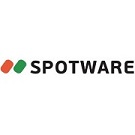
Topics
Forum Topics not found
Replies
cAlgo_Fanatic
15 May 2013, 11:25
Open your solution in Visual Studio and add a reference to the cAlgo.API.dll. To locate the folder containing it, right click and open file location of cAlgo.exe from Task Manager processes.
Save the text file (cs) to the robots/indicators location and build from cAlgo.
The path to the robots/indicators can be located from the drop down box of the algorithms listing in cAlgo
@cAlgo_Fanatic
cAlgo_Fanatic
15 May 2013, 10:26
Gaps are not uncommon. When the price moves sharply up or down, with little or no trading in between a gap is produced.
@cAlgo_Fanatic
cAlgo_Fanatic
15 May 2013, 10:26
Also see examples at the API Refence fo PlaySound and SendEmail.
@cAlgo_Fanatic
cAlgo_Fanatic
14 May 2013, 17:39
Daily High and Low Indicator. Displays horizontal lines that correspond to the daily high and low.
Source Code:
using System; using cAlgo.API; namespace cAlgo.Indicators { [Indicator(IsOverlay = true)] public class DailyHighLow : Indicator { public override void Calculate(int index) { DateTime today = MarketSeries.OpenTime[index].Date; DateTime tomorrow = today.AddDays(1); double high = MarketSeries.High.LastValue; double low = MarketSeries.Low.LastValue; for (int i = MarketSeries.Close.Count - 1; i > 0; i--) { if (MarketSeries.OpenTime[i].Date < today) break; high = Math.Max(high, MarketSeries.High[i]); low = Math.Min(low, MarketSeries.Low[i]); } ChartObjects.DrawLine("high " + today, today, high, tomorrow, high, Colors.Pink); ChartObjects.DrawLine("low " + today, today, low, tomorrow, low, Colors.Pink); } } }
@cAlgo_Fanatic
cAlgo_Fanatic
14 May 2013, 17:07
Indicator showing Round Numbers based on input step pips calculated between the chart High and Low.
Source Code:
using System; using cAlgo.API; namespace cAlgo.Indicators { [Indicator(IsOverlay = true)] public class RoundNumbers : Indicator { [Parameter(DefaultValue = 100)] public int StepPips { get; set; } protected override void Initialize() { double max = MarketSeries.High.Maximum(MarketSeries.High.Count); double min = MarketSeries.Low.Minimum(MarketSeries.Low.Count); double step = Symbol.PipSize*StepPips; double start = Math.Floor(min/step)*step; for (double level = start; level <= max + step; level += step) { ChartObjects.DrawHorizontalLine("line_" + level, level, Colors.Gray); } } public override void Calculate(int index) { } } }
@cAlgo_Fanatic
cAlgo_Fanatic
14 May 2013, 14:22
cAlgo will reconnect automatically if the connection is lost.
@cAlgo_Fanatic
cAlgo_Fanatic
14 May 2013, 12:10
Thank you for the suggestion. We will add it to our list of future implementations.
@cAlgo_Fanatic
cAlgo_Fanatic
14 May 2013, 12:10
Currently this is not possible but the ability for robots to receive input from the chart is in our list of future implementations.
@cAlgo_Fanatic
cAlgo_Fanatic
14 May 2013, 11:50
Alerts are comming soon in cTrader. You may do this programmatically with cAlgo if you wish. If you need help coding this let us know and we will provide instructions.
@cAlgo_Fanatic
cAlgo_Fanatic
14 May 2013, 11:49
Please remove the backtesting cache and see if the issue is resolved. To do this follow the instructions below:
Close cAlgo/cTrader application
Open the folder: \AppData\Roaming:
Press win key + r
Type %appdata%
Locate the application cache folder:
Open the folder cTrader or cAlgo
Open the folder "Cache"
Delete ALL the files in the Cache folder.
Restart the application.
@cAlgo_Fanatic
cAlgo_Fanatic
14 May 2013, 11:19
You can close the position once the price crosses below the ema20. Below is an example that creates one position on robot start and if the ema20 crosses above the close the position is closed.
private ExponentialMovingAverage ema20; private Position position; // Source is the price (O-L-H-C) used in the ExponentialMovingAverage calculation [Parameter] public DataSeries Source { get; set; } // Period of the ExponentialMovingAverage [Parameter(DefaultValue = 20, MinValue = 1)] public int Period { get; set; } protected override void OnStart() { // Initialize ExponentialMovingAverage Indicator ema20 = Indicators.ExponentialMovingAverage(Source, Period); Trade.CreateMarketOrder(TradeType.Buy, Symbol, 100000); } protected override void OnTick() { // EMA is above price if (ema20.Result.HasCrossedAbove(MarketSeries.Close, 0)) { Trade.Close(position); } } protected override void OnPositionOpened(Position openedPosition) { position = openedPosition; }
@cAlgo_Fanatic
cAlgo_Fanatic
13 May 2013, 10:06
You may create a custom indicator that references the two indicators and plots their results. e.g.
[Indicator(IsOverlay = false, ScalePrecision = 5)] public class twoIndicators : Indicator { StandardDeviation standDev; HistoricalVolatility histVolatility; [Output("StandardDeviation")] public IndicatorDataSeries Result { get; set; } [Output("HistoricalVolatility")] public IndicatorDataSeries Result2 { get; set; } protected override void Initialize() { // Initialize and create nested indicators standDev = Indicators.StandardDeviation(MarketSeries.Close, 14, MovingAverageType.Simple); histVolatility = Indicators.HistoricalVolatility(MarketSeries.Close, 20, 262); } public override void Calculate(int index) { // Calculate value at specified index Result[index] = standDev.Result[index]; Result2[index] = histVolatility.Result[index]; }
@cAlgo_Fanatic
cAlgo_Fanatic
10 May 2013, 15:01
In order to risk 5% of the balance, you should calculate the stop loss to equal 5% of the balance.
@cAlgo_Fanatic
cAlgo_Fanatic
10 May 2013, 12:46
We will upload a robot for you based on your description in the Robots section.
Before we do this we need you to clarify your definition of Risk: "The cBot risk 1% of account balance to go long/short the equivelent amount of currency (NO stop loss orders are used in this "system"). "
You cannot have a risk 1% of account balance without stop loss or stop out.
@cAlgo_Fanatic
cAlgo_Fanatic
10 May 2013, 09:53
Only favourites and email alert settings are currently saved in the cloud. Your settings will be saved only locally on your browser. Could you please confirm that you logged back in using the same browser on the same machine and could you let us know which browser you used? In the future all your settings will be saved in the cloud as well.
@cAlgo_Fanatic
cAlgo_Fanatic
09 May 2013, 17:04
Yes, given that the currency of your account is in USD. You can calculate the profit of all positions of the account as well as close all positions of the account:
var netProfit = 0.0; foreach (var openedPosition in Account.Positions) { netProfit += openedPosition.NetProfit; } if(netProfit >= 30.0) { foreach (var openedPosition in Account.Positions) { Trade.Close(openedPosition); } }
@cAlgo_Fanatic
cAlgo_Fanatic
09 May 2013, 16:50
You can have a robot that calculates the pips of all positions closed that were opened by the same robot. To do this add the positions in a List (see List example) in the OnPositionClosed event:
private readonly List<Position> _closedPositions = new List<Position>(); //... protected override void OnPositionClosed(Position closedPosition) { _closedPositions.Add(closedPosition); } //... protected override void OnTick() { double totalPipsClosed = 0.0; foreach(Position pos in _closedPositions) { totalPipsClosed += pos.Pips; } //... }
Calculating the pips of all closed positions of the account is not currently possible. Access to historical orders will be added soon to the API.
@cAlgo_Fanatic
cAlgo_Fanatic
15 May 2013, 16:34
The limit order does not become a market order.
The limit order stands in the order book and it is filled at the asked price or better.
You can place a limit order inside the spread and it will be filled at the price you specify or better.
So, it is "a real standing order" that stands in the order book on the server matching engine.
@cAlgo_Fanatic