechanisms.
Just lock some common object and then Read/Write. When that object is locked only one thread can work on it. The others will wait till the lock is released.
@moneybiz