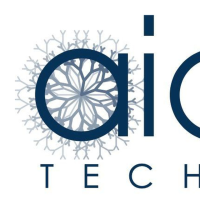
Topics
Replies
PanagiotisChar
10 Apr 2023, 08:05
Hi there,
Can you be more specific? Where do you see this? How can we see this too?
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
10 Apr 2023, 08:04
Hi there,
Unfortunately I cannot tell what happens from a snippet I cannot run. I would need a complete cBot code that I can run and reproduce this behavior.
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
10 Apr 2023, 08:03
Hi there,
This is possible but needs some work. If you are interested in a quote, contact me at development@clickalgo.com
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
10 Apr 2023, 08:01
Hi there,
Here is the code
// -------------------------------------------------------------------------------------------------
//
// This code is a cTrader Automate API example.
//
// This cBot is intended to be used as a sample and does not guarantee any particular outcome or
// profit of any kind. Use it at your own risk.
//
// All changes to this file might be lost on the next application update.
// If you are going to modify this file please make a copy using the "Duplicate" command.
//
// The "Sample RSI cBot" will create a buy order when the Relative Strength Index indicator crosses the level 30,
// and a Sell order when the RSI indicator crosses the level 70. The order is closed be either a Stop Loss, defined in
// the "Stop Loss" parameter, or by the opposite RSI crossing signal (buy orders close when RSI crosses the 70 level
// and sell orders are closed when RSI crosses the 30 level).
//
// The cBot can generate only one Buy or Sell order at any given time.
//
// -------------------------------------------------------------------------------------------------
using System;
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class SampleRSIcBot : Robot
{
[Parameter("Quantity (Lots)", Group = "Volume", DefaultValue = 1, MinValue = 0.01, Step = 0.01)]
public double Quantity { get; set; }
[Parameter("Source", Group = "RSI")]
public DataSeries Source { get; set; }
[Parameter("Periods", Group = "RSI", DefaultValue = 14)]
public int Periods { get; set; }
[Parameter("Buy Level", Group = "RSI", DefaultValue = 14)]
public int BuyLevel { get; set; }
[Parameter("Sell Level", Group = "RSI", DefaultValue = 14)]
public int SellLevel { get; set; }
private RelativeStrengthIndex rsi;
protected override void OnStart()
{
rsi = Indicators.RelativeStrengthIndex(Source, Periods);
}
protected override void OnTick()
{
if (rsi.Result.LastValue < BuyLevel)
{
Close(TradeType.Sell);
Open(TradeType.Buy);
}
else if (rsi.Result.LastValue > SellLevel)
{
Close(TradeType.Buy);
Open(TradeType.Sell);
}
}
private void Close(TradeType tradeType)
{
foreach (var position in Positions.FindAll("SampleRSI", SymbolName, tradeType))
ClosePosition(position);
}
private void Open(TradeType tradeType)
{
var position = Positions.Find("SampleRSI", SymbolName, tradeType);
var volumeInUnits = Symbol.QuantityToVolumeInUnits(Quantity);
if (position == null)
ExecuteMarketOrder(tradeType, SymbolName, volumeInUnits, "SampleRSI");
}
}
}
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
10 Apr 2023, 08:00
Hi there,
Which broker do you use? What is the backtesting data source?
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
10 Apr 2023, 07:57
Hi there,
You can use Stop() method.
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
10 Apr 2023, 07:55
Hi afaha,
We sell one, you can find it below
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
07 Apr 2023, 10:37
( Updated at: 07 Apr 2023, 10:39 )
I guess. This is what usually happens in these cases.
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
07 Apr 2023, 10:03
Probably this is what is going to happen but the damage is done. Software vendors, regulators, authorities can only act retroactively in most cases. The suggestion is to only work with reputable brokers in the future.
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
07 Apr 2023, 09:08
Hi there,
Please share the cBot code that will allow us to reproduce this behavior so that we can explain what happens.
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
07 Apr 2023, 09:01
Traders need to do their due diligence before depositing money to any broker. No matter what Spotware does, it's impossible to filter out a client with malevolent intentions before they commit the wrong doing.
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
06 Apr 2023, 14:21
Hi there,
Try these two
https://clickalgo.com/ctrader-trade-copy
@PanagiotisChar
PanagiotisChar
06 Apr 2023, 08:10
Hi Jay,
You need to wrap your volume with the Symbol.NormalizeVolumeInUnits() method. See below
double newVolume = Symbol.NormalizeVolumeInUnits(currentVolume * 0.66);
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
06 Apr 2023, 08:07
Hi there,
There are free tools available, like this one
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
06 Apr 2023, 08:06
Hi there,
What fees are you referring to, commissions? If yes, then they are paid on each deal execution.
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
06 Apr 2023, 08:05
Hi newbee,
See below
using System;
using System.Linq;
using System.Collections.Generic;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
using cAlgo.Indicators;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class TestMulti : Robot
{
[Parameter("RSI Period", DefaultValue = 14)]
public int Period { get; set; }
// Multi symbol bot test
string[] symbols =
{
"AUDCAD",
"AUDCHF",
"EURUSD",
"CHFJPY",
"EURUSD",
};
List<RSI_Strategy> strategies = new List<RSI_Strategy>();
protected override void OnStart()
{
foreach (var sym in symbols)
{
var rsiStrategy = new RSI_Strategy();
rsiStrategy.init(this, sym);
strategies.Add(rsiStrategy);
}
}
}
// Sell when RSI crosses above 70
public class RSI_Strategy
{
RelativeStrengthIndex rsi;
Robot bot;
string symbol;
public void init(TestMulti bot, string symbol)
{
this.symbol = symbol;
this.bot = bot;
Bars bars = bot.MarketData.GetBars(bot.TimeFrame, symbol);
rsi = bot.Indicators.RelativeStrengthIndex(bars.ClosePrices, bot.Period);
bars.BarOpened += OnBarOpened;
}
void OnBarOpened(BarOpenedEventArgs obj)
{
if (rsi.Result.Last(1) > 70 && rsi.Result.Last(2) <= 70)
{
bot.ExecuteMarketOrder(TradeType.Sell, symbol, 10000, symbol, 10.0, 10.0);
}
if (rsi.Result.Last(1) < 30 && rsi.Result.Last(2) >= 30)
{
bot.ExecuteMarketOrder(TradeType.Buy, symbol, 10000, symbol, 10.0, 10.0);
}
}
}
}
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
06 Apr 2023, 08:00
Hi there,
It would be better to contact your broker for more information about the relevant trade.
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
04 Apr 2023, 08:55
Hi there,
Check here
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
04 Apr 2023, 08:53
Hi there,
In case Pepperstone is your broker, they had some issues yesterday.
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar
PanagiotisChar
10 Apr 2023, 08:10
Hi there,
Here you go
Aieden Technologies
Need help? Join us on Telegram
Need premium support? Trade with us
@PanagiotisChar