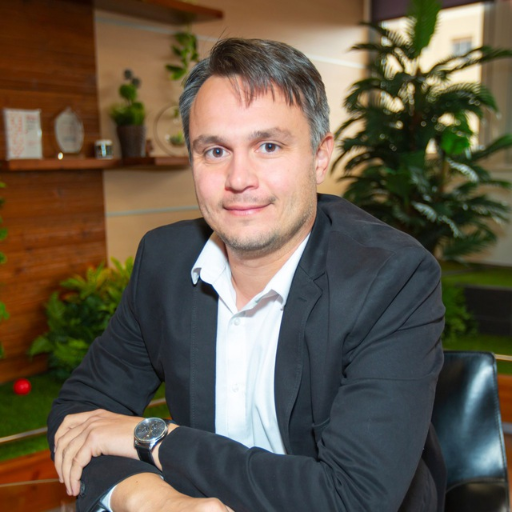
Topics
Replies
PanagiotisCharalampous
12 Feb 2018, 11:22
Hi thegreat.super,
You can find an explanation for this kind of exceptions here. The problem probably occurs because you are checking lastposition without checking if it is null first. See below
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class ashi : Robot { [Parameter(DefaultValue = 0.0)] public double Parameter { get; set; } [Parameter("ProfitTarget", DefaultValue = 300)] public double ProfitTarget { get; set; } [Parameter("Volume", DefaultValue = 10000)] public int Volume { get; set; } public double balance; public double lastTradePrice; public TradeResult lastposition; protected override void OnStart() { // Get the initial balance balance = Account.Balance; Print(balance); // Start hedge grid lastposition = ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, "buy", 0, 2); ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, "buy", 0, 2); } protected override void OnTick() { // Open more trades if the price moved 5 pips if (lastposition != null && lastposition.Position.Pips > 5) { lastposition = ExecuteMarketOrder(TradeType.Buy, Symbol, Volume, "buy", 0, 2); } if (lastposition != null && lastposition.Position.Pips < -5) { lastposition = ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, "sell", 0, 2); } // Check if equity is in profit then shut down the grid cycle if (Account.Equity >= balance + 2) { foreach (var position in Positions) { ClosePosition(position); } balance = Account.Balance; Print(balance); // Start hedge grid ExecuteMarketOrder(TradeType.Buy, Symbol, 1000, "buy", 0, 2); ExecuteMarketOrder(TradeType.Sell, Symbol, 1000, "sell", 0, 2); } } protected override void OnStop() { // Put your deinitialization logic here } } }
Let me know if the above resolves the problem.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Feb 2018, 11:12
Hi dordkash@gmail.com,
Let me know if this works for you
if (Symbol.Bid - MarketSeries.High.LastValue > Symbol.PipSize) { // Do something }
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Feb 2018, 10:51
Hi tradingu,
Currently our servers are located in LD5. Therefore you should preferably look for a VPS provider located in LD5.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
09 Feb 2018, 17:04
Hi tmc.
It is going to take some time to fix this issue due to some architectural changes that need to take place. The issue has to do with the fact that TP and SL messages are sent slightly after the position is opened resulting to the arguments not having this information. However if you can wait for a little you can TP and SL are updated and you can read the information. See my code sample below
using System; using cAlgo.API; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleOnPositionOpenedBug : Robot { private readonly Action[] _actions = new Action[6]; private int _index; protected override void OnStart() { Positions.Opened += Positions_Opened; _actions[0] = () => PlaceStopOrderAsync(TradeType.Buy, Symbol, 1000, Symbol.Ask, "label", 5, 5, null, "Stop Order Async"); _actions[1] = () => PlaceLimitOrderAsync(TradeType.Buy, Symbol, 1000, Symbol.Bid, "label", 5, 5, null, "Limit Order Async"); _actions[2] = () => ExecuteMarketOrderAsync(TradeType.Buy, Symbol, 1000, "label", 5, 5, null, "Market Order Async"); _actions[3] = () => PlaceStopOrder(TradeType.Buy, Symbol, 1000, Symbol.Ask, "label", 5, 5, null, "Stop Order"); _actions[4] = () => PlaceLimitOrder(TradeType.Buy, Symbol, 1000, Symbol.Bid, "label", 5, 5, null, "Limit Order"); _actions[5] = () => ExecuteMarketOrder(TradeType.Buy, Symbol, 1000, "label", 5, 5, null, "Market Order"); Timer.Start(2); } protected override void OnTick() { foreach (var position in Positions) { Print("{0} (TP: {1} SL: {2})", position.Comment, position.TakeProfit, position.StopLoss); } } protected override void OnTimer() { if (_index >= _actions.Length) { Stop(); } _actions[_index](); _index++; } private void Positions_Opened(PositionOpenedEventArgs e) { if (e.Position.Label == "label") { Print("{0} filled (TP: {1} SL: {2})", e.Position.Comment, e.Position.TakeProfit, e.Position.StopLoss); } } } }
Could we work on a workaround based on the above?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
09 Feb 2018, 14:34
Hi itmfar,
See below
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; using System.Collections.Generic; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class NewcBot : Robot { [Parameter(DefaultValue = 0.0)] public double Parameter { get; set; } [Parameter("MA Type", DefaultValue = MovingAverageType.Simple)] public MovingAverageType maType { get; set; } private StochasticOscillator _stochastic; private readonly List<bool> _ssdRising = new List<bool>(); [Parameter("Source")] public DataSeries Source { get; set; } protected override void OnStart() { _stochastic = Indicators.StochasticOscillator(9, 3, 9, maType); } protected override void OnBar() { _ssdRising.Add(_stochastic.PercentD.IsRising()); if (_ssdRising.Count > 1) { FindSSCrossovers(Source.Count - 1); DrawLinesSSD(Source.Count - 1); } } private void FindSSCrossovers(int index) { if (_stochastic.PercentD.HasCrossedAbove(_stochastic.PercentK, 0)) { ChartObjects.DrawText("MAXss1" + index, "S⮟", index, Source[index], VerticalAlignment.Top, HorizontalAlignment.Center, Colors.Yellow); } else { ChartObjects.RemoveObject("MAXss1" + index); } if (_stochastic.PercentK.HasCrossedAbove(_stochastic.PercentD, 0)) { ChartObjects.DrawText("Minss1" + index, "S⮝", index, Source[index], VerticalAlignment.Bottom, HorizontalAlignment.Center, Colors.Yellow); } else { ChartObjects.RemoveObject("Minss1" + index); } } private void DrawLinesSSD(int index) { if (_ssdRising[_ssdRising.Count - 2] != _ssdRising[_ssdRising.Count - 1] && _stochastic.PercentD[index] > 60 && _ssdRising[_ssdRising.Count - 1] == false) { ChartObjects.DrawText("maxrssd" + index, "D⮟", index, Source[index], VerticalAlignment.Top, HorizontalAlignment.Center, Colors.White); ExecuteMarketOrder(TradeType.Sell, Symbol, 1000, "lalbelmax", 120, 200); } else { ChartObjects.RemoveObject("maxrssd" + index); } if (_ssdRising[_ssdRising.Count - 2] != _ssdRising[_ssdRising.Count - 1] && _stochastic.PercentD[index] < 40 && _ssdRising[_ssdRising.Count - 1] == true) { ChartObjects.DrawText("minrssd" + index, "D⮝", index, Source[index], VerticalAlignment.Bottom, HorizontalAlignment.Center, Colors.White); ExecuteMarketOrder(TradeType.Buy, Symbol, 1000, "lalbelmax", 120, 200); } else { ChartObjects.RemoveObject("minrssd" + index); } } protected override void OnStop() { // Put your deinitialization logic here } } }
Let me know if this is working for you,
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
09 Feb 2018, 11:41
Hi megna,
See below an example of an Order Mass Status Request
8=FIX.4.4|9=117|35=AF|34=3|49=theBroker.12345|52=20170404-07:20:55.325|56=CSERVER|57=TRADE|225=20170404-07:20:44.582|584=mZzEY|585=7|10=065|
Let me know if this is what you are looking for
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
09 Feb 2018, 09:55
Hi jetza,
Currently there is no such feature in cTrader. You can post it as a Suggestion so that it can be considered by the product team.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Feb 2018, 16:58
Hi thegreat.super,
I understand that. But the code you posted, closes all of them. Did you try to run it on cTrader and it did not close all positions? Did you just backtest it? I am just trying to understand how you reached to the conclusion that it is not working.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Feb 2018, 16:45
Hi itmfar,
I don't know what to suggest since I cannot reproduce the problem and understand what is going wrong. Could you share a short video demonstrating the problem? Also, I don't understand how you will solve the problem by calling Calculate on a more frequent basis. Calculate is called on every tick therefore frequently enough. Do you mean redrawing the entire indicator instead? Even if this is what you are looking for, it is not a proper solution. It would be easier to understand how the issue is caused and apply a proper solution instead of forcing a brute force redrawing of the indicator.
Best Regards
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Feb 2018, 12:39
Hi gallderhen,
Thanks for posting in our forum. Unfortunately it is not possible to change the Symbol of an Indicator. It is always the Symbol of the chart to which the indicator is attached to.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Feb 2018, 11:20
Hi thegreat.super,
From what i see
foreach (var position in Positions) { ClosePosition(position); }
closes all positions.
Why do you say that it closes only profitable ones?
Best Regards
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
08 Feb 2018, 11:09
Hi patrick.sifneos@gmail.com,
The issue has been fixed in our internal builds, the fix will be there with the next release. We have a very rigirous QA process and releases are composed of several new features and bug fixes, most of them interrelated. We will release as soon as we make sure that everything is working properly. In other words, we do not fix bugs one by one on the live server :).
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
07 Feb 2018, 17:19
Hi tmc,
Your proposal is a nice workaround and could work for most of the cases. However, since SL is a market order, the closing price could be both above and below the price. Therefore, the solution would not be 100% accurate (e.g. in case of positive slippage).
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
07 Feb 2018, 15:16
Hi Alexander,
Currently there is no way to know how a position was closed. You could post a suggestion in the Suggestions section.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
07 Feb 2018, 15:11
Hi trend_meanreversion,
Your email has been received. We will investigate and come back to you.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
07 Feb 2018, 14:25
( Updated at: 19 Mar 2025, 08:57 )
Hi trend_meanreversion,
Please send me your broker and the relevant Order ID or Deal ID at support@ctrader.com.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
07 Feb 2018, 11:24
Hi itmfar,
Thanks for posting the indicator. I used your indicator on a minute chart for some time but did not experience any problem. It would be helpful to be able to reproduce the problem so that I can see it and see how I can help you.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
06 Feb 2018, 14:19
Hi kuba.pod@gmail.com,
Thanks for posting in our forum. Currently there is no way to set a starting time for a cBot. Therefore, I think that your solution is the way to solve this issue. You can set the starting time as a parameter of the cBot and then make the relevant checks in the code.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
06 Feb 2018, 14:15
Hi trend_meanreversion,
TP is a is just a limit order linked to your position. So when the price reaches TP price, cServer sends a limit order to the LP. However I cannot provide an explanation of what happened since we do not control the entire execution chain. The broker does. Let them investigate and give you a response. If there is an issue with cTrader, they will contact us directly.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Feb 2018, 11:35
Hi irmscher9,
Why do you say that the code is not working? Do you get an exception? Do you get unexpected results? If you give us more information, it will be easier for us to help you.
Best Regards,
Panagiotis
@PanagiotisCharalampous