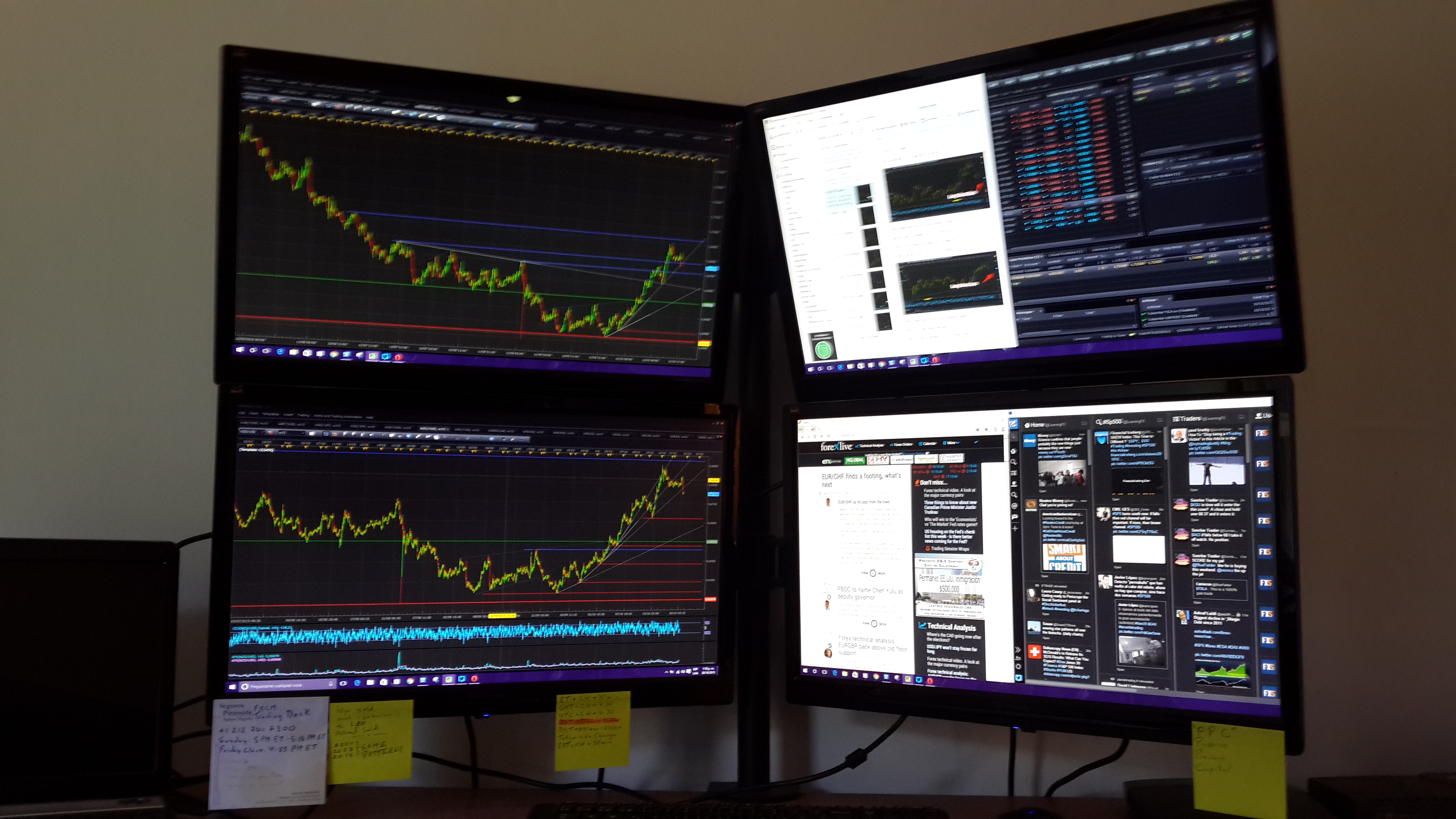
Topics
Replies
AlgoCorner
23 Dec 2022, 22:23
RE:
This isn't possible, you can use indicator data from a bot but you cannot use attributes like Output inside a bot.
@AlgoCorner
AlgoCorner
23 Dec 2022, 22:15
RE: RE:
What he means is that in
var kyushu_d_bullish = kyushu_d.kyushu_bullish;
Since kyushu_d is a Bars object, it doesn't have any "kyushu_bullish" property
kyushu_d has properties like kyushu_d.OpenPrices.Last(n) and etc you can use instead
@AlgoCorner
AlgoCorner
18 Dec 2022, 01:16
( Updated at: 21 Dec 2023, 09:23 )
RE: Still not working for me With VS2022 17.3.4 and Ctrader Automate version 1.0.4 (which is the latest version)
So, in case someone is still experiencing this issue, I was able to sort it out by changing the kind of debug symbols, inside the project properties, changed it to PDB file, current platform:
The System.Private.Core issue is still present in some of my solutions though
Regards,
@AlgoCorner
AlgoCorner
04 Nov 2022, 08:42
RE: RE: RE:
That's different, not sure but something like:
var checkPrice = 1.5;
var crossedBar = Bars.Select((bar, index) => (bar, index)).LastOrDefault(x => x.bar.Low <= checkPrice && x.bar.High >= checkPrice);
if (crossedBar.index != 0)
Print($"Bar Index is {crossedBar.index}");
@AlgoCorner
AlgoCorner
01 Nov 2022, 21:04
RE:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using cAlgo.API;
using cAlgo.API.Collections;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
namespace cAlgo
{
[Indicator(AccessRights = AccessRights.None)]
public class NewIndicator : Indicator
{
private ChartVerticalLine _verticalLine;
/*
-- I was wondering if it were possible for me to get the Bars info (eg. OpenPrices, ClosePrices, etc.) from a ChartObject's (such as a vertical line) Time index?
-- Any help is appreciated, thanks.
*/
protected override void Initialize()
{
_verticalLine = Chart.DrawVerticalLine("VerticalLine", Bars.OpenTimes.LastValue, Color.Red);
var index = Bars.OpenTimes.GetIndexByTime(_verticalLine.Time);
var bar = Bars[index];
Print("Open: {0}, High: {1}, Low: {2}, Close: {3}", bar.Open, bar.High, bar.Low, bar.Close);
}
public override void Calculate(int index)
{
// Calculate value at specified index
// Result[index] =
}
}
}
@AlgoCorner
AlgoCorner
01 Nov 2022, 20:31
Hello Spotware,
Thanks for your answer, and I may take the opportunity to ask to also give us the option soon to choose whether to open by default in visual studio or these other 2 IDEs, VsCode or Rider.
Thanks for your support,
Regards,
@AlgoCorner
AlgoCorner
31 Oct 2022, 19:47
RE:
Hello Panagiotis,
I'm using Spotware 4.4.17, this doesn't work either way with string or enum. but it should work with using enums as before, there shouldn't be a downgrade to this feature, it's just a small bug I believe, that should be fixed asap.
Regards,
@AlgoCorner
AlgoCorner
18 Oct 2022, 04:05
This is quite an issue on 1.0.3, please fix as soon as possible.
Regards,
@AlgoCorner
AlgoCorner
13 Oct 2022, 13:05
RE: RE:
I'm having some reference issues with WebView and Window
- Severity Code Description Project File Line Suppression State
Error CS0246 The type or namespace name 'Window' could not be found (are you missing a using directive or an assembly reference?) WindowSample C:\Users\USUARIO\Documents\cAlgo\Sources\Indicators\WindowSample\WindowSample\WindowSample.cs 23 Active
- Severity Code Description Project File Line Suppression State
Error CS0103 The name 'WindowStartupLocation' does not exist in the current context WindowSample C:\Users\USUARIO\Documents\cAlgo\Sources\Indicators\WindowSample\WindowSample\WindowSample.cs 73 Active
- Severity Code Description Project File Line Suppression State
Error CS0246 The type or namespace name 'WebView' could not be found (are you missing a using directive or an assembly reference?) WebViewOnWindow C:\Users\USUARIO\Documents\cAlgo\Sources\Indicators\WebViewOnWindow\WebViewOnWindow\WebViewOnWindow.cs 8 Active
- Severity Code Description Project File Line Suppression State
Error CS0246 The type or namespace name 'WebViewLoadedEventArgs' could not be found (are you missing a using directive or an assembly reference?) WebViewOnWindow C:\Users\USUARIO\Documents\cAlgo\Sources\Indicators\WebViewOnWindow\WebViewOnWindow\WebViewOnWindow.cs 41 Active
I'm using latest version of Spotware cTrader, these references should not be missing.
Also, when I build with "Embedded" compiler, these errors are not shown, but it doesn't let me add any instance.
@AlgoCorner
AlgoCorner
13 Oct 2022, 10:45
RE: RE: Answer about Sharpe and Sortino Ratio calculation
Your example works great, thank you!
@AlgoCorner
AlgoCorner
12 Oct 2022, 08:14
Assign a label to the positions, it should be an unique label per instance, so there's no risk of conflict.
Example
private string _label;
protected override void OnStart()
{
_label = "BotName" + Server.Time.Ticks;
}
//Then everytime you trade use this label, i.e. and check for positions with that label
protected override void OnBar()
{
if (Positions.Count(x => x.Label == _label) < 5)
ExecuteMarketOrder(TradeType.Buy, SymbolName, 10000, _label, InputStopLoss, InputTakeProfit);
}
@AlgoCorner
AlgoCorner
11 Oct 2022, 08:20
RE: RE: RE: RE: RE:
The problem is that this message is always showing up since last updates, I would like to have it fixed:
System.Private.CoreLib.pdb not included
@AlgoCorner
AlgoCorner
10 Oct 2022, 22:57
RE: RE: RE:
Hello Spotware,
Here are my steps to reproduce this issue, I find little info about this on internet, and never happened to me before, would appreciate your help.
10.10.2022-14.51.58 (screencast.com)
Regards,
@AlgoCorner
AlgoCorner
08 Oct 2022, 11:37
RE: RE: RE: RE: RE: RE: Figured out!
Do check if my settings may help you somehow please. But I'd also like Spotware to help about the annoying System.Private.CoreLib.dll issue
@AlgoCorner
AlgoCorner
08 Oct 2022, 09:36
( Updated at: 08 Oct 2022, 09:37 )
RE: RE: RE: RE: Figured out!
Please see video attached, so I have these problems but I'm still able to debug afterwards.
@AlgoCorner
AlgoCorner
07 Oct 2022, 21:08
RE: RE: Figured out!
Even if these messages show up you should be able to keep debugging after closing them, that's what I did.
The problem is that these errors show up and it's annoying, haven't been able to fix it.
@AlgoCorner
AlgoCorner
06 Oct 2022, 00:54
RE:
Hello Spotware, great update as always.
Would you please show examples of the following features?
- Use WebView control
- Send email Notifications via MailKit
- Display Custom Windows
- Display Active Chart in Minimized View
Regards,
@AlgoCorner
AlgoCorner
01 Jun 2021, 06:23
( Updated at: 21 Dec 2023, 09:22 )
RE:
PanagiotisCharalampous said:
Hi Waxy,
Can you please provide us with the following?
- Application scale
- Windows scale
- Display resolution
Also please send us troubleshooting information. Paste the link to this discussion in the text box.
Best Regards,
Panagiotis
It seems it only happens when using "Change the size of text, apps and other items" to 150%
@AlgoCorner
AlgoCorner
10 Jan 2023, 21:34 ( Updated at: 21 Dec 2023, 09:23 )
RE:
Spotware said:
Hello Spotware,
You can see I have Load all modules checked, but still, it doesn't work, would appreciate your support:
Regards,
@AlgoCorner