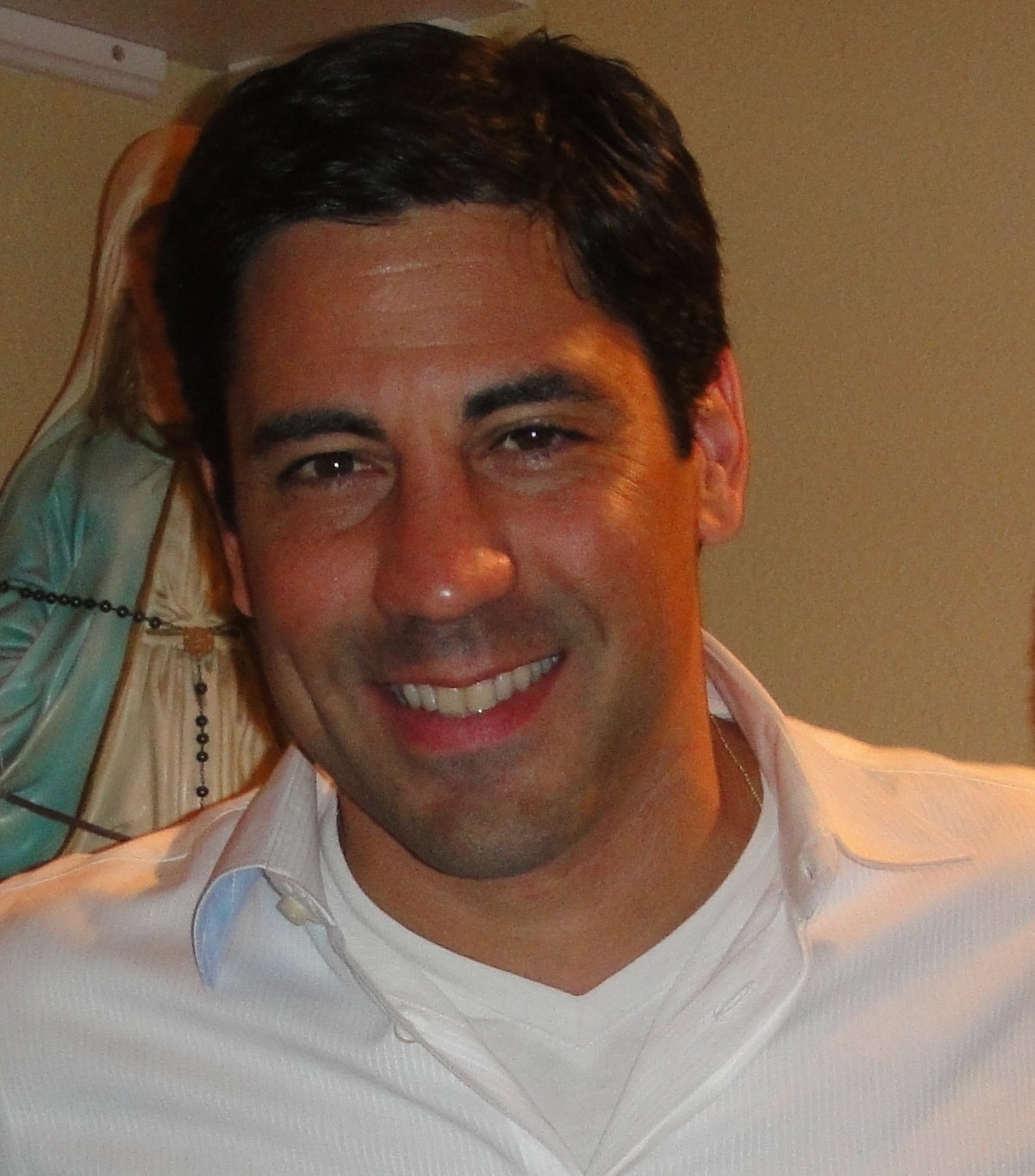
Topics
Replies
lec0456
14 Dec 2012, 22:49
Here is the modified Sample SMA I am using
using System; using cAlgo.API; using cAlgo.API.Indicators; namespace cAlgo.Indicators { [Indicator(IsOverlay = true)] public class SampleSMA : Indicator { [Parameter] public DataSeries Source { get; set; } [Parameter(DefaultValue = 14)] public int Periods { get; set; } [Output("Main", Color = Colors.Turquoise)] public IndicatorDataSeries Result { get; set; } private int PlotCount; protected override void Initialize() { PlotCount=0; } public override void Calculate(int index) { double sum = 0.0; DateTime opentime1 = MarketSeries.OpenTime[index]; for (int i = index - Periods + 1; i <= index; i++) { sum += Source[i]; } Result[index] = sum / Periods; Print("{0,20:MM/dd/yyyy HH:mm}{1,20}{2,20}{3,20}{4,20:#.000000#}",opentime1,index, PlotCount, Result[index],"indicator"); PlotCount++; } } }
@lec0456
lec0456
14 Dec 2012, 22:48
Here is the robot which will print 2 indicator calculations for each on bar event
//#reference: C:\Users\lcespedes\Documents\cAlgo\Sources\Indicators\Sample SMA.algo // ------------------------------------------------------------------------------- // ------------------------------------------------------------------------------- using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot] public class TestIndicatorBot : Robot { private SampleSMA sma; protected override void OnStart() { sma = Indicators.GetIndicator<SampleSMA>(MarketSeries.Close,10); } protected override void OnBar() { if (Trade.IsExecuting) return; //** Indicator calculations and Analysis int t0 = MarketSeries.Close.Count-1;//** t0 results are not final because the bar has not completed int t1 = t0 - 1; int t2 = t1 - 1; if(t2<0){return;}//** prevent crash caused by posibly using a negetive index value DateTime opentime1 = MarketSeries.OpenTime[t1]; if(double.IsNaN(sma.Result[t2])){return;} //** skip printing bar until moving average data is calculated decimal MA1t1 = (decimal) Math.Round(sma.Result[t1],7); decimal MA1t2 = (decimal) Math.Round(sma.Result[t2],7); decimal MA1Slope1 = (MA1t1 - MA1t2)/(decimal)Symbol.PointSize; if(!double.IsNaN(sma.Result[t1])){ Print("{0,20:MM/dd/yyyy HH:mm}{1,20}{2,20}{3,20}{4,20}",opentime1,t1,sma.Result[t1],MA1Slope1,"robot"); } } }//** End of class ********** }//** End of namespace **********
@lec0456
lec0456
14 Dec 2012, 15:52
( Updated at: 23 Jan 2024, 13:11 )
RE:
Please look at the examples in the API Reference for the methods in [ChartObjects]. If you like to DrawText everytime the order is executed you should use this in the OnPositionOpened method.
For example:
protected override void OnPositionOpened(Position openedPosition) { ChartObjects.DrawText("myObject", "text", StaticPosition.TopLeft); }
So, yes, I have this line in my OnPositionOpened. it does not crash but it does not place a B or S on the chart where trades were executed???
ChartObjects.DrawText("Trade" + TradeBarID.ToString(), position.TradeType==TradeType.Buy?"B"+position.Id:"S", MarketSeries.Close.Count-1, position.EntryPrice+3*Symbol.PipSize, VerticalAlignment.Center, HorizontalAlignment.Center, Colors.Yellow);
@lec0456
lec0456
12 Dec 2012, 23:35
( Updated at: 21 Dec 2023, 09:20 )
RE:
Recently cAlgo has become extremely slow. The memory usage is huge. Is this normal? when I first started using it, it was very fast.
looks like it was the broker. when I switched demo accounts cAlgo started to work at normal speed. I notified them of the issue
@lec0456
lec0456
29 Nov 2012, 03:55
RE: RE: RE:
Sure, you need to add a reference to the .net assembly:lec0456 said:Hilec0456 said:Thats ok I figured it out!I am trying to use the System.Data.SqlClient namespace but it gives me an error. It says the namespace does not exist and asks if I am missing an assembly.
Do you know whats wrong?
I was having precisely the same issue as you. Could you share how you did it to make it work? And what database did you use?Thanks
using System.Data.OleDb;
@lec0456
lec0456
27 Nov 2012, 00:17
Well, it still cause cAlgo to "freeze" on my system. The memory consumption goes through the roof over about 1.5 gigs. But I used a work around that seems to work for my purposes, which is to inspect a sample of the data, especially upon starting the robot. I just placed a counter so that it only returns a fixed number of bars. I set it to 100 or 1000 and it works. Don't know why though. But since you say it works on your system maybe its something with my computer. Thanks!
@lec0456
lec0456
25 Nov 2012, 10:18
RE:
@lec0456
lec0456
25 Nov 2012, 03:30
RE:
Right, but I was asking if it was something included in the API like, closedPosition.ClosingPrice. If not I think it should be.I think the close price can be calculated as we know EntryPrice and Pips.
If position is long ClosedPrice = EntryPrice + Pips*PipSize,
if short ClosedPrice = EntryPrice - Pips*PipSize
@lec0456
lec0456
21 Nov 2012, 01:55
RE:
I guess I'm not sure, I have been testing different settings. I will verify and get back to you.Could you please check the settings you are using for the spread? It could be the issue that if you are using live spread then it may trigger an entry at a higher price.
@lec0456
lec0456
14 Dec 2012, 23:02 ( Updated at: 21 Dec 2023, 09:20 )
Here is a better example of the problem: by taking out the print statement in the robot and replacing it with just printing "onBar". You can see that at the same time interval the indicator is printing twice with different values, one before the onbar event and one after???
@lec0456