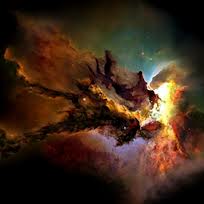
Topics
Replies
atrader
21 Jun 2013, 12:06
There is something similar in this robot: /algos/robots/show/271 Is this the one you are saying it's difficult to reproduce?
I haven't tested this but I think it should be something like this:
protected int getVolume { get { var risk = (int) (RiskPct*Account.Balance/100); var volumeOnRisk = (int) (risk*Symbol.Ask/(Symbol.PipSize*StopLoss)); // or Bid double maxVolume = Account.Equity*Leverage*100/101;
var vol = Math.Min(volumeOnRisk, maxVolume);
return (int)Math.Truncate(Math.Round(vol) / 10000) * 10000; // round to 10K } }
@atrader
atrader
22 May 2013, 17:29
Hi, I posted a new code file. Download it again. The problem were the variables fish1 and value1. I modified it to use IndicatorDataSeries instead of double, because the method is called on each Tick so there are different values for those for the same Bar. Anyway, now it produces the correct results.
@atrader
atrader
23 Apr 2013, 16:24
This should work:
Locate System.Windows.Forms.dll in your system and add a reference to it in cAlgo.
for instance if you have framework 4.0 installed:
//#reference: C:\Program Files (x86)\Reference Assemblies\Microsoft\Framework\.NETFramework\v4.0\System.Windows.Forms.dll using System; using cAlgo.API; using System.Windows.Forms; using System.Threading; namespace cAlgo.Robots { [Robot] public class NewRobot : Robot { protected override void OnStart() { copy_to_clipboard(); } public void somethingToRunInThread() { Clipboard.SetText("this is in clipboard now");
} protected void copy_to_clipboard() { Thread clipboardThread = new Thread(somethingToRunInThread); clipboardThread.SetApartmentState(ApartmentState.STA); clipboardThread.Start(); } } }
@atrader
atrader
19 Apr 2013, 11:50
Hi,
Uploaded here: /algos/robots/show/259
Read the comments, you may need more modifications.
@atrader
atrader
17 Apr 2013, 14:57
Hi Mandril,
I added the code from the cAlgo samples (Sample Buy/Sell Trailing) to your code and uploaded here: /algos/robots/show/257
@atrader
atrader
04 Apr 2013, 15:04
Based on this:
http://www.fxstreet.com/education/learning-center/unit-3/chapter-3/the-position-size/
and
http://www.babypips.com/tools/forex-calculators/positionsize.php
You can use this formula:
var Volume = (((Account.Balance * Risk) / StopLossPips) / PipValue) * 100000;
I believe for xxxUSD where the account currency is USD, the pip value is $10. Not sure how you would calculate this for other currencies.
Risk should be input as a percentage, i.e. 0.01. or if you input 1 then convert it by
Risk = Risk/100; prior to the formula.
StopLossPips should be in pips, i.e. 10 for 10 pips.
@atrader
atrader
11 Jan 2013, 16:24
Something like this maybe.
using System; using cAlgo.API; namespace cAlgo.Robots { [Robot] public class OrdersEveryHour:Robot { [Parameter("Interval", DefaultValue = 1, MaxValue = 12)] public int Interval { get; set; } [Parameter("Volume", DefaultValue = 10000)] public int Volume{get; set;} [Parameter("StopLoss", DefaultValue = 10)] public double StopLoss{get; set;} [Parameter("TakeProfit", DefaultValue = 10)] public double TakeProfit { get; set; } private DateTime _startTime; private int _count; private bool[] _tradedHour; protected override void OnStart() { // Initialize to 00:00:00 of the same day. _startTime = Server.Time.Date; _count = 24 / Interval; _tradedHour = new bool[_count]; } protected override void OnTick() { double sellOrderTargetPrice = Symbol.Bid - Symbol.PipSize; for (int i = 0; i < _count; i++) { if (!_tradedHour[i] && Server.Time.Hour == _startTime.Date.AddHours(i*Interval).Hour) { _tradedHour[i] = true; Print("Trade time {0}", Server.Time); Trade.CreateSellStopOrder(Symbol, Volume, sellOrderTargetPrice, sellOrderTargetPrice + StopLoss*Symbol.PipSize, sellOrderTargetPrice - TakeProfit*Symbol.PipSize); } } } } }
@atrader
atrader
11 Jan 2013, 11:59
Hi phamvanthanh,
I translated your indicator and uploaded it to the indicators library: /algos/show/213
Can you tell us a description of how you use it?
Thanks.
@atrader
atrader
31 Oct 2012, 14:19
Hello,
The object name needs to be unique, otherwise it will be the same object assuming a new position.
Therefore modify the object name for each new instance of text.
For example:
string test = Convert.ToString(MarketSeries.Close[index]);
string objectName = "test" + index.ToString();
ChartObjects.DrawText(objectName, test, index, MarketSeries.High[index], VerticalAlignment.Top, HorizontalAlignment.Center, Colors.White);
@atrader
atrader
24 Jun 2013, 16:53
I'm not sure what you mean. Do you still need help? Post the code you managed to come up with so far and I will try to correct it.
@atrader