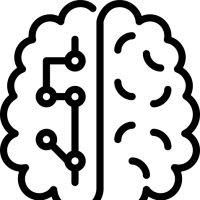
Topics
Replies
paolo.panicali
28 Jul 2022, 14:53
( Updated at: 21 Dec 2023, 09:22 )
Maybe you want an indicator, more reusable and with some minor changes can work ok every timeframe perfectly
Hi, I made an indicator for this because trading the australian session from 21 to 6 central european time the < and > caused me troubles.
Just add a reference to your bot and if Hourok.Result.LastValue is 1 you can place your trade.
If you want to use it on timeframes < 1 hour you will have to do some minor changes adding minutes filtering, if you want to use it for timeframes >1 hour you have to decode bar time to and index(1 to 6 for 4hours timeframe) otherwise you will be missing lot of bars( 4h bars starting time changes over time....).
Ctrader , Indicator and bot on the same timezone, this is central european. Remember that if the timezone has a daylight saving time in order to show correctly the time you have to change ctrader time(we are on summer and you wanna check the indicator on winter you will have to change ctrader time)
// TIME TO TRADE INDICATOR by Paolo Panicali 2022
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.CentralEuropeStandardTime, AccessRights = AccessRights.None)]
public class Hourok : Indicator
{
[Parameter("Start Hour", Group = "Time To Trade", DefaultValue = 0, MinValue = 0, MaxValue = 23)]
public int StartHour { get; set; }
[Parameter("End Hour", Group = "Time To Trade", DefaultValue = 23, MinValue = 0, MaxValue = 23)]
public int EndHour { get; set; }
[Output("Main")]
public IndicatorDataSeries Result { get; set; }
DateTime StartTime, EndTime;
bool HourOk;
public override void Calculate(int index)
{
DateTime DT, DC;
DC = Bars.OpenTimes[index];
HourOk = false;
//IF START AND END HOUR ARE EQUAL THE RESULT IS ALWAYS TRUE
if (StartHour == DC.Hour || EndHour == DC.Hour || StartHour == EndHour)
{
HourOk = true;
}
else
{
for (int i = 0; i <= 24; i++)
{
DT = DC.AddHours(-i);
if (DT.Hour == EndHour)
{
break;
}
if (DT.Hour == StartHour)
{
StartTime = DT;
break;
}
}
for (int i = 0; i <= 24; i++)
{
DT = DC.AddHours(i);
if (DT.Hour == StartHour)
{
break;
}
if (DT.Hour == EndHour)
{
EndTime = DT;
break;
}
}
HourOk = Bars.OpenTimes[index] >= StartTime && Bars.OpenTimes[index] <= EndTime ? true : false;
}
Result[index] = HourOk == true ? 1 : 0;
}
}
}
Hope it will help, before using it on real trading check the code carefully.
Bye.
@paolo.panicali
paolo.panicali
28 Jul 2022, 14:07
I managed to understand what you wanted to achieve, here is the code
Hi, several errors. Z was set as an integer while it is a double, when you divide it by 100 it goes to 0 if set as integer, while as double you get a real value.
Bar lastvalue is literally the last value, for an indicator you have to use bars.closeprices[index] for the current bar over time.
There are some LastValue left over you can correct them, the indicator works now.
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class RetracementBar : Indicator
{
[Parameter("Inventory Retracement Percentage %", DefaultValue = 45)]
public double z { get; set; }
[Output("Main")]
public IndicatorDataSeries Result { get; set; }
public override void Calculate(int index)
{
// Candle Range
double a = Math.Abs(Bars.HighPrices[index] - Bars.LowPrices[index]);
// Candle Body
double b = Math.Abs(Bars.ClosePrices[index] - Bars.OpenPrices[index]);
// Percent to Decimal
double c = z / 100;
var rv = def_rv(a, b, c);
var x = Bars.LowPrices[index] + (c * a);
var y = Bars.HighPrices[index] - (c * a);
var sl = def_sl(index, rv, y);
var ss = def_ss(rv, x);
var li = def_li(sl, y, ss, x);
Result[index] = li;
if (sl)
{
Chart.DrawIcon(Bars.OpenTimes.LastValue.ToString(), ChartIconType.DownArrow, Bars.OpenTimes.LastValue, Bars.HighPrices.LastValue, Color.Blue);
Print("sl");
}
if (ss)
{
Chart.DrawIcon(Bars.OpenTimes.LastValue.ToString(), ChartIconType.UpArrow, Bars.OpenTimes.LastValue, Bars.LowPrices.LastValue, Color.Red);
Print("ss");
}
}
public bool def_rv(double a, double b, double c)
{
// Print(a + " " + b + " " + c);
if (b < c * a)
{
return true;
}
else
{
return false;
}
}
public bool def_sl(int index, bool rv, double y)
{
if (rv)
{
//Print("rv true");
// Print("rv " + rv + " Y value passed: " + y + " high: " + Bars.HighPrices[index] + " open: " + Bars.OpenPrices[index] + " close: " + Bars.ClosePrices[index]);
}
//y = y - 1E-05;
if (rv && Bars.HighPrices[index] > y && Bars.ClosePrices[index] < y && Bars.OpenPrices[index] < y)
{
//Print("rv " + rv + " Y value passed: " + y + " high: " + Bars.HighPrices[index] + " open: " + Bars.OpenPrices[index] + " close: " + Bars.ClosePrices[index]);
return true;
}
else
{
//Print("rv " + rv + " Y value passed: " + y + " high: " + Bars.HighPrices[index] + " open: " + Bars.OpenPrices[index] + " close: " + Bars.ClosePrices[index]);
return false;
}
}
public bool def_ss(bool rv, double x)
{
if (rv && Bars.LowPrices.LastValue < x && Bars.ClosePrices.LastValue > x && Bars.OpenPrices.LastValue > x)
{
return true;
}
else
{
return false;
}
}
public double def_li(bool sl, double y, bool ss, double x)
{
if (sl)
{
return y;
}
if (ss && !sl)
{
return x;
}
else
{
return ((x + y) / 2);
}
}
}
}
REMEMBER TO REPLACE LASTVALUE WITH [INDEX] OTHERWISE YOU GET THE VALUES OF THE LAST BAR ALWAYS
Bye.
@paolo.panicali
paolo.panicali
28 Jul 2022, 13:42
( Updated at: 21 Dec 2023, 09:22 )
Rv is always false and Y is equal to HighPrices
If you debug the old fashioned way adding this:
....................
public bool def_sl(bool rv, double y)
{
if (rv)
{
Print("rv " + rv + " Y value passed: " + y + " high: " + Bars.HighPrices.LastValue + " open: " + Bars.OpenPrices.LastValue + " close: " + Bars.ClosePrices.LastValue);
}
.................
you realize that rv is never true.
While if you add a not condition to rv:
....................
public bool def_sl(bool rv, double y)
{
if (!rv)
{
Print("rv " + rv + " Y value passed: " + y + " high: " + Bars.HighPrices.LastValue + " open: " + Bars.OpenPrices.LastValue + " close: " + Bars.ClosePrices.LastValue);
}
.................
and you realize that Y is always equal to HighPrices so that the second condition is never True neither
if fact if you manually subtract a minimal value to Y and removing rv true condition, adding the following code, you get an arrow on every bar.
public bool def_sl(bool rv, double y)
{
if (!rv)
{
Print("rv " + rv + " Y value passed: " + y + " high: " + Bars.HighPrices.LastValue + " open: " + Bars.OpenPrices.LastValue + " close: " + Bars.ClosePrices.LastValue);
}
y = y - 1E-05;
if (Bars.HighPrices.LastValue > y && Bars.ClosePrices.LastValue < y && Bars.OpenPrices.LastValue < y)
{
return true;
}
else...
So the problem is the rv condition and the y value, I do not understand what is the idea of the indicator so that cannot provide you a solution.
A fatherly advice, do not use alphabet letters to name variables.
@paolo.panicali
paolo.panicali
28 Jul 2022, 13:06
For the Minute separator on a tick chart I answered to you on the other thread
@paolo.panicali
paolo.panicali
28 Jul 2022, 13:04
Minute bar separator on tick chart
//Answer #2 to MongolTrader since: 12 Feb 2015 28 Jul 2022, 09:53
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class MongolTrader2 : Indicator
{
public override void Calculate(int index)
{
var srcPrev = Bars.OpenTimes[index - 1];
var src = Bars.OpenTimes[index];
if (srcPrev.Minute == src.AddMinutes(-1).Minute)
{
var line1 = Chart.DrawVerticalLine("Line" + index, src.AddMinutes(-1), Color.Red, 2, LineStyle.DotsRare);
}
}
}
}
@paolo.panicali
paolo.panicali
28 Jul 2022, 12:51
Try this code, it works for me with timeframe set to 1 minute it updates every minute....
//Answer to MongolTrader since: 12 Feb 2015 28 Jul 2022, 09:53
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class MongolTrader : Indicator
{
public override void Calculate(int index)
{
var src = Bars.OpenTimes[index];
var line1 = Chart.DrawVerticalLine("1", src.AddMinutes(-1), Color.Red, 2, LineStyle.DotsRare);
var line2 = Chart.DrawVerticalLine("2", src.AddMinutes(-2), Color.Blue, 2, LineStyle.DotsRare);
var line3 = Chart.DrawVerticalLine("3", src.AddMinutes(-3), Color.Blue, 2, LineStyle.DotsRare);
var line4 = Chart.DrawVerticalLine("4", src.AddMinutes(-4), Color.Blue, 2, LineStyle.DotsRare);
var line5 = Chart.DrawVerticalLine("5", src.AddMinutes(-5), Color.Blue, 2, LineStyle.DotsRare);
var line6 = Chart.DrawVerticalLine("6", src.AddMinutes(-6), Color.Blue, 2, LineStyle.DotsRare);
var line7 = Chart.DrawVerticalLine("7", src.AddMinutes(-7), Color.Blue, 2, LineStyle.DotsRare);
}
}
}
Is that what you wanted? Bye
@paolo.panicali
paolo.panicali
28 Jul 2022, 12:28
Have a look to this Indicator
Hi, check this post . Bar.Close won't work for Renko, you need an indicator to handle Renko. Bye
@paolo.panicali
paolo.panicali
18 Jul 2019, 17:13
Thank you Pepper Team, you do a great Job, I am the happiest man ever with Ctrader. i d love you to give some support on Ctrader+Python, it turns out that Convolutional Neural nets performs decently predicting market direction, not the price, i mean feeding the net with multivariate time series(OHLC volume others....some of them). Bottom line, if you could give some help, cz algorithmic trading doesn not work, i mean pure.....AI plus some Algo much better. Thank you you are the best!
@paolo.panicali
paolo.panicali
28 Jul 2022, 16:15 ( Updated at: 21 Dec 2023, 09:22 )
Rsi Breakout Indicator for Renko
Hello not sure if this is what you wanted to do... Plots Rsi tops > of all the previous tops for N bars and in a certain range of RSI (exapmple RSI >=60 and RSI<=90)
For the bottoms just do the opposite...
it seems it also works for other timeframes...
// Rsi Breakout for Renko by paolo panicali 2022
// answer to hamijonzsince: 09 May 2022 06 Jun 2022, 12:11
using System;
using cAlgo.API;
using cAlgo.API.Internals;
using cAlgo.API.Indicators;
using cAlgo.Indicators;
namespace cAlgo
{
[Indicator(IsOverlay = false, TimeZone = TimeZones.UTC, AccessRights = AccessRights.None)]
public class RenkoRsictrader : Indicator
{
[Parameter("Period", Group = "RSI", DefaultValue = 14)]
public int RsiPeriod { get; set; }
[Parameter("Rsi Min Value", Group = "RSI", DefaultValue = 60)]
public int RsiMin { get; set; }
[Parameter("Rsi Max Value", Group = "RSI", DefaultValue = 100)]
public int RsiMax { get; set; }
[Output("Breakout Top Signal", Color = Colors.Aqua, Thickness = 5, PlotType = PlotType.Points)]
public IndicatorDataSeries BreakOutTop { get; set; }
[Output("RSI", Color = Colors.Red, Thickness = 1, PlotType = PlotType.DiscontinuousLine)]
public IndicatorDataSeries Rsi_Value { get; set; }
RelativeStrengthIndex RSI;
double prevRsi_Value, TopRsi_Value, LastRsi_Value;
int LookBack;
IndicatorDataSeries RelativeTop_Value_Series, RelativeTop_Top_Series;
protected override void Initialize()
{
RSI = Indicators.RelativeStrengthIndex(Bars.ClosePrices, RsiPeriod);
RelativeTop_Value_Series = CreateDataSeries();
RelativeTop_Top_Series = CreateDataSeries();
LookBack = 24;
}
public override void Calculate(int index)
{
Rsi_Value[index] = RSI.Result[index];
prevRsi_Value = RSI.Result[index - 2];
TopRsi_Value = RSI.Result[index - 1];
LastRsi_Value = RSI.Result[index];
if (TopRsi_Value > prevRsi_Value && TopRsi_Value > LastRsi_Value)
{
RelativeTop_Top_Series[index - 1] = 1;
RelativeTop_Value_Series[index - 1] = TopRsi_Value;
}
else
{
RelativeTop_Top_Series[index - 1] = 0;
}
if (RelativeTop_Top_Series[index - 1] == 1 && RelativeTop_Value_Series[index - 1] >= RsiMin && RelativeTop_Value_Series[index - 1] <= RsiMax)
{
bool IsBreakout = true;
for (int i = 2; i <= 2 + LookBack; i++)
{
if (RelativeTop_Top_Series[index - i] == 1 && RelativeTop_Value_Series[index - i] > RelativeTop_Value_Series[index - 1])
{
IsBreakout = false;
break;
}
}
if (IsBreakout)
{
BreakOutTop[index - 1] = TopRsi_Value;
}
else
{
//BreakOutTop[index - 1] = 0;
}
}
}
}
}
Hope it ll help, bye.
@paolo.panicali