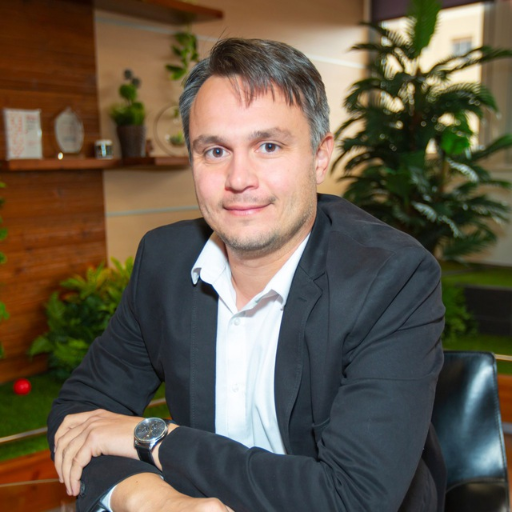
Topics
Replies
PanagiotisCharalampous
19 Sep 2019, 12:41
Hi ctid1541941,
When we say we do not support Chromium or Linux (or any other browser/OS not on the list), it means that we do not test cTrader on these environment neither we fix bugs specific to them. However this does not mean that you cannot use them and they work fine 99.9% of the cases. But if you want a fully functional platform, use one of the supported environments.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
19 Sep 2019, 12:33
Here it is
using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; using cAlgo.Indicators; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class NewIndicator : Indicator { [Parameter(DefaultValue = 14)] public int Periods { get; set; } [Parameter(DefaultValue = 50)] public double Level { get; set; } [Output("Main")] public IndicatorDataSeries MA { get; set; } [Output("Leven Up")] public IndicatorDataSeries LevelUp { get; set; } [Output("Leven Down")] public IndicatorDataSeries LevelDown { get; set; } SimpleMovingAverage _sma; protected override void Initialize() { _sma = Indicators.SimpleMovingAverage(MarketSeries.Close, Periods); } public override void Calculate(int index) { // Calculate value at specified index MA[index] = _sma.Result[index]; LevelUp[index] = _sma.Result[index] + Symbol.TickSize * Level; LevelDown[index] = _sma.Result[index] - Symbol.TickSize * Level; } } }
@PanagiotisCharalampous
PanagiotisCharalampous
19 Sep 2019, 11:53
( Updated at: 21 Dec 2023, 09:21 )
Hi darcome,
This is already available using the Market Snapshot. See below
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
19 Sep 2019, 11:45
Hi beej.sanchez,
I used pips but it seems that MT4 uses points. It can be easily modified if you wish.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
19 Sep 2019, 11:31
Hi Wiktor,
You can reset the sequence number whenever you want using 35=4. Check the documentation.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
19 Sep 2019, 11:24
Hi ctid1541941,
Please note that Chromium and Linux are not supported for cTrader Web.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
19 Sep 2019, 11:10
Hi beej.sanchez,
There is no such feature in cTrader but you can easily achieve this with a custom indicator. See below an example
using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; using cAlgo.Indicators; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class NewIndicator : Indicator { [Parameter(DefaultValue = 14)] public int Periods { get; set; } [Parameter(DefaultValue = 5)] public double Level { get; set; } [Output("Main")] public IndicatorDataSeries MA { get; set; } [Output("Leven Up")] public IndicatorDataSeries LevelUp { get; set; } [Output("Leven Down")] public IndicatorDataSeries LevelDown { get; set; } SimpleMovingAverage _sma; protected override void Initialize() { _sma = Indicators.SimpleMovingAverage(MarketSeries.Close, Periods); } public override void Calculate(int index) { // Calculate value at specified index MA[index] = _sma.Result[index]; LevelUp[index] = _sma.Result[index] + Symbol.PipSize * Level; LevelDown[index] = _sma.Result[index] - Symbol.PipSize * Level; } } }
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
19 Sep 2019, 08:39
Hi Matt,
To be able to give advice you need to share with us the cBot code, cBot parameters and exact steps to reproduce these issues.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
19 Sep 2019, 08:37
Hi Meer,
Thanks for posting in our forum. Please use the Suggestions section for posting such suggestions.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
18 Sep 2019, 09:29
Hi chim,
The fix is expected in v3.7.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
18 Sep 2019, 08:43
Hi tgjobscv,
Even thoubh adding more indicators in cTrader Web and cTrader Mobile is in our future plans, there is no such plan for the upcoming versions yet.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
17 Sep 2019, 16:47
Hi chim,
This is a known issue and will be fixedin an update. A workaround is to zoom in and then drag the time counter on the bottom axis left and right and past data will be retrieved. Let me know if this helps.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
17 Sep 2019, 14:49
Hi Bhavin Dholakia,
See below an example
using System; using System.Linq; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)] public class NewcBot : Robot { ExponentialMovingAverage _ema; bool _askBelowPrice; bool _bidAbovePrice; protected override void OnStart() { _ema = Indicators.ExponentialMovingAverage(MarketSeries.Close, 28); _askBelowPrice = Symbol.Ask >= _ema.Result.LastValue; _bidAbovePrice = Symbol.Bid <= _ema.Result.LastValue; } protected override void OnTick() { if (Symbol.Ask <= _ema.Result.LastValue) { if (!_askBelowPrice) ExecuteMarketOrder(TradeType.Buy, Symbol.Name, 1000); _askBelowPrice = true; } else { _askBelowPrice = false; } if (Symbol.Bid >= _ema.Result.LastValue) { if (!_bidAbovePrice) ExecuteMarketOrder(TradeType.Sell, Symbol.Name, 1000); _bidAbovePrice = true; } else { _bidAbovePrice = false; } } protected override void OnStop() { // Put your deinitialization logic here } } }
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
17 Sep 2019, 14:00
Hi Xavier,
All fonts installed on your PC are supported. You can specify multiple fonts seperated by comma. The first font found will be used or it will fall back to the default one, if no fonts found.
Regarding the question about the grid. RemoveRowAt and RemoveColumnAt do not remove child elements. E.g. if you remove the third row, the child in this row will be moved to the second one.
RemoveChild() method has only a child parameter. A child element will be removed regardless of the row and column. So for RemoveChild there is no need to know about row and column of the child
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
17 Sep 2019, 12:51
Hi Bhavin Dholakia,
HasCrossedAbove and HasCrossedBelow tell you the direction of crossing. If you want to implement this on a tick based strategy you can compare the value of the ema with the previous and with the current tick. If you clarify what do you need exactly, I can provide you with an example.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
17 Sep 2019, 09:15
Hi wiktor,
Can you add tag 50 as well and let me know if it works?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
17 Sep 2019, 08:14
Hi Bhavin Dholakia,
Thanks for posting in our forum. Why do you need a special function for this? Current prices can be found in Symbol.Bid and Symbol.Ask and the latest EMA value can be found in the ema.Result.LastValue property. You can use them to make any comparison you like.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
17 Sep 2019, 07:59
Hi Cam_maC,
Can you share the cBot code so that we can have a look?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
16 Sep 2019, 14:13
Hi Vince,
Is it possible to arrange a TeamViewer session? In order to investigate this issue further we need to check your computer. If yes, please send us an email at community@spotware.com to arrange it.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
19 Sep 2019, 15:55
Hi Pedro,
Indeed this is true. See below a workaround
Best Regards,
Panagiotis
@PanagiotisCharalampous