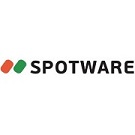
Topics
Forum Topics not found
Replies
cAlgo_Fanatic
22 May 2013, 15:08
The Position.NetProfit/Position.GrossProfit is available.
@cAlgo_Fanatic
cAlgo_Fanatic
22 May 2013, 15:05
No. (-deltaY, deltaX) are window coordinates. If you set the ray and then modify the chart scale alone the angle value will change.
@cAlgo_Fanatic
cAlgo_Fanatic
21 May 2013, 17:40
This code will loop through the Account positions when the robot is stoped and each position whose profit is less than 200 pips will be closed. If this is not your algorithm logic please send some more information or example.
protected override void OnStop() { foreach (var position in Account.Positions) { var pipValue = Symbol.PipSize/Symbol.Ask*position.Volume; if (position.NetProfit < -200*pipValue) { Trade.Close(position); } } }
@cAlgo_Fanatic
cAlgo_Fanatic
21 May 2013, 17:31
var angle = (int) (Math.Atan2(-deltaY, deltaX) * 180 / Math.PI);
It's calculated in pixels in current chart scale. If you want to do it in cAlgo API, it's impossible because we do not operate with pixels there.
@cAlgo_Fanatic
cAlgo_Fanatic
21 May 2013, 17:07
( Updated at: 11 Mar 2016, 14:16 )
If you change the code of the referenced indicator you have to rebuild the robot before backtesting again.
@cAlgo_Fanatic
cAlgo_Fanatic
20 May 2013, 14:08
( Updated at: 23 Jan 2024, 13:16 )
Please see this sample: [High and Low of Current Week]
@cAlgo_Fanatic
cAlgo_Fanatic
20 May 2013, 14:07
using System; using cAlgo.API; namespace cAlgo.Indicators { [Indicator(IsOverlay = true)] public class HighLowCurrentWeek : Indicator { public override void Calculate(int index) { double high = MarketSeries.High.LastValue; double low = MarketSeries.Low.LastValue; for (int i = MarketSeries.Close.Count - 1; i > 0; i--) { if (MarketSeries.OpenTime[i].DayOfWeek < DayOfWeek.Monday) break; high = Math.Max(high, MarketSeries.High[i]); low = Math.Min(low, MarketSeries.Low[i]); } ChartObjects.DrawHorizontalLine("high", high, Colors.Pink); ChartObjects.DrawHorizontalLine("low", low, Colors.Pink); } } }
@cAlgo_Fanatic
cAlgo_Fanatic
20 May 2013, 12:18
You can start by looking at this example that creates multiple positions: Multiple Positions.
OnPositionOpened method is triggered when a position is opened and OnPositionClosed when a position is closed by the robot.
@cAlgo_Fanatic
cAlgo_Fanatic
20 May 2013, 12:01
We are investigating this. This bug is related to our change that improves text rendering performance.
@cAlgo_Fanatic
cAlgo_Fanatic
20 May 2013, 11:41
The .algo file is not protected. If you run it on your pc, nobody can access it but if you send it to someone else it is possible that they can view the code. In the future we will implement encryption for .algo files.
@cAlgo_Fanatic
cAlgo_Fanatic
20 May 2013, 10:34
You can replace ID by Label. Since for the time being you cannot set the label for manual trades this will work with existing positions created by a robot where you can specify the label.
[Parameter("Position Label")] public string PositionLabel { get; set; } //... private Position Position { get { return Account.Positions.FirstOrDefault(position => position.Label == PositionLabel); } } //... private void CheckPosition() { if (Position == null) { Print("Invalid position Label {0} or position closed ", PositionLabel); Stop(); } } //...
Documentation for C# syntax and examples may be found on the msdn website.
@cAlgo_Fanatic
cAlgo_Fanatic
20 May 2013, 10:11
The code cannot be seen by anyone. It is only on your local pc. Nobody has access to it.
@cAlgo_Fanatic
cAlgo_Fanatic
20 May 2013, 10:00
This is so that the calculation occurs only on loading of the indicator, in the Initialize() method and thereon only when there are new bars, in the Calculate() method.
@cAlgo_Fanatic
cAlgo_Fanatic
20 May 2013, 09:17
It is by definition of risking a percent of your balance. If you only risk a certain percentage of your balance then you need to set stop loss. Otherwise you are risking 100%.
@cAlgo_Fanatic
cAlgo_Fanatic
17 May 2013, 17:51
Currently there is no version history. We may post it here on cTDN in the future.
@cAlgo_Fanatic
cAlgo_Fanatic
17 May 2013, 17:41
Hello,
The cBot has been uploaded here: /algos/robots/show/271
@cAlgo_Fanatic
cAlgo_Fanatic
16 May 2013, 16:52
The equity is calculated according to what would have been the balance if all trades were closed at that instance. If a position is long the profit will be calculated according to the bid and if a position is short according to the offer. Equity is unrealized profit and loss.
@cAlgo_Fanatic
cAlgo_Fanatic
16 May 2013, 11:26
( Updated at: 23 Jan 2024, 13:16 )
Hello,
To get started use the cAlgo platfrom editor. When you create a new Indicator/Robot it automatically inserts the necessary methods for you.
Here are some usefull links.
Get Started:
http://help.spotware.com/calgo/cbots/create-edit
http://help.spotware.com/calgo/indicators/create-edit
executing trades/orders:
/api/requests also
position management
/api/position
/api/robot/onpositionopened-caab
DOM (MarketDepth) provides access to Level 2 prices. In cTrader you can view the DOM for each currency pair on the left. MarketDepth provides access to that data.
/forum/calgo-reference-samples/253
It is possible to create your own helper classes/libraries that can be used by multiple custom indicators/robots by adding a reference to their dll. (Add reference button located next to build on top of cAlgo editor)
The code is provided for some of the cAlgo Indicators in this section:
/forum/calgo-reference-samples
e.g. [MACD], [Momentum Oscillator], RSI
If you need to code for another let us know.
BackTesting provides one year of data for the time being. It will be increased in the future. The actual spread of the market at the historical point in time is not available for backtesting right now.
We don't provide a list of bugs for the time being. There are currently no known bugs as far as backtesting is concerned.
@cAlgo_Fanatic
cAlgo_Fanatic
15 May 2013, 18:01
Thank you for your suggestions. Some of them are already in our to do list.
@cAlgo_Fanatic
cAlgo_Fanatic
22 May 2013, 15:35
You can modify the code in Sample Trailing Robot and check each position's volume and modify the stop loss accordingly.
Use variables such as trigger and trailingStop which will be set to the corresponding input parameter - Trigger1/Trigger2 and TrailingStop1/TrailingStop2 according to the Volume of the position.
The code below is a modification of the Sample Trailing Stop Robot in the cAlgo platform:
Where Volume1, Volume2, Trigger1, Trigger2,TrailingStop1, and TrailingStop2 are input parameters.
@cAlgo_Fanatic