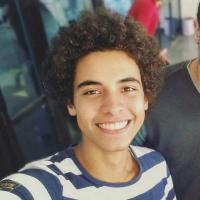
Topics
Replies
abdulhamid0yusuf
07 Apr 2020, 16:05
Simple and robust trailing algorithm
I came here to see how to implement a trailing stop loss, but the code provided by cTrader Team was unnecessarily complicated to me, so I would like to share my way ...
using cAlgo.API;
using System.Collections.Generic;
using System.Linq;
namespace cAlgo.Robots
{
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class TestTrailingStop : Robot
{
// Save the maximum winning pips each position has reached
private Dictionary<Position, double> PositionsMaxProfits;
[Parameter("Winning Pips to protect", DefaultValue = 10)]
public int PipsToProtect { get; set; }
[Parameter("Trailing Stop Distance", DefaultValue = 5)]
public int TrailingDistance { get; set; }
protected override void OnStart()
{
PositionsMaxProfits = new Dictionary<Position, double>();
// Market order for illustration purposes
var p = ExecuteMarketOrder(TradeType.Sell, SymbolName, 1000).Position;
// Add every order you create to the dictionary and set its initial max pips to 0
PositionsMaxProfits.Add(p, 0);
}
protected override void OnTick()
{
// Update Stop loss on each tick once the position has yeilded winning pips >= PipsToProtect + TrailingDistance
foreach (Position position in PositionsMaxProfits.Keys.ToList())
{
var positionMaxProfit = PositionsMaxProfits[position];
// 1st condition checks if winning pips >= PipsToProtect + TrailingDistance
// 2ed condition checks if winning pips is greater than previous winning pips
if ((position.Pips >= PipsToProtect + TrailingDistance) && position.Pips > positionMaxProfit)
{
// Assign the new higher winning pips to the position
PositionsMaxProfits[position] = position.Pips;
// Modify Stop loss to be $TrailingDistance pips away from current winnig pips
// ** -1 means put stop loss on oppostie direction than normal
position.ModifyStopLossPips(-1 * (position.Pips - TrailingDistance));
}
}
}
}
}
@abdulhamid0yusuf
abdulhamid0yusuf
23 Mar 2020, 02:41
RE: [Solved]
abdulhamid0yusuf said:
I'm getting this error when Instantiating a Selenium ChromeDriver Object.
The Exception is thrown from the last line in the code.
var driverService = ChromeDriverService.CreateDefaultService(); driverService.HideCommandPromptWindow = true; var options = new ChromeOptions(); options.AddUserProfilePreference("profile.managed_default_content_settings.images", 2); options.AddArguments("--headless", "disable-gpu"); _Session = new ChromeDriver(driverService, options);
The robot has the full rights to access resources
[Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)]
I Had to copy all .dll files and the chrome driver.exe in the project to the execution path of the robot, This is really overwhelming and unacceptable, there should be a platform independent solution.
@abdulhamid0yusuf
abdulhamid0yusuf
10 Apr 2020, 00:20
RE: RE: Simple and robust trailing algorithm
jani said:
Hey jani, Yes I have tested this code and it works as expected, but in order to add a step to. modify the trailing stop every time a new level is reached, you will need to modify the conditional statement to be as follows:
Let me know if this is what you want,
Thanks.
@abdulhamid0yusuf