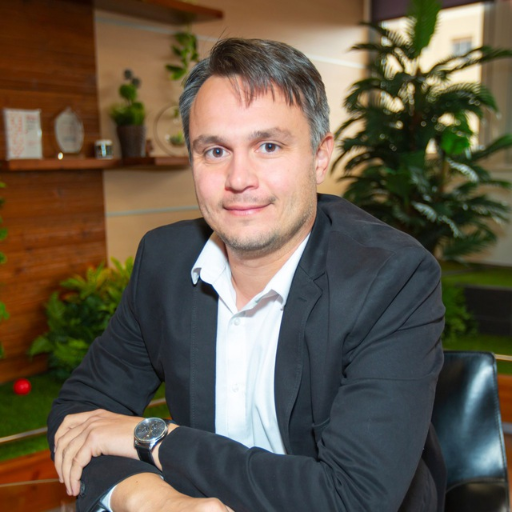
Topics
Replies
PanagiotisCharalampous
22 Feb 2022, 15:24
Hi sirinath,
Yes there can be. There is nothing else you need to do besides the code above.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
22 Feb 2022, 13:28
Hi somersmick,
As per our investigation, the follower's account does not have enough funds to follow the strategy's trades.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
22 Feb 2022, 13:13
Hi there,
Here you go
foreach (var position in Positions)
{
position.ModifyStopLossPips(null);
}
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
22 Feb 2022, 12:21
Dear Shares4UsDevelopment,
Thank you for your honest and constructive feedback. I understand how you feel so I have to provide you with some insight. As you may already read in other discussions, our team is working on migrating the entire cTrader Desktop application to the latest .Net version. This is a humongous task and we have been working on this for the last two years. It is also a task that prevents us from releasing new features until it is completed. We are near completion and we hope that soon there will be announcements. Below there is a list of major improvements just to prove that we are not ignoring developers at all
- Upgrade to the latest .Net Runtime
- Out of process algo execution
- Any IDE support (no more VS dependencies)
- New Built in Code Editor
- New installer
- Backtesting and optimization improvements
- OnDestroy method for indicators
- Improved logs
and many more. After the above are delivered, we will be able to start implementing the features pending in the backlog, like renko and range backtesting and many more.
We are also developing a brand new ctrader.com hence we are giving less attention to the existing one :)
As far as developer support is concerned, I am 100% sure that we have the best community team out there!
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
22 Feb 2022, 11:50
Hi peak2020,
We were not able to reproduce this issue. Are you able to reproduce this consistently? If yes, are you available for a TeamViewer session where an engineer can inspect the issue on your computer?
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
22 Feb 2022, 08:47
Hi bugazzza,
We are working on a fix. It should be released next weekend.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 17:07
Hi peak2020,
We tried to reproduce the problem but we couldn't. In the meantime, a new version has been released on FxPro. Can you check if this still happens?
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 15:54
Hi Florent,
Yes you can. You can even do this on the same VPS.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 15:23
Hi Florent,
I don't understand what you mean when you say "VPS 1 linked...". A VPS is not linked to anything by its own. If you launch cTrader on VPS 1 and login with cTrader ID 1, then you will have access to cTrader ID 1 accounts. If you login with cTrader ID 2, cTrader ID 2 accounts. If you login to both cTrader IDs, then you will have access to both.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 15:09
Hi Florent,
I still have a hard time understanding your question. A VPS is just a computer running a OS, Windows in this case. It works exactly as your laptop. You can operate it as you wish. You can open as many instances of cTrader you want and login to as many cTrader IDs you wish.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 14:41
Hi Florent,
I am not sure I understand your question. On each VPS you can login with any cTrader ID you want.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 11:49
Hi peak2020,
The team is investigating this issue.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 11:48
Hi roan.is,
This is a known bug and we are working on a fix.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 11:47
Hi peak2020,
The video has been deleted. Can you please send it again?
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 11:46
Hi somersmick,
Please provide us with the following information
- Strategy name
- Follower's account number and brokers
- Screenshots of strategy deals and followers deals showing the missing deals you are referring to.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 11:39
Hi auspall,
It's a bug and the team is working on a fix.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 08:21
Hi shikuagnes21,
You can share your suggestions for cTrader Copy in the Suggestions section.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 08:20
Hi ja.idzikowski,
Can you please provide some more information regarding this issue e.g. some screenshots or a video?
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
21 Feb 2022, 08:13
Hi ctid2082553,
Please post suggestions in the Suggestions section. Also this can be easily implemented as an indicator.
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous
PanagiotisCharalampous
22 Feb 2022, 17:20
Hi Bjorn,
Your problem has nothing to do with lazy loading. Instead this happens because of a poorly programmed indicator. You problem is here.
You cannot do this since the Calculate is called many times during a current candle's life. It is clearly described here. This variable will just produce nonsense. What you actually need to do is to keep the state of the last selection. Here is a very rough solution. The code is still poor but I do not have the time to rewrite the entire indicator. It's just an example of how you need to handle this
Here is the full source code
and here are the results
Best Regards,
Panagiotis
Join us on Telegram and Facebook
@PanagiotisCharalampous