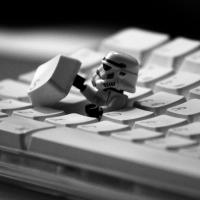
Topics
Replies
mindbreaker
18 Mar 2015, 16:46
RE: RE: RE: RE: RE: RE: RE: RE: RE:
it fixed set all orders from file:
// System using System; using System.IO; using System.Net; using System.Text; using System.Text.RegularExpressions; using System.Collections.Generic; // cAlgo using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class NewcBot : Robot { private string responseFromServer = ""; //private string openPositionsString = ""; [Parameter("Username", DefaultValue = "")] public string Username { get; set; } [Parameter("Password", DefaultValue = "")] public string Password { get; set; } [Parameter("MoneyForSignalUSD", DefaultValue = 100, MinValue = 100)] public int MoneyForSignalUSD { get; set; } List<string> PosOpenID = new List<string>(); List<string> PosCloseID = new List<string>(); List<string> PosServerID = new List<string>(); List<string> PosServerAll = new List<string>(); int multiply = 0; protected override void OnStart() { // Put your initialization logic here } protected override void OnBar() { // Put your initialization logic here } protected override void OnTick() { if (MoneyForSignalUSD >= 100) { multiply = (int)MoneyForSignalUSD / 100; multiply = multiply * 1000; Print("Multiply " + multiply); } // Put your core logic here //sendPositions(Username, Password); // Initialize initializePositions(); getPositions(Username, Password); closeAll(); comparePositions(); } protected override void OnStop() { // Put your deinitialization logic here } protected void closeAll() { string[] posres = responseFromServer.Split('#'); string inp = Convert.ToString(posres[1]); Print("String " + inp); char znak = Convert.ToChar(inp[0]); char znak1 = Convert.ToChar("["); if (znak == znak1) { Print(inp); foreach (var position in Positions) { ClosePosition(position); } } } //==================================================================================================================== // Compare Positions //==================================================================================================================== protected void comparePositions() { try { string[] posres = responseFromServer.Split('#'); string inp = "" + posres[1]; // cut [GO] and [OG] //inp = inp.Substring(4); //inp = inp.Substring(0, inp.Length - 6); // pociapać na pozycję lista string[] posin = inp.Split('|'); PosServerAll = new List<string>(posin); initializePositions(); foreach (string pos in posin) { string[] p = pos.Split(';'); if (!PosOpenID.Contains(p[1]) && p[1] != "" && !PosCloseID.Contains(p[1])) { Symbol symbol = MarketData.GetSymbol(p[3]); // Print(p[3]); if (p[5] == "BUY") { Print("BUY " + p[5]); //p[5].Replace(",", p[5]); double pips1 = double.Parse(p[2], System.Globalization.CultureInfo.InvariantCulture) - double.Parse(p[6], System.Globalization.CultureInfo.InvariantCulture); double sl1 = pips1 / symbol.PipSize; double pips2 = double.Parse(p[7], System.Globalization.CultureInfo.InvariantCulture) - double.Parse(p[2], System.Globalization.CultureInfo.InvariantCulture); double tp1 = pips2 / symbol.PipSize; Print(p[4]); double volume = double.Parse(p[4], System.Globalization.CultureInfo.InvariantCulture) * multiply; volume = volume / 100; volume = 1 * multiply; ; Print(volume); ExecuteMarketOrder(TradeType.Buy, symbol, Convert.ToInt64(volume), "", sl1, tp1, 1, p[1]); //ExecuteMarketOrder(TradeType.Buy, Symbol, Convert.ToInt64(Convert.ToDecimal(p[4])), "Slave", Convert.ToDouble(p[6]), Convert.ToDouble(p[7])); } if (p[5] == "SELL") { Print("SELL " + p[5]); double pips1 = double.Parse(p[6], System.Globalization.CultureInfo.InvariantCulture) - double.Parse(p[2], System.Globalization.CultureInfo.InvariantCulture); double sl = pips1 / symbol.PipSize; double pips2 = double.Parse(p[2], System.Globalization.CultureInfo.InvariantCulture) - double.Parse(p[7], System.Globalization.CultureInfo.InvariantCulture); double tp = pips2 / symbol.PipSize; double volume = double.Parse(p[4], System.Globalization.CultureInfo.InvariantCulture) * multiply; volume = volume / 100; volume = 1 * multiply; ExecuteMarketOrder(TradeType.Sell, symbol, Convert.ToInt64(volume), "", sl, tp, 1, p[1]); //ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, MyLabel, StopLoss, TakeProfit); } } initializePositions(); } } catch (Exception rrr) { Print(rrr); } } //==================================================================================================================== // Initialize Positions //==================================================================================================================== protected void initializePositions() { // open id PosOpenID.Clear(); var AllPositions = Positions.FindAll(""); foreach (var position in AllPositions) { if (position.Comment != "") { PosOpenID.Add(position.Comment); } } // colose id PosCloseID.Clear(); foreach (HistoricalTrade trade in History) { if (trade.Comment != "") { PosCloseID.Add(trade.Comment); } } // Loop through List with foreach //Print("OPEN POS ID COMMENT "); foreach (string prime in PosOpenID) { // Print(prime); } // Print("CLOSED POS ID COMMENT "); // Loop through List with foreach foreach (string prime in PosCloseID) { // Print(prime); } } //==================================================================================================================== // Send POST to HTTPS Server //==================================================================================================================== protected void getPositions(string Username, string Password) { // log file path string desktopFolder = Environment.GetFolderPath(Environment.SpecialFolder.DesktopDirectory); //string logPath = Path.Combine(desktopFolder, "MasterLog.db"); //================================================================================ // Send request //================================================================================ try { /* using (StreamWriter w = File.AppendText(logPath)) { // log request w.WriteLine("REQUEST: " + DateTimeToUnixTimestamp(DateTime.UtcNow) + " : " + openPositionsString); w.Flush(); w.Close(); } */ WebRequest request = WebRequest.Create("https://fx-breakermind.rhcloud.com/orders/all.txt"); Print("===================================================================================="); byte[] postBytes = Encoding.ASCII.GetBytes("user=" + Username + "&pass=" + Password); request.Proxy = null; request.Method = "POST"; request.ContentType = "application/x-www-form-urlencoded"; request.ContentLength = postBytes.Length; Stream requestStream = request.GetRequestStream(); requestStream.Write(postBytes, 0, postBytes.Length); HttpWebResponse response = (HttpWebResponse)request.GetResponse(); Stream dataStream = response.GetResponseStream(); StreamReader reader = new StreamReader(dataStream); responseFromServer = reader.ReadToEnd(); } catch (Exception e) { Print("===================================================================================="); Print("Post Error: " + e); Print("===================================================================================="); /* using (StreamWriter w = File.AppendText(logPath)) { // log response w.WriteLine("ERROR: " + DateTimeToUnixTimestamp(DateTime.UtcNow) + " : " + e); w.Flush(); w.Close(); } */ } Print("===================================================================================="); Print("<<== " + responseFromServer); Print("===================================================================================="); } /* using (StreamWriter w = File.AppendText(logPath)) { // log response w.WriteLine("RESPONSE: " + DateTimeToUnixTimestamp(DateTime.UtcNow) + " : " + responseFromServer); w.Flush(); w.Close(); } */ /// datatime to timestamp public static double DateTimeToUnixTimestamp(DateTime dateTime) { return (dateTime - new DateTime(1970, 1, 1).ToLocalTime()).TotalSeconds; } // end //================================================================================================================ // End Send POST to HTTPS Server //================================================================================================================ } } //==================================================================================================================== // Initialize Positions //====================================================================================================================
Bye.
@mindbreaker
mindbreaker
18 Mar 2015, 16:27
RE: RE: RE: RE: RE: RE: RE: RE:
this work but set only first position:
// System using System; using System.IO; using System.Net; using System.Text; using System.Text.RegularExpressions; using System.Collections.Generic; // cAlgo using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class NewcBot : Robot { private string responseFromServer = ""; //private string openPositionsString = ""; [Parameter("Username", DefaultValue = "")] public string Username { get; set; } [Parameter("Password", DefaultValue = "")] public string Password { get; set; } [Parameter("MoneyForSignalUSD", DefaultValue = 100, MinValue = 100)] public int MoneyForSignalUSD { get; set; } List<string> PosOpenID = new List<string>(); List<string> PosCloseID = new List<string>(); List<string> PosServerID = new List<string>(); List<string> PosServerAll = new List<string>(); int multiply = 0; protected override void OnStart() { // Put your initialization logic here } protected override void OnBar() { // Put your initialization logic here } protected override void OnTick() { if (MoneyForSignalUSD >= 100) { multiply = (int)MoneyForSignalUSD / 100; multiply = multiply * 100000; Print("Multiply " + multiply); } // Put your core logic here //sendPositions(Username, Password); // Initialize initializePositions(); getPositions(Username, Password); closeAll(); comparePositions(); } protected override void OnStop() { // Put your deinitialization logic here } protected void closeAll() { string[] posres = responseFromServer.Split('#'); string inp = Convert.ToString(posres[1]); Print("String " + inp); char znak = Convert.ToChar(inp[0]); char znak1 = Convert.ToChar("["); if (znak == znak1) { Print(inp); foreach (var position in Positions) { ClosePosition(position); } } } //==================================================================================================================== // Compare Positions //==================================================================================================================== protected void comparePositions() { try { string[] posres = responseFromServer.Split('#'); string inp = "" + posres[1]; // cut [GO] and [OG] //inp = inp.Substring(4); //inp = inp.Substring(0, inp.Length - 6); // pociapać na pozycję lista string[] posin = inp.Split('|'); PosServerAll = new List<string>(posin); initializePositions(); string[] p = posin[0].Split(';'); if (!PosOpenID.Contains(p[1]) && p[1] != "" && !PosCloseID.Contains(p[1])) { Symbol symbol = MarketData.GetSymbol(p[3]); // Print(p[3]); if (p[5] == "BUY") { Print("BUY " + p[5]); //p[5].Replace(",", p[5]); double pips1 = double.Parse(p[2], System.Globalization.CultureInfo.InvariantCulture) - double.Parse(p[6], System.Globalization.CultureInfo.InvariantCulture); double sl1 = pips1 / symbol.PipSize; double pips2 = double.Parse(p[7], System.Globalization.CultureInfo.InvariantCulture) - double.Parse(p[2], System.Globalization.CultureInfo.InvariantCulture); double tp1 = pips2 / symbol.PipSize; Print(p[4]); double volume = double.Parse(p[4], System.Globalization.CultureInfo.InvariantCulture) * multiply; volume = volume / 100; Print(volume); ExecuteMarketOrder(TradeType.Buy, symbol, Convert.ToInt64(volume), "", sl1, tp1, 1, p[1]); //ExecuteMarketOrder(TradeType.Buy, Symbol, Convert.ToInt64(Convert.ToDecimal(p[4])), "Slave", Convert.ToDouble(p[6]), Convert.ToDouble(p[7])); } if (p[5] == "SELL") { Print("SELL " + p[5]); double pips1 = double.Parse(p[6], System.Globalization.CultureInfo.InvariantCulture) - double.Parse(p[2], System.Globalization.CultureInfo.InvariantCulture); double sl = pips1 / symbol.PipSize; double pips2 = double.Parse(p[2], System.Globalization.CultureInfo.InvariantCulture) - double.Parse(p[7], System.Globalization.CultureInfo.InvariantCulture); double tp = pips2 / symbol.PipSize; double volume = double.Parse(p[4], System.Globalization.CultureInfo.InvariantCulture) * multiply; volume = volume / 100; ExecuteMarketOrder(TradeType.Sell, symbol, Convert.ToInt64(volume), "", sl, tp, 1, p[1]); //ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, MyLabel, StopLoss, TakeProfit); } } initializePositions(); } catch (Exception rrr) { Print(rrr); } } //==================================================================================================================== // Initialize Positions //==================================================================================================================== protected void initializePositions() { // open id PosOpenID.Clear(); var AllPositions = Positions.FindAll(""); foreach (var position in AllPositions) { if (position.Comment != "") { PosOpenID.Add(position.Comment); } } // colose id PosCloseID.Clear(); foreach (HistoricalTrade trade in History) { if (trade.Comment != "") { PosCloseID.Add(trade.Comment); } } // Loop through List with foreach //Print("OPEN POS ID COMMENT "); foreach (string prime in PosOpenID) { // Print(prime); } // Print("CLOSED POS ID COMMENT "); // Loop through List with foreach foreach (string prime in PosCloseID) { // Print(prime); } } //==================================================================================================================== // Send POST to HTTPS Server //==================================================================================================================== protected void getPositions(string Username, string Password) { // log file path string desktopFolder = Environment.GetFolderPath(Environment.SpecialFolder.DesktopDirectory); //string logPath = Path.Combine(desktopFolder, "MasterLog.db"); //================================================================================ // Send request //================================================================================ try { /* using (StreamWriter w = File.AppendText(logPath)) { // log request w.WriteLine("REQUEST: " + DateTimeToUnixTimestamp(DateTime.UtcNow) + " : " + openPositionsString); w.Flush(); w.Close(); } */ WebRequest request = WebRequest.Create("https://fx-breakermind.rhcloud.com/orders/all.txt"); Print("===================================================================================="); byte[] postBytes = Encoding.ASCII.GetBytes("user=" + Username + "&pass=" + Password); request.Proxy = null; request.Method = "POST"; request.ContentType = "application/x-www-form-urlencoded"; request.ContentLength = postBytes.Length; Stream requestStream = request.GetRequestStream(); requestStream.Write(postBytes, 0, postBytes.Length); HttpWebResponse response = (HttpWebResponse)request.GetResponse(); Stream dataStream = response.GetResponseStream(); StreamReader reader = new StreamReader(dataStream); responseFromServer = reader.ReadToEnd(); } catch (Exception e) { Print("===================================================================================="); Print("Post Error: " + e); Print("===================================================================================="); /* using (StreamWriter w = File.AppendText(logPath)) { // log response w.WriteLine("ERROR: " + DateTimeToUnixTimestamp(DateTime.UtcNow) + " : " + e); w.Flush(); w.Close(); } */ } Print("===================================================================================="); Print("<<== " + responseFromServer); Print("===================================================================================="); } /* using (StreamWriter w = File.AppendText(logPath)) { // log response w.WriteLine("RESPONSE: " + DateTimeToUnixTimestamp(DateTime.UtcNow) + " : " + responseFromServer); w.Flush(); w.Close(); } */ /// datatime to timestamp public static double DateTimeToUnixTimestamp(DateTime dateTime) { return (dateTime - new DateTime(1970, 1, 1).ToLocalTime()).TotalSeconds; } // end //================================================================================================================ // End Send POST to HTTPS Server //================================================================================================================ } } //==================================================================================================================== // Initialize Positions //====================================================================================================================
why?
@mindbreaker
mindbreaker
18 Mar 2015, 16:20
RE: RE: RE: RE: RE: RE: RE:
This FxPro.com cAlgo shows different errors each day on this same cBot (poor).
@mindbreaker
mindbreaker
18 Mar 2015, 16:11
RE: RE: RE: RE: RE: RE:
and this error
18/03/2015 14:05:48.544 | System.ArgumentOutOfRangeException: Index and length must refer to a location within the string. Parameter name: length at System.String.InternalSubStringWithChecks(Int32 startIndex, Int32 length, Boolean fAlwaysCopy) at cAlgo.NewcBot.comparePositions()
@mindbreaker
mindbreaker
18 Mar 2015, 16:10
RE: RE: RE: RE: RE:
and how open positions only from one user?
@mindbreaker
mindbreaker
18 Mar 2015, 16:08
RE: RE: RE: RE:
Hi,
this is copy bot
// System using System; using System.IO; using System.Net; using System.Text; using System.Text.RegularExpressions; using System.Collections.Generic; // cAlgo using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class NewcBot : Robot { private string responseFromServer = ""; //private string openPositionsString = ""; [Parameter("Username", DefaultValue = "")] public string Username { get; set; } [Parameter("Password", DefaultValue = "")] public string Password { get; set; } [Parameter("MoneyForSignalUSD", DefaultValue = 100, MinValue = 100)] public int MoneyForSignalUSD { get; set; } List<string> PosOpenID = new List<string>(); List<string> PosCloseID = new List<string>(); List<string> PosServerID = new List<string>(); List<string> PosServerAll = new List<string>(); int multiply = 0; protected override void OnStart() { // Put your initialization logic here } protected override void OnBar() { // Put your initialization logic here } protected override void OnTick() { if (MoneyForSignalUSD >= 100) { multiply = (int)MoneyForSignalUSD / 100; multiply = multiply * 100000; Print("Multiply " + multiply); } // Put your core logic here //sendPositions(Username, Password); // Initialize initializePositions(); getPositions(Username, Password); closeAll(); comparePositions(); } protected override void OnStop() { // Put your deinitialization logic here } protected void closeAll() { string[] posres = responseFromServer.Split('#'); string inp = Convert.ToString(posres[1]); Print("String " + inp); char znak = Convert.ToChar(inp[0]); char znak1 = Convert.ToChar("["); if (znak == znak1) { Print(inp); foreach (var position in Positions) { ClosePosition(position); } } } //==================================================================================================================== // Compare Positions //==================================================================================================================== protected void comparePositions() { try { string[] posres = responseFromServer.Split('#'); string inp = "" + posres[1]; // cut [GO] and [OG] //inp = inp.Substring(4); //inp = inp.Substring(0, inp.Length - 6); // pociapać na pozycję lista string[] posin = inp.Split('|'); PosServerAll = new List<string>(posin); initializePositions(); string[] p = posin[0].Split(';'); if (!PosOpenID.Contains(p[1]) && p[1] != "" && !PosCloseID.Contains(p[1])) { Symbol symbol = MarketData.GetSymbol(p[3]); // Print(p[3]); if (p[5] == "BUY") { Print("BUY " + p[5]); //p[5].Replace(",", p[5]); double pips1 = double.Parse(p[2], System.Globalization.CultureInfo.InvariantCulture) - double.Parse(p[6], System.Globalization.CultureInfo.InvariantCulture); double sl1 = pips1 / symbol.PipSize; double pips2 = double.Parse(p[7], System.Globalization.CultureInfo.InvariantCulture) - double.Parse(p[2], System.Globalization.CultureInfo.InvariantCulture); double tp1 = pips2 / symbol.PipSize; Print(p[4]); double volume = double.Parse(p[4].Substring(0, 4), System.Globalization.CultureInfo.InvariantCulture) * multiply; Print(volume); ExecuteMarketOrder(TradeType.Buy, symbol, Convert.ToInt64(volume), "", sl1, tp1, 1, p[1]); //ExecuteMarketOrder(TradeType.Buy, Symbol, Convert.ToInt64(Convert.ToDecimal(p[4])), "Slave", Convert.ToDouble(p[6]), Convert.ToDouble(p[7])); } if (p[5] == "SELL") { Print("SELL " + p[5]); double pips1 = double.Parse(p[6], System.Globalization.CultureInfo.InvariantCulture) - double.Parse(p[2], System.Globalization.CultureInfo.InvariantCulture); double sl = pips1 / symbol.PipSize; double pips2 = double.Parse(p[2], System.Globalization.CultureInfo.InvariantCulture) - double.Parse(p[7], System.Globalization.CultureInfo.InvariantCulture); double tp = pips2 / symbol.PipSize; double volume = double.Parse(p[4].Substring(0, 4), System.Globalization.CultureInfo.InvariantCulture) * multiply; ExecuteMarketOrder(TradeType.Sell, symbol, Convert.ToInt64(volume), "", sl, tp, 1, p[1]); //ExecuteMarketOrder(TradeType.Sell, Symbol, Volume, MyLabel, StopLoss, TakeProfit); } } initializePositions(); } catch (Exception rrr) { Print(rrr); } } //==================================================================================================================== // Initialize Positions //==================================================================================================================== protected void initializePositions() { // open id PosOpenID.Clear(); var AllPositions = Positions.FindAll(""); foreach (var position in AllPositions) { if (position.Comment != "") { PosOpenID.Add(position.Comment); } } // colose id PosCloseID.Clear(); foreach (HistoricalTrade trade in History) { if (trade.Comment != "") { PosCloseID.Add(trade.Comment); } } // Loop through List with foreach //Print("OPEN POS ID COMMENT "); foreach (string prime in PosOpenID) { // Print(prime); } // Print("CLOSED POS ID COMMENT "); // Loop through List with foreach foreach (string prime in PosCloseID) { // Print(prime); } } //==================================================================================================================== // Send POST to HTTPS Server //==================================================================================================================== protected void getPositions(string Username, string Password) { // log file path string desktopFolder = Environment.GetFolderPath(Environment.SpecialFolder.DesktopDirectory); //string logPath = Path.Combine(desktopFolder, "MasterLog.db"); //================================================================================ // Send request //================================================================================ try { /* using (StreamWriter w = File.AppendText(logPath)) { // log request w.WriteLine("REQUEST: " + DateTimeToUnixTimestamp(DateTime.UtcNow) + " : " + openPositionsString); w.Flush(); w.Close(); } */ WebRequest request = WebRequest.Create("https://fx-breakermind.rhcloud.com/orders/all.txt"); Print("===================================================================================="); byte[] postBytes = Encoding.ASCII.GetBytes("user=" + Username + "&pass=" + Password); request.Proxy = null; request.Method = "POST"; request.ContentType = "application/x-www-form-urlencoded"; request.ContentLength = postBytes.Length; Stream requestStream = request.GetRequestStream(); requestStream.Write(postBytes, 0, postBytes.Length); HttpWebResponse response = (HttpWebResponse)request.GetResponse(); Stream dataStream = response.GetResponseStream(); StreamReader reader = new StreamReader(dataStream); responseFromServer = reader.ReadToEnd(); } catch (Exception e) { Print("===================================================================================="); Print("Post Error: " + e); Print("===================================================================================="); /* using (StreamWriter w = File.AppendText(logPath)) { // log response w.WriteLine("ERROR: " + DateTimeToUnixTimestamp(DateTime.UtcNow) + " : " + e); w.Flush(); w.Close(); } */ } Print("===================================================================================="); Print("<<== " + responseFromServer); Print("===================================================================================="); } /* using (StreamWriter w = File.AppendText(logPath)) { // log response w.WriteLine("RESPONSE: " + DateTimeToUnixTimestamp(DateTime.UtcNow) + " : " + responseFromServer); w.Flush(); w.Close(); } */ /// datatime to timestamp public static double DateTimeToUnixTimestamp(DateTime dateTime) { return (dateTime - new DateTime(1970, 1, 1).ToLocalTime()).TotalSeconds; } // end //================================================================================================================ // End Send POST to HTTPS Server //================================================================================================================ } } //==================================================================================================================== // Initialize Positions //====================================================================================================================
but have some error:
18/03/2015 14:05:48.887 | Crashed in OnTick with IndexOutOfRangeException: Index was outside the bounds of the array.
Maybe someone know how fix it
@mindbreaker
mindbreaker
18 Mar 2015, 15:53
RE: RE: RE:
Position string (|):
time;id;openprice;symbol;volume;type;sl;tp;ProfitPips;Signal:UserName
1426626282000;79831685;0.712248;EURGBP;5.0;SELL;0.7275;0.70879;-107.7;Signal:UserName
https://www.dukascopy.com/tradercontest/?action=blog&trader=UserName
Positions from demo account.
@mindbreaker
mindbreaker
18 Mar 2015, 15:46
RE: RE:
Hi
link:
https://fx-breakermind.rhcloud.com/orders/all.php
Account initial month deposit for user is 100 000 USD each month position volume from 1 to 5 millions for each currency (symbol) max 1 position on Symbol. Leve 1:100.
if you want copy from 100USD then Volume / 100
@mindbreaker
mindbreaker
18 Mar 2015, 15:00
RE:
modarkat said:
Are you sure that you pass correct string? Try "1.23" and "1,23"
Thanks
but it dont works to,
i need parse string first, now works but some time before this works on diferent robot (ble calgo).
There is contest positions from dukascopy onTick for copy positions to mt4 or calgo robots (Only 11 users added, but i can add more :] hihihii ).
https://fx-breakermind.rhcloud.com/orders/all.txt
Byeeeeeeeeeeeeeeeeeeeeee.
@mindbreaker
mindbreaker
17 Mar 2015, 14:23
cAlgo cant convert string to double
17/03/2015 11:41:18.823 | System.FormatException: Input string was not in a correct format. at System.Number.ParseDouble(String value, NumberStyles options, NumberFormatInfo numfmt) at System.Convert.ToDouble(String value) at cAlgo.NewcBot.comparePositions() 17/03/2015 12:03:43.547 | System.FormatException: Input string was not in a correct format. at System.Number.StringToNumber(String str, NumberStyles options, NumberBuffer& number, NumberFormatInfo info, Boolean parseDecimal) at System.Number.ParseDecimal(String value, NumberStyles options, NumberFormatInfo numfmt) at System.Convert.ToDecimal(String value) at cAlgo.NewcBot.comparePositions()
@mindbreaker
mindbreaker
08 Mar 2015, 15:43
Hello,
change this ) /api/reference/accessrights (
AccessRights = AccessRights.None
AccessRights.Internet
or
AccessRights.FullAccess
probably.
@mindbreaker
mindbreaker
14 Feb 2015, 21:10
RE:
Fritz125 said:
I would still like some help to finish my bot though
I would write, but I did not write too many indicatoros. I transformed the existing.
I threw cAlgo and cTrader from my computer - something not work forever, and could not be regression tested to backtest,
I do not use anymore cAlgo.
Bye.
@mindbreaker
mindbreaker
14 Feb 2015, 20:55
( Updated at: 21 Dec 2023, 09:20 )
Hi hi hi fx
Fritz125 said:
Well i have regression installed but i don't know how to trade with it or when does it gives signals. Any help?
Regression it looks like this in my mt4
(on bold blue line buy ---- on red bold line sell)
my settings on mt4 [3, 2 , 2000] ,
cTrader/cAlgo [RegDegree 3, RegStrdDev 2, RegPeriod 2000]
M1 period for (day trading):
M5 and M15 and H1 for long time
M15
image: ma50(level up dn 50pips), ma200, ma1300(level up/dn 150Pips black), 100Pips levels(blue line) support/resistance(red line), MACD(50,200,19), double dashed lines week start
my demo signals on mt4:
https://www.mql5.com/en/users/woow
on
www.cmirror.com signal mindbreaker but dont work this page with (ble....).
Bye ;)
@mindbreaker
mindbreaker
14 Feb 2015, 17:38
Hello forex
Hey,
You will learn faster than anything you get here :D:D:D:D I know this from my experience.
Do not waste your time -> the indicators here goes only to the top or to the bottom from weekOpenPrice or monthOpenPrice line.
Deposit 100USD position Lot(volume) 0.01(1000) - 100Pips pending on each level from weekOpenPrice [123,000 124,000 125,000 like GBPJPY] 100Pips ---> 10% - praktycznie w żadnym miesiącu się nie wyzerujesz grając tylko jedną pozycją do góry lub do dołu :).
And earn !!!!
Only brokers want you to think that these indicators in something to help!
When you longer hold the position then more you earn.
Often does not mean better (in this way 100% in month is not a problem).
but i dont know better indicator than ---------------> Regression :]
100% per month x 12 periods = > milions
Bye.
@mindbreaker
mindbreaker
04 Feb 2015, 11:53
cBots - Indicators
Hi,
cBots and Indicators(100PipsEnvelope, Regression2000, FireBot ... ):
https://github.com/breakermind/cAlgoRobotsIndicators
Bye
@mindbreaker
mindbreaker
04 Feb 2015, 10:32
RE:
kricka said:
Hi guys,
this is a community for cAlgo, cTrader, cBots and Spotware and programmers bringing out their creations for just that.
Keep your concentration on building robots and code only for the benefits of this community, please!
Hi,
it was private message :) why you eavesdropping on ;) :D
Spotware Please create a possibility of sending private messages.
Bye
Ps. Kricka dont need to have broad interests, can not be limited ;)
@mindbreaker
mindbreaker
03 Feb 2015, 09:29
RE:
maninjapan said:
Thanks mindbreaker. Much appreciated What do I do with this SocketServerMultiple.cs file though?
Also I have just tried to open the Provider file in CAlgo but says that Source code is not available, am I doing something wrong here?
Hi,
it's txt files (create new cbot in cAlgo and copy and past cbot source [How to copy:: Ctrl + A (Select all), Ctrl + C (Copy selected) Ctrl + V (Past text)]) and then compile in cAlgo.
SocketServerMultiple.cs You need compile this file in c# (download visual studio its free - create new c# console project in Visual Studio 2010 or high) past this code and hit green arrow on top of the screen.
It compile and run Server on localhost ip 127.0.0.1
and create .exe file in Users/Documents/visual studio/.... projects folder/projectName/bin ....
You need start this exe when you copy positions (or compile from VisualStudio each time)
cAlgoProvider send positions to this Server and then cAlgoClients can read this posions.
Bye
@mindbreaker
mindbreaker
02 Feb 2015, 12:33
cAlgoCopier
maninjapan said:
Mindbreaker, I would be interested in your ready made bot. Can you provide information on how I could get a copy?
Hi Maninjapan,
Here(Try this):
https://github.com/breakermind/cAlgoCopier
Bye
@mindbreaker
mindbreaker
18 Mar 2015, 16:53
RE: RE: RE: RE: RE: RE: RE: RE: RE: RE:
@mindbreaker