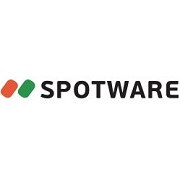
Topics
Replies
admin
24 Jan 2013, 12:22
// ------------------------------------------------------------------------------- // // This is a Sample used as a guideline to build your own Robot. // Please use the forum to provide us with your suggestions about cAlgo’s API. // // ------------------------------------------------------------------------------- using cAlgo.API; namespace cAlgo.Robots { [Robot] public class ModifyAccountPositions : Robot { protected override void OnTick() { foreach (var position in Positions) { if (position.StopLoss == null) { Print("Modifying {0}", position.Id); ModifyPosition(position, GetAbsoluteStopLoss(position, 10), GetAbsoluteTakeProfit(position, 10)); } } } private double GetAbsoluteStopLoss(Position position, int stopLossInPips) { return position.TradeType == TradeType.Buy ? position.EntryPrice - Symbol.PipSize * stopLossInPips : position.EntryPrice + Symbol.PipSize * stopLossInPips; } private double GetAbsoluteTakeProfit(Position position, int takeProfitInPips) { return position.TradeType == TradeType.Buy ? position.EntryPrice + Symbol.PipSize * takeProfitInPips : position.EntryPrice - Symbol.PipSize * takeProfitInPips; } } }
@admin
admin
24 Jan 2013, 11:00
The reason inheritance of robot classes is no longer supported is for better performance. The only change that can be made is that inheritance may be supported for as long as no metadata is in the base class. In other words, attributes (IndicatorAttribute, RobotAttribute, ParameterAttribute, OutputAttribute, etc) are not included in the base class. Let us know if this is something usefull to you and we will request an implementation for it.
@admin
admin
24 Jan 2013, 10:59
Have you tried backtesting it? If you are using OnBar that means that the code will execute on each new trendbar which will depend on the timeframe that you are using. If you are using the default hourly timeframe you would have to wait up to an hour before the code executes. Try a small timeframe like a minute, as well as backtesting.
@admin
admin
23 Jan 2013, 17:55
Yes, it is correct. A simple print statement will prove that this condition is met within the code. It is probably something else that is not correct.
You can test this code and let us know:
[Robot] public class NewRobot : Robot { Position _position; protected override void OnTick() { if(Trade.IsExecuting) return; double firstclose = MarketSeries.Close[MarketSeries.Close.Count-1]; double firstopen = MarketSeries.Open[MarketSeries.Close.Count-1]; if (firstopen > firstclose) { //Sell if(_position == null) Trade.CreateSellMarketOrder(Symbol, 1000); } } protected override void OnPositionOpened(Position openedPosition) { _position = openedPosition; } }
@admin
admin
23 Jan 2013, 16:54
For the time being, the backtesting feature supports a year of historical data. More data is likely to be included in the future but it is difficult to say up to which date, though. Could you let us know why it is important to you to have such large range of data for backtesting? Thank you in advance.
@admin
admin
23 Jan 2013, 16:42
Yes, you are correct. This functionality has been removed since the latest update. If the purpose of doing this is to hide the code, you may easily do so by distributing the compiled file (.algo file) and a text file (.cs file) that has the same name but does not contain any code. Probably, you should include some comments in the text file, like do not build or modify this file, etc. If the reason is different may we ask you to let us know about it, so that we can decide on an appropriate course of action. Thank you in advance.
@admin
admin
23 Jan 2013, 14:56
Hello,
Those files may be removed but they do not affect performance. Try some code optimizations. See for instance: http://www.dotnetperls.com/optimization
@admin
admin
23 Jan 2013, 11:07
It looks like the problem may be in the rest of the code.
If you have two positions or more open simultaneously then you need to use a list to store multiple positions. See list sample. Using one global field position will work only with one position open at a time.
Alternatively if your code has only two positions opened at a time, you may use two global fields one for sell and one for buy. Then you will need to check the TradeType in the OnPositionOpened and OnPositionClosed methods to assign the correct position object (from the parameter) to the corresponding global position field.
protected override void OnPositionOpened(Position openedPosition) { if (openedPosition.TradeType == TradeType.Buy) _buyPosition = openedPosition; else _sellPosition = openedPosition; } protected override void OnPositionClosed(Position closedPosition) { if (closedPosition.TradeType == TradeType.Buy) _buyPosition = null; else _sellPosition = null; }
You would also have to adjust the rest of the code to replace the one field position using the same logic.
Let us know if you require additional help.
@admin
admin
21 Jan 2013, 14:51
Thank you for bringing this to our attention. We will add documentation or sample code for the above.
When you say protection do you mean in case you distribute your algorithms? If that is the case you may only share the .algo file along with a dummy text file which will have the same name and the .cs extension (the cs file is for the purpose of having the algorithm populated in the indicator/robot list of cAlgo).
@admin
admin
21 Jan 2013, 12:51
Some tips about Windows 8:
-Increase page file:
Go to Advanced System Settings menu
Alter the OS virtual memory settings and visual options
Switch to Desktop mode
Click the Libraries shortcut in the taskbar
Right-click on Computer
In the resulting context menu select Properties.The System control panel will open
Click on Advanced System Settings in the left pane of the window. The System Properties control panel will open.
Click on the Advanced tab
click on the Settings button in the Performance section at the top to open the Performance Options control panel.
Click on the Advanced tab at the top and on the resulting menu
click on the Change button in the Virtual Memory section
Click on Custom Size and since you have 8GB or ram make sure that the initial size and Maximum size is over 8156MBs.
To evaluate issue:
Use Event Viewer and check what else could be going wrong during the time that cAlgo crashed.
To access event viewer in Windows 8:
Press Windows key + R
Type in eventvwr.msc
At windows logs select Application section
Evaluate the logs there at the time cAlgo crashes you will see there a log of cAlgo crashing. You may send us this log in order to investigate further.
You may also check the System section, you might find some usefull information as to what is causing this issue.
@admin
admin
21 Jan 2013, 12:16
Those are the only error codes.
You would have to move this statement orderPlaced = true to the OnPendingOrderCreated.
Due to asynchronous operation the pending order would probably not have been placed right after the statements to create the limit orders.
@admin
admin
21 Jan 2013, 12:03
Hello Scott,
You need to declare Period1, Period2, etc. Like you had in your original code as input parameter for example.
Below is the declaration of Period1. It is of type integer (int) and it is taged as an input parameter.
[Parameter("L1", DefaultValue = 8)] public int Period1 { get; set; }
Useful links : c# Tutorials
Your complete code should be:
using System; using cAlgo.API; using cAlgo.API.Indicators; namespace cAlgo.Indicators { [Indicator(IsOverlay = true)] public class sma8 : Indicator { [Parameter] public DataSeries Source { get; set; } [Parameter("L1", DefaultValue = 8)] public int Periods { get; set; } [Parameter("L2", DefaultValue = 11)] public int Periods2 { get; set; } [Parameter("L3", DefaultValue = 16)] public int Periods3 { get; set; } [Parameter("L4", DefaultValue = 27)] public int Periods4 { get; set; } [Parameter("L5", DefaultValue = 43)] public int Periods5 { get; set; } [Parameter("L6", DefaultValue = 75)] public int Periods6 { get; set; } [Parameter("L7", DefaultValue = 100)] public int Periods7 { get; set; } [Parameter("L8", DefaultValue = 144)] public int Periods8 { get; set; } [Output("Level1", Color = Colors.Green, Thickness = 5)] public IndicatorDataSeries Result { get; set; } [Output("Level2", Color = Colors.Yellow, Thickness = 5)] public IndicatorDataSeries Result2 { get; set; } [Output("Level3", Color = Colors.White, Thickness = 5)] public IndicatorDataSeries Result3 { get; set; } [Output("Level4", Color = Colors.Aqua, Thickness = 5)] public IndicatorDataSeries Result4 { get; set; } [Output("Level5", Color = Colors.Gray, Thickness = 5)] public IndicatorDataSeries Result5 { get; set; } [Output("Level6", Color = Colors.Orange, Thickness = 5)] public IndicatorDataSeries Result6 { get; set; } [Output("Level7", Color = Colors.Red, Thickness = 5)] public IndicatorDataSeries Result7 { get; set; } [Output("Level8", Color = Colors.Purple, Thickness = 5)] public IndicatorDataSeries Result8 { get; set; } private SimpleMovingAverage _simpleMovingAverage1; private SimpleMovingAverage _simpleMovingAverage2; private SimpleMovingAverage _simpleMovingAverage3; private SimpleMovingAverage _simpleMovingAverage4; private SimpleMovingAverage _simpleMovingAverage5; private SimpleMovingAverage _simpleMovingAverage6; private SimpleMovingAverage _simpleMovingAverage7; private SimpleMovingAverage _simpleMovingAverage8; protected override void Initialize() { _simpleMovingAverage1 = Indicators.SimpleMovingAverage(Source, Periods); _simpleMovingAverage2 = Indicators.SimpleMovingAverage(Source, Periods2); _simpleMovingAverage3 = Indicators.SimpleMovingAverage(Source, Periods3); _simpleMovingAverage4 = Indicators.SimpleMovingAverage(Source, Periods4); _simpleMovingAverage5 = Indicators.SimpleMovingAverage(Source, Periods5); _simpleMovingAverage6 = Indicators.SimpleMovingAverage(Source, Periods6); _simpleMovingAverage7 = Indicators.SimpleMovingAverage(Source, Periods7); _simpleMovingAverage8 = Indicators.SimpleMovingAverage(Source, Periods8); } public override void Calculate(int index) { Result[index] = _simpleMovingAverage1.Result[index]; Result2[index] = _simpleMovingAverage2.Result[index]; Result3[index] = _simpleMovingAverage3.Result[index]; Result4[index] = _simpleMovingAverage4.Result[index]; Result5[index] = _simpleMovingAverage5.Result[index]; Result6[index] = _simpleMovingAverage6.Result[index]; Result7[index] = _simpleMovingAverage7.Result[index]; Result8[index] = _simpleMovingAverage8.Result[index]; } } }
@admin
admin
24 Jan 2013, 12:35
Please use the code in this sample and let us know if it does not function properly. If it does then it is probably something else in the code that is not functioning as intended.
The code in your previous post does not modify any orders. You need this statement in order to modify orders Trade.ModifyPosition. See ModifyPosition in the API reference.
@admin