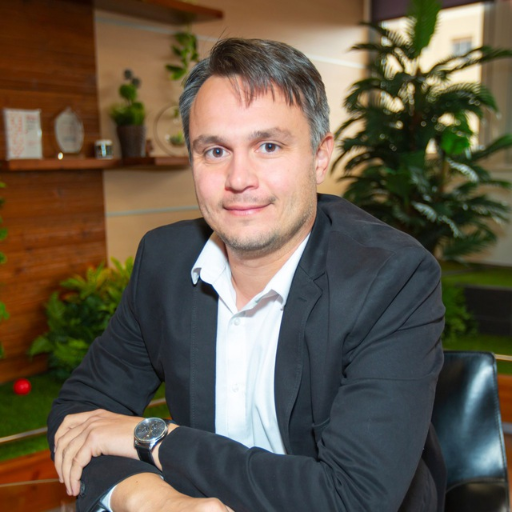
Topics
Replies
PanagiotisCharalampous
12 Mar 2019, 15:47
Hi thamimsibi3,
Thanks for the clarification, that was not very clear.
See below the cBot based on your clarification
// ------------------------------------------------------------------------------------------------- // // This code is a cAlgo API sample. // // This cBot is intended to be used as a sample and does not guarantee any particular outcome or // profit of any kind. Use it at your own risk // // The "Sample Martingale cBot" creates a random Sell or Buy order. If the Stop loss is hit, a new // order of the same type (Buy / Sell) is created with double the Initial Volume amount. The cBot will // continue to double the volume amount for all orders created until one of them hits the take Profit. // After a Take Profit is hit, a new random Buy or Sell order is created with the Initial Volume amount. // // ------------------------------------------------------------------------------------------------- using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleMartingalecBot : Robot { [Parameter("Initial Quantity (Lots)", DefaultValue = 0.01, MinValue = 0.01, Step = 0.01)] public double InitialQuantity { get; set; } [Parameter("Stop Loss", DefaultValue = 3)] public int StopLoss { get; set; } [Parameter("Take Profit", DefaultValue = 3)] public int TakeProfit { get; set; } private Random random = new Random(); protected override void OnStart() { Positions.Closed += OnPositionsClosed; ExecuteOrder(InitialQuantity, GetRandomTradeType()); } private void ExecuteOrder(double quantity, TradeType tradeType) { var volumeInUnits = Symbol.QuantityToVolumeInUnits(quantity); var result = ExecuteMarketOrder(tradeType, Symbol, volumeInUnits, "Martingale", StopLoss, TakeProfit); if (result.Error == ErrorCode.NoMoney) Stop(); } private void OnPositionsClosed(PositionClosedEventArgs args) { Print("Closed"); var position = args.Position; if (position.Label != "Martingale" || position.SymbolCode != Symbol.Code) return; if (position.GrossProfit > 0) { ExecuteOrder(InitialQuantity, position.TradeType); } else { if (position.TradeType == TradeType.Sell) ExecuteOrder(position.Quantity * 2, TradeType.Buy); else ExecuteOrder(position.Quantity * 2, TradeType.Sell); } } private TradeType GetRandomTradeType() { return random.Next(2) == 0 ? TradeType.Buy : TradeType.Sell; } } }
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 15:18
Hi ryan.a.blake,
As the name indicates Spotware Beta is a beta version of cTrader. So usually it is one or two versions ahead. After properly tested and stabilized it is released to brokers.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 15:02
Hi astevani,
This is an old thread. Do you still experience the issue of indicators displaying differently on normal chart and on a backtesting chart? If yes, can you share one to check?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 14:48
Hi a.fernandez.martinez,
Here is an example
var series = MarketData.GetSeries(TimeFrame.Minute); Print(series.TickVolume.LastValue);
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 14:45
Hi thamimsibi3,
Thanks for posting in our forum. See below
// ------------------------------------------------------------------------------------------------- // // This code is a cAlgo API sample. // // This cBot is intended to be used as a sample and does not guarantee any particular outcome or // profit of any kind. Use it at your own risk // // The "Sample Martingale cBot" creates a random Sell or Buy order. If the Stop loss is hit, a new // order of the same type (Buy / Sell) is created with double the Initial Volume amount. The cBot will // continue to double the volume amount for all orders created until one of them hits the take Profit. // After a Take Profit is hit, a new random Buy or Sell order is created with the Initial Volume amount. // // ------------------------------------------------------------------------------------------------- using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class SampleMartingalecBot : Robot { [Parameter("Initial Quantity (Lots)", DefaultValue = 0.01, MinValue = 0.01, Step = 0.01)] public double InitialQuantity { get; set; } [Parameter("Stop Loss", DefaultValue = 3)] public int StopLoss { get; set; } [Parameter("Take Profit", DefaultValue = 3)] public int TakeProfit { get; set; } private Random random = new Random(); protected override void OnStart() { Positions.Closed += OnPositionsClosed; ExecuteOrder(InitialQuantity, GetRandomTradeType()); } private void ExecuteOrder(double quantity, TradeType tradeType) { var volumeInUnits = Symbol.QuantityToVolumeInUnits(quantity); var result = ExecuteMarketOrder(tradeType, Symbol, volumeInUnits, "Martingale", StopLoss, TakeProfit); if (result.Error == ErrorCode.NoMoney) Stop(); } private void OnPositionsClosed(PositionClosedEventArgs args) { Print("Closed"); var position = args.Position; if (position.Label != "Martingale" || position.SymbolCode != Symbol.Code) return; if (position.GrossProfit > 0) { ExecuteOrder(InitialQuantity, position.TradeType); } else { ExecuteOrder(position.Quantity * 2, position.TradeType); } } private TradeType GetRandomTradeType() { return random.Next(2) == 0 ? TradeType.Buy : TradeType.Sell; } } }
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 14:43
Hi pozhy,
I offered a solution to a similar problem here.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 14:35
Hi procumulative@gmail.com,
We have received your email, thanks.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 14:30
Hi Nasser,
The information is not enough for somebody to help you. Please provide us with the following
1) The complete cBot code.
2) The parameters you use to run the code.
3) The parameters you use for the displayed indicators.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 14:08
Hi erikvb,
It seems we have different indicators and it crashes on parameter initialization.You need to change the initialization of the indicator to the following
_hmaSignal = Indicators.GetIndicator<HMASignals>(Periods,false,false,1,false,1);
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 13:02
Hi pozhy,
In principle it is unless it is blocked. Paul Hayes has written a nice article about this.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 12:58
Hi everythingbusiness10,
If you need professional assistance for developing your cBot, you can always post a Job or contact a Consultant.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 12:57
( Updated at: 19 Mar 2025, 08:57 )
Hi Adrian,
Is it possible to send us the cBot and your settings file at support@ctrader.com so that we can have a look?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 12:52
Hi hmozahem,
Thanks for posting in our forum. Can you please let us know if you can still reproduce this behavior? If yes, can you tell us the broker please?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 12:49
Hi guys,
I tried to reproduce the problem but could not. If you can isolate the issue into a smaller indicator with more specific reproduction steps I can take another look.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 12:30
Hi dmn,
We will check this.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 12:26
Hi Jeziel,
This is because the indicator recalculates its values when zoom is changed from the code. When changed from the interface, you need to handle this change yourself, as my solution did.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 12:21
Hi astevani,
This is a known issue. Please read more here.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 12:14
Hi a.fernandez.martinez,
No there is no such feature. A cBot operates on a single chart.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 12:11
( Updated at: 21 Dec 2023, 09:21 )
RE:
gakazas said:
Hello, Thanks for the update.
"Load Parameters" for a cbot doesn't work when dealing with custom enums, as even if the parameter is saved in the .cbotset file, when loading from it an error message appears and the first option is always selected.
Hi gakazas,
Thans for letting us know about this. We will investigate it.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Mar 2019, 15:49
Hi Xavier,
What you are trying to do is not supported. The proper way to read output attributes is the below
Best Regards,
Panagiotis
@PanagiotisCharalampous