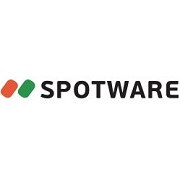
Topics
Replies
admin
24 Sep 2012, 10:50
Hello TETL,
DateTime.Now is the current date and time on your machine
Server.Time is the current date and time on the server.
You can access various members of the Datetime like Date, Time, Hour, etc. as well as functions like AddHours(), AddMinutes(), etc.
In a Robot you need to initialize your time variable in the OnStart event and check the condition in either the OnTick or the OnBar event, depending on your algorithm.
Look at the code snippet below as an example of how you can use DateTime and Server.Time.
private DateTime stopTime; protected override void OnStart() { stopTime = DateTime.Now.Date.AddHours(22); } protected override void OnTick() { // if (Server.Time < stopTime) { // Open new positions }
@admin
admin
20 Sep 2012, 15:19
Then you need to modify your if statement to the following:
if (cci.Result.LastValue > 1 && (_position == null || _position.TradeType == TradeType.Sell) )
{
OpenPosition(TradeType.Buy);
}
else if (cci.Result.LastValue < -1 && (_position == null || _position.TradeType == TradeType.Buy))
{
OpenPosition(TradeType.Sell);
}
@admin
admin
19 Sep 2012, 15:31
Hello SpotTrader,
Yes you can but make sure that the code of one Robot does not affect the other. For instance one Robot may be closing all positions that are open for the account.
You may use a List to keep track of the positions that each robot is creating and closing:
// Declaration private readonly List _positions = new List(); // OnPositionOpened protected override void OnPositionOpened(Position openedPosition) { _positions.Add(openedPosition); } // OnPositionClosed protected override void OnPositionClosed(Position closedPosition) { if (_positions.Contains(closedPosition)) { _positions.Remove(closedPosition); } }
// access within OnTick/OnBar foreach (Position position in _positions ) { // do something e.g. if(position.NetProfit < ProfitLevel) Trade.Close(position); }
@admin
admin
19 Sep 2012, 15:22
Hello PsykotropyK,
If you are still unable to reference an indicator from a robot please post your indicator and your robot code here so that we can troubleshoot it.
@admin
admin
19 Sep 2012, 14:55
Hello Qingyang2005,
Historical orders will be accessible in the robots in the near future.
@admin
admin
19 Sep 2012, 14:34
( Updated at: 23 Jan 2024, 13:14 )
Hello josker,
The Indicator's default location is: My Documents > cAlgo > Sources > Indicators
and the Robot's is: My Documents > cAlgo > Sources > Robots
You can find more information in the algorithms page: [/algos]
There is a small link under Algorithms "How to Install" with instructions.
@admin
admin
19 Sep 2012, 14:13
( Updated at: 23 Jan 2024, 13:11 )
Hello misado,
Your code needs some modification in order to work properly.
First you need to make sure the position is not null before accessing it.
So, your code could be modified to this:
if (cci.Result.LastValue < 0 && (_position == null || _position.TradeType == TradeType.Sell) )
{
OpenPosition(TradeType.Buy);
}
if (cci.Result.LastValue > 0 && (_position == null || _position.TradeType == TradeType.Buy))
{
OpenPosition(TradeType.Sell);
}
Please visit this link to understand how to code using Position.
[/docs/reference/calgo/api/position]
Also, when you use Trade.ModifyPosition to modify the stop loss and take profit, you need to provide the full take profit or stop loss price not the pips.
In other words this: Trade.ModifyPosition(openedPosition,GetAbsoluteStopLoss(openedPosition,StopLoss),1);
means this: Take Profit Hit will occur when the currency quote will equal 1.
Therefore, if we want Take Profit to be equal to the entry price plus 1 pip, we need
takeprofit = _position.EntryPrice + Symbol.PipSize
More here: [/docs/reference/calgo/api/internals/itrade/modifyposition]
Please visit this link to understand more about using Trade and it's member methods:
[/docs/reference/calgo/api/internals/itrade]
@admin
admin
05 Sep 2012, 16:20
This is a sample of how to close all profitable position in your account, from a cBot (cAlgo Robot)
using System; using cAlgo.API; using cAlgo.API.Indicators; namespace cAlgo.Robots { [Robot] public class CloseProfitablePositions : Robot { protected override void OnStart() { foreach (var position in Positions) { if (position.GrossProfit > 0) { ClosePosition(position); } } } } }
@admin
admin
24 Sep 2012, 11:36
Hello,
There is no way for cTrader to know that a Robot is running.
@admin