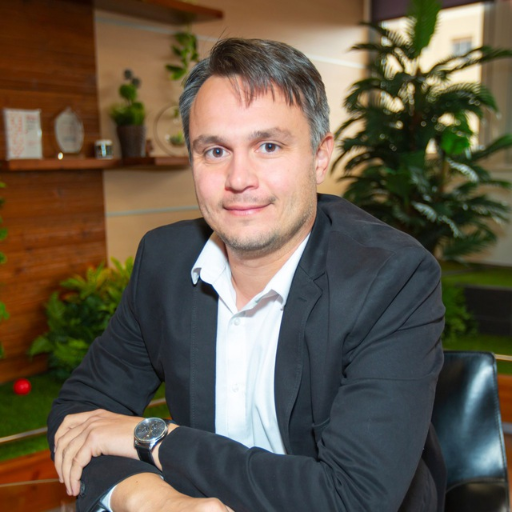
Topics
Replies
PanagiotisCharalampous
13 Jun 2019, 14:49
Hi Bjorn,
Here it is
using System; using System.Linq; using System.Text; using cAlgo.API; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class DayTradeG1 : Robot { [Parameter("News Hour", DefaultValue = 14, MinValue = 0, MaxValue = 23)] public int NewsHour { get; set; } [Parameter("News Minute", DefaultValue = 30, MinValue = 0, MaxValue = 59)] public int NewsMinute { get; set; } [Parameter("Pips away", DefaultValue = 10)] public int PipsAway { get; set; } [Parameter("Take Profit", DefaultValue = 50)] public int TakeProfit { get; set; } [Parameter("Stop Loss", DefaultValue = 10)] public int StopLoss { get; set; } [Parameter("Volume", DefaultValue = 100000, MinValue = 10)] public int Volume { get; set; } [Parameter("Seconds Before", DefaultValue = 10, MinValue = 1)] public int SecondsBefore { get; set; } [Parameter("Seconds Timeout", DefaultValue = 10, MinValue = 1)] public int SecondsTimeout { get; set; } [Parameter("One Cancels Other")] public bool Oco { get; set; } [Parameter("ShowTimeLeftNews", DefaultValue = false)] public bool ShowTimeLeftToNews { get; set; } [Parameter("ShowTimeLeftPlaceOrders", DefaultValue = true)] public bool ShowTimeLeftToPlaceOrders { get; set; } [Parameter("Label", DefaultValue = "Label")] public string Label { get; set; } private bool _ordersCreated; private DateTime _triggerTimeInServerTimeZone; protected override void OnStart() { Positions.Opened += OnPositionOpened; Timer.Start(1); var triggerTimeInLocalTimeZone = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, NewsHour, NewsMinute, 0); if (triggerTimeInLocalTimeZone < DateTime.Now) triggerTimeInLocalTimeZone = triggerTimeInLocalTimeZone.AddDays(1); _triggerTimeInServerTimeZone = TimeZoneInfo.ConvertTime(triggerTimeInLocalTimeZone, TimeZoneInfo.Local, TimeZone); } protected override void OnTimer() { var remainingTime = _triggerTimeInServerTimeZone - Server.Time; DrawRemainingTime(remainingTime); if (!_ordersCreated) { var sellOrderTargetPrice = Symbol.Bid - PipsAway * Symbol.PipSize; ChartObjects.DrawHorizontalLine("sell target", sellOrderTargetPrice, Colors.Red, 1, LineStyle.DotsVeryRare); var buyOrderTargetPrice = Symbol.Ask + PipsAway * Symbol.PipSize; ChartObjects.DrawHorizontalLine("buy target", buyOrderTargetPrice, Colors.Blue, 1, LineStyle.DotsVeryRare); if (Server.Time <= _triggerTimeInServerTimeZone && (_triggerTimeInServerTimeZone - Server.Time).TotalSeconds <= SecondsBefore) { _ordersCreated = true; var expirationTime = _triggerTimeInServerTimeZone.AddSeconds(SecondsTimeout); PlaceStopOrder(TradeType.Sell, Symbol, Volume, sellOrderTargetPrice, Label, StopLoss, TakeProfit, expirationTime); PlaceStopOrder(TradeType.Buy, Symbol, Volume, buyOrderTargetPrice, Label, StopLoss, TakeProfit, expirationTime); ChartObjects.RemoveObject("sell target"); ChartObjects.RemoveObject("buy target"); } } if (_ordersCreated && !PendingOrders.Any(o => o.Label == Label)) { Print("Orders expired"); Stop(); } } private void DrawRemainingTime(TimeSpan remainingTimeToNews) { if (ShowTimeLeftToNews) { if (remainingTimeToNews > TimeSpan.Zero) { ChartObjects.DrawText("countdown1", "Time left to news: " + FormatTime(remainingTimeToNews), StaticPosition.TopLeft); } else { ChartObjects.RemoveObject("countdown1"); } } if (ShowTimeLeftToPlaceOrders) { var remainingTimeToOrders = remainingTimeToNews - TimeSpan.FromSeconds(SecondsBefore); if (remainingTimeToOrders > TimeSpan.Zero) { ChartObjects.DrawText("countdown2", "Time left to place orders: " + FormatTime(remainingTimeToOrders), StaticPosition.TopRight); } else { ChartObjects.RemoveObject("countdown2"); } } } private static StringBuilder FormatTime(TimeSpan remainingTime) { var remainingTimeStr = new StringBuilder(); if (remainingTime.TotalHours >= 1) remainingTimeStr.Append((int)remainingTime.TotalHours + "h "); if (remainingTime.TotalMinutes >= 1) remainingTimeStr.Append(remainingTime.Minutes + "m "); if (remainingTime.TotalSeconds > 0) remainingTimeStr.Append(remainingTime.Seconds + "s"); return remainingTimeStr; } private void OnPositionOpened(PositionOpenedEventArgs args) { var position = args.Position; if (position.Label == Label && position.SymbolCode == Symbol.Code) { if (Oco) { foreach (var order in PendingOrders) { if (order.Label == Label && order.SymbolCode == Symbol.Code) { CancelPendingOrderAsync(order); } } } Stop(); } } } }
If you need assistance in changing your cBots, you can always reach out to a Consultant.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
13 Jun 2019, 14:29
Hi Bjorn,
You set a label to each order but is constant
private const string Label = "News Straddler v1";
Your code filters out orders based on symbol and label
foreach (var order in PendingOrders) { if (order.Label == Label && order.SymbolCode == Symbol.Code) { CancelPendingOrderAsync(order); } }
You should make Label a parameter and set a different one for each instance so that each instance operates only on its orders.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
13 Jun 2019, 14:22
Hi diegorcirelli,
I just tried it and it works. I don't see anything wrong.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
13 Jun 2019, 14:11
Hi bjornvandekerkhof,
Thanks for posting in our forum. You should try setting a different label for each instance's orders.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
13 Jun 2019, 10:05
Hi exane,
Can you please send us troubleshooting information next time this happens? To do so, please press Ctrl+Alt+Shift+T, paste a link to this discussion in the textbox and then press Submit.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
13 Jun 2019, 10:02
Hi FireMyst,
If you can share the cBot code and cBot parameters we can investigate further.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
13 Jun 2019, 09:57
Hi FireMyst,
No there is no such option at the moment.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
13 Jun 2019, 09:55
Hi calgodemo,
Can you share the cBot and Indicator code?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
13 Jun 2019, 09:52
Hi diegorcirelli,
If you need assistance in developing your cBot, I would advise you to contact a Consultant or post a Job.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
13 Jun 2019, 09:49
Hi mo798ua,
At the moment there is no suvh functionality in the mobile application.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jun 2019, 16:00
Hi,
Here is a more correct condition
if (Positions.Count(x => x.SymbolCode == Symbol.Code && x.Label == Label && x.TradeType == TradeType.Buy) > 0)
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jun 2019, 15:16
Hi diegorcirelli,
Here is an attempt to guess what you are trying to do
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class MeuMesmo : Robot { [Parameter("Volume", DefaultValue = 1)] public double Vol { get; set; } [Parameter("StopLoss", DefaultValue = 1000)] public double SL { get; set; } [Parameter("TakeProfit", DefaultValue = 100)] public double TP { get; set; } [Parameter("Distância", DefaultValue = 100)] public double Pips { get; set; } private readonly string Label = "MeuMesmo"; protected override void OnStart() { ExecuteMarketOrder(TradeType.Buy, Symbol, Vol, Label, SL, TP); } protected override void OnTick() { if (Positions.Count > 0) { var highestPriceBuy = Positions.FindAll(Label, Symbol, TradeType.Buy).Max(x => x.EntryPrice); if (highestPriceBuy + (Symbol.PipValue * Pips) <= Symbol.Bid) ExecuteMarketOrder(TradeType.Buy, Symbol, Vol, Label, SL, TP); var lowestPriceBuy = Positions.FindAll(Label, Symbol, TradeType.Buy).Min(x => x.EntryPrice); if (lowestPriceBuy - (Symbol.PipValue * Pips) >= Symbol.Bid) ExecuteMarketOrder(TradeType.Buy, Symbol, Vol, Label, SL, TP); } } protected override void OnStop() { // Put your deinitialization logic here } } }
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jun 2019, 15:00
Ηι diegorcirelli,
If you run the cBot as is, it will not do anything as there are no open positions in the first place. When the cBot starts, from which position do you expect it to measure the distance since there is none open?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jun 2019, 14:36
Hi diegorcirelli,
Can you provide enough information to reproduce what you are seeing? We need
- The full cBot code
- Backtesting parameters
- Date and time where you would expect the trade to happed but does not.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jun 2019, 10:26
RE:
diegorcirelli said:
Good evening ... now a little developed this code, but also did not work, could you help me ??
protected override void OnTick() { var highestPriceBuy = Positions.FindAll(Label, Symbol, TradeType.Buy).Max(x => x.EntryPrice); if (highestPriceBuy + Pips <= Symbol.Bid) ExecuteMarketOrder(TradeType.Buy, Symbol, Vol, Label, SL, TP); }
Hi diegorcirelli,
I do not see any problem in this code. Can you please explain to us why you think it is not working? What do you expect it to do and what does it do instead?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jun 2019, 09:35
Hi bitmext,
Thanks for posting in our forum and for your nice feedback about the platform. There is no feature in the interface that would allow you to do so but you can achieve this by running a simple cBot. See the source code below
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class ReversePositions : Robot { protected override void OnStart() { foreach (var position in Positions) position.Reverse(); Stop(); } protected override void OnTick() { // Put your core logic here } protected override void OnStop() { // Put your deinitialization logic here } } }
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
12 Jun 2019, 09:28
Hi zedodia,
Probably you are not passing the correct parameters to one of the indicators? Which is the VWAP indicator you are using?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
11 Jun 2019, 09:49
Hi martin100181@gmail.com,
Thanks for posting in our forum. See below
var targetPrice = PendingOrders.First(x => x.Label == "Long_1").TargetPrice;
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
11 Jun 2019, 09:42
Hi David,
You can always contact a Consultant to help you with your cBot.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
13 Jun 2019, 15:01
Hi diegorcirelli,
Check your volume. The mimimum for EURUSD is 1000.
Best Regards,
Panagiotis
@PanagiotisCharalampous