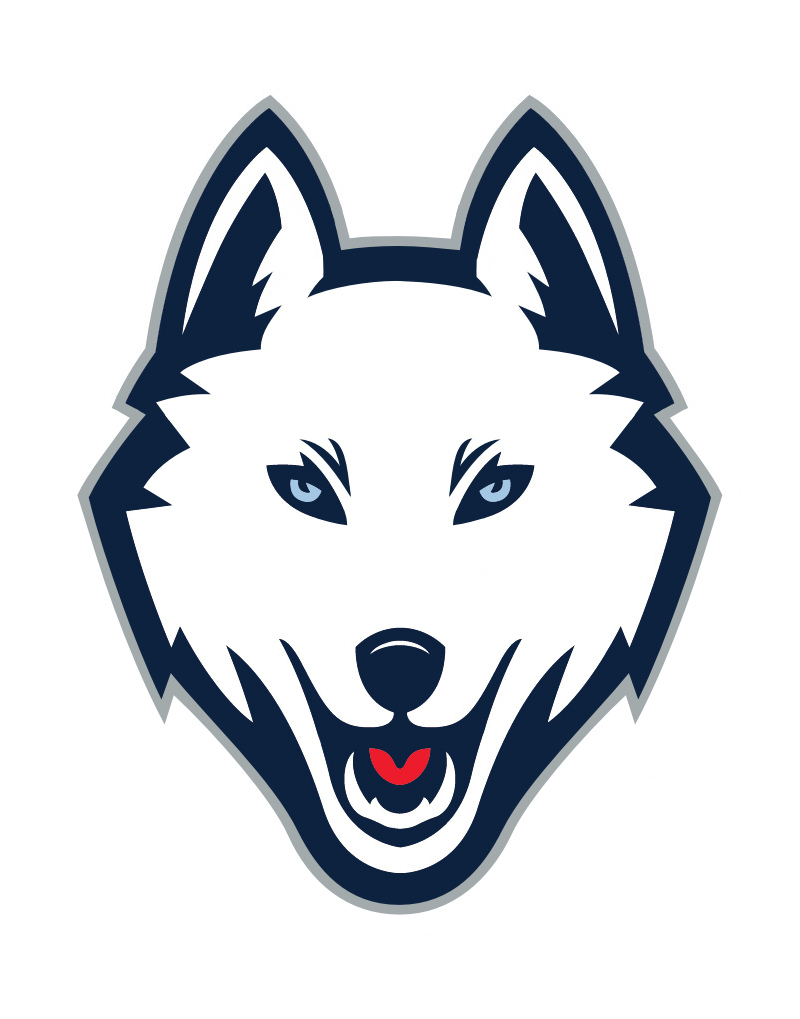
Multi-timeframe MA lines ...
12 Oct 2013, 14:00
Hello,
Why if I move the MA from timeframe m15 on the m10 chart, moving averages lines are draw incorrectly?
m10
code:
//================================================ // boomood3MA //================================================ using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Indicators { [Indicator(IsOverlay = true, AutoRescale = false)] public class boomood3MA : Indicator { public int LineBold; public Colors LineColor; private int Period = 20; private MovingAverage expo; private MovingAverage expo1; private MovingAverage expo2; private MovingAverage expo3; [Parameter(DefaultValue = 1)] public bool MAx3Set { get; set; } [Parameter(DefaultValue = 50)] public int MA1Period { get; set; } [Parameter(DefaultValue = 100)] public int MA2Period { get; set; } [Parameter(DefaultValue = 200)] public int MA3Period { get; set; } [Parameter(DefaultValue = 1)] public bool RainbowSet { get; set; } [Parameter(DefaultValue = 400)] public int EnvelopePeriod { get; set; } [Parameter(DefaultValue = 0.25)] public double BandDistance1 { get; set; } [Parameter(DefaultValue = 0.45)] public double BandDistance2 { get; set; } [Parameter(DefaultValue = 0.6)] public double BandDistance3 { get; set; } [Parameter(DefaultValue = 0.8)] public double BandDistance4 { get; set; } [Parameter(DefaultValue = 1.0)] public double BandDistance5 { get; set; } [Parameter(DefaultValue = 1.7)] public double BandDistance6 { get; set; } [Output("MA1", Color = Colors.Aqua)] public IndicatorDataSeries MA1 { get; set; } [Parameter("MA1Type", DefaultValue = 5)] public MovingAverageType matype1 { get; set; } [Output("MA2", Color = Colors.Red)] public IndicatorDataSeries MA2 { get; set; } [Parameter("MA2Type", DefaultValue = 5)] public MovingAverageType matype2 { get; set; } [Output("MA3", Color = Colors.Yellow)] public IndicatorDataSeries MA3 { get; set; } [Parameter("MA3Type", DefaultValue = 5)] public MovingAverageType matype3 { get; set; } [Output("MainMA")] public IndicatorDataSeries EnvelopeMain { get; set; } [Output("ChannelUp1", Color = Colors.Aqua)] public IndicatorDataSeries ChannelUp1 { get; set; } [Output("ChannelUp2", Color = Colors.Teal)] public IndicatorDataSeries ChannelUp2 { get; set; } [Output("ChannelUp3", Color = Colors.Yellow)] public IndicatorDataSeries ChannelUp3 { get; set; } [Output("ChannelUp4", Color = Colors.OrangeRed)] public IndicatorDataSeries ChannelUp4 { get; set; } [Output("ChannelUp5", Color = Colors.Red)] public IndicatorDataSeries ChannelUp5 { get; set; } [Output("ChannelUp6", Color = Colors.Red)] public IndicatorDataSeries ChannelUp6 { get; set; } [Output("ChannelLow1", Color = Colors.Aqua)] public IndicatorDataSeries ChannelLow1 { get; set; } [Output("ChannelLow2", Color = Colors.Teal)] public IndicatorDataSeries ChannelLow2 { get; set; } [Output("ChannelLow3", Color = Colors.Yellow)] public IndicatorDataSeries ChannelLow3 { get; set; } [Output("ChannelLow4", Color = Colors.OrangeRed)] public IndicatorDataSeries ChannelLow4 { get; set; } [Output("ChannelLow5", Color = Colors.Red)] public IndicatorDataSeries ChannelLow5 { get; set; } [Output("ChannelLow6", Color = Colors.Red)] public IndicatorDataSeries ChannelLow6 { get; set; } [Parameter("MainMAType", DefaultValue = 5)] public MovingAverageType matype { get; set; } [Parameter(DefaultValue = 0)] public bool Set100Levels { get; set; } // line bold [Parameter(DefaultValue = 1)] public bool L1 { get; set; } [Parameter(DefaultValue = 0)] public bool L2 { get; set; } [Parameter(DefaultValue = 0)] public bool L3 { get; set; } [Parameter(DefaultValue = 0)] public bool L4 { get; set; } // ===== linr color [Parameter(DefaultValue = 1)] public bool SetRed { get; set; } [Parameter(DefaultValue = 0)] public bool SetWhite { get; set; } [Parameter(DefaultValue = 0)] public bool SetBlack { get; set; } [Parameter(DefaultValue = 0)] public bool SetGreen { get; set; } private MarketSeries m15; protected override void Initialize() { m15 = MarketData.GetSeries(TimeFrame.Minute15); expo = Indicators.MovingAverage(m15.Close, EnvelopePeriod, matype); //expo = Indicators.MovingAverage(MarketSeries.Close, EnvelopePeriod, matype); expo1 = Indicators.MovingAverage(MarketSeries.Close, MA1Period, matype1); expo2 = Indicators.MovingAverage(MarketSeries.Close, MA2Period, matype2); expo3 = Indicators.MovingAverage(MarketSeries.Close, MA3Period, matype3); } public override void Calculate(int index) { if (RainbowSet) { EnvelopeMain[index] = expo.Result[index]; ChannelUp1[index] = expo.Result[index] + (expo.Result[index] * BandDistance1) / 100; ChannelUp2[index] = expo.Result[index] + (expo.Result[index] * BandDistance2) / 100; ChannelUp3[index] = expo.Result[index] + (expo.Result[index] * BandDistance3) / 100; ChannelUp4[index] = expo.Result[index] + (expo.Result[index] * BandDistance4) / 100; ChannelUp5[index] = expo.Result[index] + (expo.Result[index] * BandDistance5) / 100; ChannelUp6[index] = expo.Result[index] + (expo.Result[index] * BandDistance6) / 100; ChannelLow1[index] = expo.Result[index] - (expo.Result[index] * BandDistance1) / 100; ChannelLow2[index] = expo.Result[index] - (expo.Result[index] * BandDistance2) / 100; ChannelLow3[index] = expo.Result[index] - (expo.Result[index] * BandDistance3) / 100; ChannelLow4[index] = expo.Result[index] - (expo.Result[index] * BandDistance4) / 100; ChannelLow5[index] = expo.Result[index] - (expo.Result[index] * BandDistance5) / 100; ChannelLow6[index] = expo.Result[index] - (expo.Result[index] * BandDistance6) / 100; } if (MAx3Set) { MA1[index] = expo1.Result[index]; MA2[index] = expo2.Result[index]; MA3[index] = expo3.Result[index]; } if (L1) { LineBold = 1; } if (L2) { LineBold = 2; } if (L3) { LineBold = 3; } if (L4) { LineBold = 4; } if (SetRed) { LineColor = Colors.Red; } if (SetWhite) { LineColor = Colors.White; } if (SetBlack) { LineColor = Colors.Black; } if (SetGreen) { LineColor = Colors.YellowGreen; } if (Set100Levels) { for (int i = 1; i < 200; i++) { ChartObjects.DrawHorizontalLine("LineLevel" + i, i * 100 * Symbol.PipSize, LineColor, LineBold); } } } } }
Did I get something wrong or ... ?
Thanks and bye.
Replies
breakermind
14 Oct 2013, 13:25
RE:
Spotware said:
You need to get the index that corresponds to the series that is in a different Timeframe. See the example: /forum/whats-new/1463#3
Hi,
that's what it says:
m15 = MarketData.GetSeries(TimeFrame.Minute15); expo = Indicators.MovingAverage (m15.Close, EnvelopePeriod, matype);
still not working?
Thanks
@breakermind
Spotware
14 Oct 2013, 14:17
Please look at the whole example.
You need to find which index corresponds to the series which is of a different Timeframe than the instance of your indicator.
So, use the method GetIndexByDate as in the example to find the index to use in your m15 series "expo".
@Spotware
breakermind
14 Oct 2013, 15:04
RE:
Spotware said:
Please look at the whole example.
You need to find which index corresponds to the series which is of a different Timeframe than the instance of your indicator.
So, use the method GetIndexByDate as in the example to find the index to use in your m15 series "expo".
Thanks it works :]
//================================================ // boomood3MA //================================================ using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Indicators { [Indicator(IsOverlay = true, AutoRescale = false)] public class boomood3MA : Indicator { public int LineBold; public Colors LineColor; private int Period = 20; private MovingAverage expo; private MovingAverage expo1; private MovingAverage expo2; private MovingAverage expo3; [Parameter(DefaultValue = 1)] public bool MAx3Set { get; set; } [Parameter(DefaultValue = 50)] public int MA1Period { get; set; } [Parameter(DefaultValue = 100)] public int MA2Period { get; set; } [Parameter(DefaultValue = 200)] public int MA3Period { get; set; } [Parameter(DefaultValue = 1)] public bool RainbowSet { get; set; } [Parameter(DefaultValue = 0)] public bool M15_RainbowSet { get; set; } [Parameter(DefaultValue = 400)] public int EnvelopePeriod { get; set; } [Parameter(DefaultValue = 0.25)] public double BandDistance1 { get; set; } [Parameter(DefaultValue = 0.45)] public double BandDistance2 { get; set; } [Parameter(DefaultValue = 0.6)] public double BandDistance3 { get; set; } [Parameter(DefaultValue = 0.8)] public double BandDistance4 { get; set; } [Parameter(DefaultValue = 1.0)] public double BandDistance5 { get; set; } [Parameter(DefaultValue = 1.7)] public double BandDistance6 { get; set; } [Output("MA1", Color = Colors.Aqua)] public IndicatorDataSeries MA1 { get; set; } [Parameter("MA1Type", DefaultValue = 5)] public MovingAverageType matype1 { get; set; } [Output("MA2", Color = Colors.Red)] public IndicatorDataSeries MA2 { get; set; } [Parameter("MA2Type", DefaultValue = 5)] public MovingAverageType matype2 { get; set; } [Output("MA3", Color = Colors.Yellow)] public IndicatorDataSeries MA3 { get; set; } [Parameter("MA3Type", DefaultValue = 5)] public MovingAverageType matype3 { get; set; } [Output("MainMA")] public IndicatorDataSeries EnvelopeMain { get; set; } [Output("ChannelUp1", Color = Colors.Aqua)] public IndicatorDataSeries ChannelUp1 { get; set; } [Output("ChannelUp2", Color = Colors.Teal)] public IndicatorDataSeries ChannelUp2 { get; set; } [Output("ChannelUp3", Color = Colors.Yellow)] public IndicatorDataSeries ChannelUp3 { get; set; } [Output("ChannelUp4", Color = Colors.OrangeRed)] public IndicatorDataSeries ChannelUp4 { get; set; } [Output("ChannelUp5", Color = Colors.Red)] public IndicatorDataSeries ChannelUp5 { get; set; } [Output("ChannelUp6", Color = Colors.Red)] public IndicatorDataSeries ChannelUp6 { get; set; } [Output("ChannelLow1", Color = Colors.Aqua)] public IndicatorDataSeries ChannelLow1 { get; set; } [Output("ChannelLow2", Color = Colors.Teal)] public IndicatorDataSeries ChannelLow2 { get; set; } [Output("ChannelLow3", Color = Colors.Yellow)] public IndicatorDataSeries ChannelLow3 { get; set; } [Output("ChannelLow4", Color = Colors.OrangeRed)] public IndicatorDataSeries ChannelLow4 { get; set; } [Output("ChannelLow5", Color = Colors.Red)] public IndicatorDataSeries ChannelLow5 { get; set; } [Output("ChannelLow6", Color = Colors.Red)] public IndicatorDataSeries ChannelLow6 { get; set; } [Parameter("MainMAType", DefaultValue = 5)] public MovingAverageType matype { get; set; } [Parameter(DefaultValue = 0)] public bool Set100Levels { get; set; } // line bold [Parameter(DefaultValue = 1)] public bool L1 { get; set; } [Parameter(DefaultValue = 0)] public bool L2 { get; set; } [Parameter(DefaultValue = 0)] public bool L3 { get; set; } [Parameter(DefaultValue = 0)] public bool L4 { get; set; } // ===== linr color [Parameter(DefaultValue = 1)] public bool SetRed { get; set; } [Parameter(DefaultValue = 0)] public bool SetWhite { get; set; } [Parameter(DefaultValue = 0)] public bool SetBlack { get; set; } [Parameter(DefaultValue = 0)] public bool SetGreen { get; set; } private MarketSeries m15; protected override void Initialize() { m15 = MarketData.GetSeries(TimeFrame.Minute15); expo = Indicators.MovingAverage(m15.Close, EnvelopePeriod, matype); //expo = Indicators.MovingAverage(MarketSeries.Close, EnvelopePeriod, matype); expo1 = Indicators.MovingAverage(MarketSeries.Close, MA1Period, matype1); expo2 = Indicators.MovingAverage(MarketSeries.Close, MA2Period, matype2); expo3 = Indicators.MovingAverage(MarketSeries.Close, MA3Period, matype3); } public override void Calculate(int index) { if (RainbowSet) { EnvelopeMain[index] = expo.Result[index]; ChannelUp1[index] = expo.Result[index] + (expo.Result[index] * BandDistance1) / 100; ChannelUp2[index] = expo.Result[index] + (expo.Result[index] * BandDistance2) / 100; ChannelUp3[index] = expo.Result[index] + (expo.Result[index] * BandDistance3) / 100; ChannelUp4[index] = expo.Result[index] + (expo.Result[index] * BandDistance4) / 100; ChannelUp5[index] = expo.Result[index] + (expo.Result[index] * BandDistance5) / 100; ChannelUp6[index] = expo.Result[index] + (expo.Result[index] * BandDistance6) / 100; ChannelLow1[index] = expo.Result[index] - (expo.Result[index] * BandDistance1) / 100; ChannelLow2[index] = expo.Result[index] - (expo.Result[index] * BandDistance2) / 100; ChannelLow3[index] = expo.Result[index] - (expo.Result[index] * BandDistance3) / 100; ChannelLow4[index] = expo.Result[index] - (expo.Result[index] * BandDistance4) / 100; ChannelLow5[index] = expo.Result[index] - (expo.Result[index] * BandDistance5) / 100; ChannelLow6[index] = expo.Result[index] - (expo.Result[index] * BandDistance6) / 100; } if (M15_RainbowSet) { RainbowSet = false; //EnvelopeMain[index] = expo.Result[index]; var index15 = GetIndexByDate(m15, MarketSeries.OpenTime[index]); if (index15 != -1) EnvelopeMain[index] = expo.Result[index15]; ChannelUp1[index] = expo.Result[index15] + (expo.Result[index15] * BandDistance1) / 100; ChannelUp2[index] = expo.Result[index15] + (expo.Result[index15] * BandDistance2) / 100; ChannelUp3[index] = expo.Result[index15] + (expo.Result[index15] * BandDistance3) / 100; ChannelUp4[index] = expo.Result[index15] + (expo.Result[index15] * BandDistance4) / 100; ChannelUp5[index] = expo.Result[index15] + (expo.Result[index15] * BandDistance5) / 100; ChannelUp6[index] = expo.Result[index15] + (expo.Result[index15] * BandDistance6) / 100; ChannelLow1[index] = expo.Result[index15] - (expo.Result[index15] * BandDistance1) / 100; ChannelLow2[index] = expo.Result[index15] - (expo.Result[index15] * BandDistance2) / 100; ChannelLow3[index] = expo.Result[index15] - (expo.Result[index15] * BandDistance3) / 100; ChannelLow4[index] = expo.Result[index15] - (expo.Result[index15] * BandDistance4) / 100; ChannelLow5[index] = expo.Result[index15] - (expo.Result[index15] * BandDistance5) / 100; ChannelLow6[index] = expo.Result[index15] - (expo.Result[index15] * BandDistance6) / 100; } if (MAx3Set) { MA1[index] = expo1.Result[index]; MA2[index] = expo2.Result[index]; MA3[index] = expo3.Result[index]; } if (L1) { LineBold = 1; } if (L2) { LineBold = 2; } if (L3) { LineBold = 3; } if (L4) { LineBold = 4; } if (SetRed) { LineColor = Colors.Red; } if (SetWhite) { LineColor = Colors.White; } if (SetBlack) { LineColor = Colors.Black; } if (SetGreen) { LineColor = Colors.YellowGreen; } if (Set100Levels) { for (int i = 1; i < 200; i++) { ChartObjects.DrawHorizontalLine("LineLevel" + i, i * 100 * Symbol.PipSize, LineColor, LineBold); } } } //calculate private int GetIndexByDate(MarketSeries series, DateTime time) { for (int i = series.Close.Count - 1; i > 0; i--) { if (time == series.OpenTime[i]) return i; } return -1; } } }
Have a nice day and bye ...
@breakermind
Spotware
14 Oct 2013, 10:55
You need to get the index that corresponds to the series that is in a different Timeframe. See the example: /forum/whats-new/1463#3
@Spotware