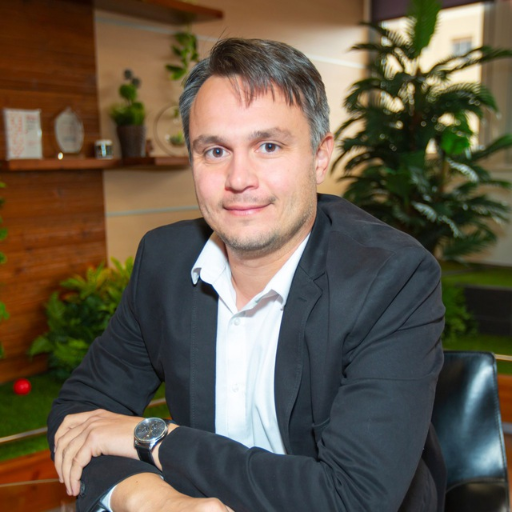
Topics
Replies
PanagiotisCharalampous
18 Jan 2018, 10:05
Hi Surady,
See below a very simple example on how to calculate your average equity with samples taken on each bar change.
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class AverageEquity : Robot { [Parameter(DefaultValue = 0.0)] public double Parameter { get; set; } private double _equity; private double _periodCount; protected override void OnStart() { ExecuteMarketOrder(TradeType.Buy, Symbol, 10000); } protected override void OnBar() { _equity += Account.Equity; _periodCount++; Print("Average Equity:" + (_equity / _periodCount)); } protected override void OnStop() { // Put your deinitialization logic here } } }
If you need something more complicated or any professional help in developing your cBot, you can post a Job or contact a professional Consultant.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
18 Jan 2018, 09:48
Hi irmscher,
Volume should work fine for indices. Can you tell us what is the problem you experience so that we can help?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
17 Jan 2018, 17:20
Hi tmc.
API functions are not thread safe therefore they should be called in the main thread of a cBot. Therefore parallelization of this functionality is currently not possible.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
17 Jan 2018, 17:07
Hi Drummond360,
Indeed it is a typo.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
17 Jan 2018, 11:21
Hi Patrick,
Thanks for notifying us for this. It seems to be a bug. I will notify the product team to have a look at this.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
16 Jan 2018, 14:49
Hi dordkash@gmail.com,
This feature is currently only available in the backtesting chart. If you want you can post a suggestion in the Suggestions section for consideration by the product.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
16 Jan 2018, 12:19
Hi anghenly,
No, as soon as the order is sent then there isn't a way to stop it. If you are concerned about accidentally clicking this button, you might consider changing your QuickTrade settings and enable the Double-Click mode.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
16 Jan 2018, 11:52
Hi mindbreaker,
The problem here is that your Take Profit pips are a value higher than 50000 which is the maximum limit that cTrader accepts currently. We will remove this limitation in a future release.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
16 Jan 2018, 11:34
Hi hadikhalil86@gmail.com,
Did you check your spam folder in case these emails end up there?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
16 Jan 2018, 11:30
Hi dordkash@gmail.com,
Is the cAlgo screenshot from the actual symbol chart or from the backtesting chart?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
15 Jan 2018, 16:30
Dear Patrick,
Thanks for taking the time to bring up this subject. This idea is already in the backlog for some time now and I have communicated with the product team to push the priority a bit more since it seems there is demand for this feature. Hopefully we will see this in one of the future releases of cTrader.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
15 Jan 2018, 10:58
Hi dordkash@gmail.com,
Thanks for reporting this issue. Can you please tell us if you took any specific action for this to happen and if you can consistently reproduce it? When it happens again then I advise you to send some troubleshooting information to our Quality Assurance team. You can achieve that by pressing Ctrl+Alt+Shift+T and then pressing the "Submit" button on the form that will pop up. In the message area please paste a link of this discussion as well so that the QA team can associate the report with the issue.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
15 Jan 2018, 10:40
Hi irmscher9,
The answer to your question is no the backtesting data start date is not moving forward with time. The availabilty of backtesting tick and trend bar data is related to the amount of availability historical data provided by your broker.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jan 2018, 17:16
Hi oneplusoc,
This happens because of the scale of the chart. If you want to combine the two indicators, you will have this problem. Momentum oscillator usually oscillates around 100.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jan 2018, 16:44
Dear oneplusoc,
See a corrected version below
using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; using cAlgo.Indicators; namespace cAlgo { [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class NewIndicator : Indicator { [Parameter(DefaultValue = 0.0)] public double Parameter { get; set; } [Output("RSI", Color = Colors.Blue)] public IndicatorDataSeries RSI { get; set; } [Output("Momentum", Color = Colors.Green)] public IndicatorDataSeries Momentum { get; set; } private RelativeStrengthIndex _rsi; private MomentumOscillator _momentum; protected override void Initialize() { _rsi = Indicators.RelativeStrengthIndex(MarketSeries.Close, 14); _momentum = Indicators.MomentumOscillator(MarketSeries.Close, 14); } public override void Calculate(int index) { RSI[index] = _rsi.Result[index]; Momentum[index] = _momentum.Result[index]; } } }
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jan 2018, 11:59
Hi oneplusoc,
The solution is to create a custom indicator that combines the two indicators together. See a relevant discussion here.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jan 2018, 11:50
Hi Drummond360,
It is better to put the information in the forum so that other community members can contribute as well and the possible solutions are kept for future reference.
However I understand that you might not want to expose your work to everybody. If this is the case then fill free to contact me at community at spotware dot com.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jan 2018, 11:42
Hi oneplusoc,
See below
using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; namespace cAlgo.Indicators { [Levels(30, 70, 80, 50, 20)] [Indicator(IsOverlay = false, TimeZone = TimeZones.UTC)] public class aarsiforall : Indicator { private RelativeStrengthIndex ma1; private MarketSeries series1; private Symbol symbol1; [Parameter(DefaultValue = "AUDUSD")] public string Symbol1 { get; set; } [Parameter(DefaultValue = 14)] public int Period { get; set; } [Output("MA Symbol 1", Color = Colors.Red, PlotType = PlotType.Line, Thickness = 1)] public IndicatorDataSeries Result1 { get; set; } protected override void Initialize() { symbol1 = MarketData.GetSymbol(Symbol1); series1 = MarketData.GetSeries(symbol1, TimeFrame); ma1 = Indicators.RelativeStrengthIndex(series1.Close, Period); } public override void Calculate(int index) { ShowOutput(symbol1, Result1, ma1, series1, index); } private void ShowOutput(Symbol symbol, IndicatorDataSeries result, RelativeStrengthIndex RelativeStrengthIndex, MarketSeries series, int index) { int index2 = GetIndexByDate(series, MarketSeries.OpenTime[index]); result[index] = RelativeStrengthIndex.Result[index2]; string text = string.Format("{0} {1}", symbol.Code, Math.Round(result[index], 0)); ChartObjects.DrawText(symbol.Code, text, index + 1, result[index], VerticalAlignment.Center, HorizontalAlignment.Right, Colors.Yellow); } private int GetIndexByDate(MarketSeries series, DateTime time) { for (int i = series.Close.Count - 1; i >= 0; i--) if (time == series.OpenTime[i]) return i; return -1; } } }
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jan 2018, 10:57
Hi Drummond360,
The provided information is not enough for us to reach to a conclusion. We need to understand why you expected that the trade should lock in a profit. In order to be able to investigate this, we will all the necessary information to be able to run the backtesting ourselves. So we need
- The full cBot code.
- The cBot's initial parameters.
- All the backtesting parameters.
- The trade that you believe should be profitable.
If you send us the above, we can have a look and advise further.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
18 Jan 2018, 16:20
Dear mosaddequ@gmail.com,
Thanks for posting in our forum. You can try something like the following
Best Regards,
Panagiotis
@PanagiotisCharalampous