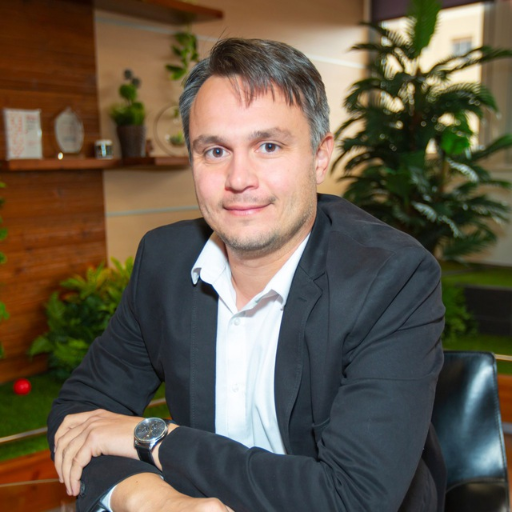
Topics
Replies
PanagiotisCharalampous
04 Mar 2019, 10:35
Hi tmfd,
Without any commitments, we hope to have this during first half of 2019.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
04 Mar 2019, 10:32
Hi Jeziel,
Check the code below
using System; using cAlgo.API; using cAlgo.API.Internals; using cAlgo.API.Indicators; using cAlgo.Indicators; using System.Linq; namespace cAlgo { [Indicator(IsOverlay = true, TimeZone = TimeZones.UTC, AutoRescale = true, AccessRights = AccessRights.None)] public class SistemaImpulsoVersionEstable : Indicator { public MacdHistogram MacdHistogram; [Parameter()] public DataSeries Source { get; set; } [Parameter("Long Cycle", DefaultValue = 26)] public int LongCycle { get; set; } [Parameter("Short Cycle", DefaultValue = 12)] public int ShortCycle { get; set; } [Parameter("Signal Periods", DefaultValue = 9)] public int Periods { get; set; } [Parameter("Ema Period", DefaultValue = 13, MinValue = 1)] public int EmaPeriod { get; set; } private ExponentialMovingAverage Ema { get; set; } [Parameter("Above up color", DefaultValue = "DarkGreen")] public string AboveDownColor { get; set; } [Parameter("Above up color", DefaultValue = "DarkGreen")] public string AboveUpColor { get; set; } [Parameter("Above up color 2", DefaultValue = "Lime")] public string AboveDownColor2 { get; set; } [Parameter("Above up color 2", DefaultValue = "Lime")] public string AboveUpColor2 { get; set; } [Parameter("Below up color", DefaultValue = "DarkRed")] public string BelowUpColor { get; set; } [Parameter("Below down color", DefaultValue = "DarkRed")] public string BelowDownColor { get; set; } [Parameter("Below up color 2", DefaultValue = "OrangeRed")] public string BelowUpColor2 { get; set; } [Parameter("Below down color 2", DefaultValue = "OrangeRed")] public string BelowDownColor2 { get; set; } [Parameter("Neutral up color", DefaultValue = "Gray")] public string NeutralUpColor { get; set; } [Parameter("Neutral down color", DefaultValue = "Gray")] public string NeutralDownColor { get; set; } private Colors _AboveUpColor; private Colors _AboveDownColor; private Colors _AboveUpColor2; private Colors _AboveDownColor2; private Colors _BelowUpColor; private Colors _BelowDownColor; private Colors _BelowUpColor2; private Colors _BelowDownColor2; private Colors _NeutralUpColor; private Colors _NeutralDownColor; private Colors color; private bool _incorrectColors; private Random _random = new Random(); /*------ SMA 200-----------*/ public int Ema200 = 200; private MovingAverage ma200; /*------FIN SMA 200-----------*/ protected override void Initialize() { MacdHistogram = Indicators.MacdHistogram(Source, LongCycle, ShortCycle, Periods); Ema = Indicators.ExponentialMovingAverage(Source, EmaPeriod); ma200 = Indicators.SimpleMovingAverage(Source, Ema200); if (!Enum.TryParse<Colors>(AboveUpColor, out _AboveUpColor) || !Enum.TryParse<Colors>(AboveDownColor, out _AboveDownColor) || !Enum.TryParse<Colors>(BelowUpColor, out _BelowUpColor) || !Enum.TryParse<Colors>(BelowDownColor, out _BelowDownColor) || !Enum.TryParse<Colors>(NeutralUpColor, out _NeutralUpColor) || !Enum.TryParse<Colors>(NeutralDownColor, out _NeutralDownColor) || !Enum.TryParse<Colors>(AboveUpColor2, out _AboveUpColor2) || !Enum.TryParse<Colors>(AboveDownColor2, out _AboveDownColor2) || !Enum.TryParse<Colors>(BelowUpColor2, out _BelowUpColor2) || !Enum.TryParse<Colors>(BelowDownColor2, out _BelowDownColor2)) { _incorrectColors = true; } Chart.ZoomChanged += Chart_ZoomChanged; } private void Chart_ZoomChanged(ChartZoomEventArgs obj) { Print("Zoom Changed"); for (int i = 0; i < MarketSeries.Close.Count; i++) { Refresh(i); } } public void Refresh(int index) { // This update the Candlewidth Variable according to the ZoomChart Value int CandleWidth = 1; switch (Chart.Zoom) { case 0: { CandleWidth = 1; break; } case 1: { CandleWidth = 1; break; } case 2: { CandleWidth = 3; break; } case 3: { CandleWidth = 5; break; } case 4: { CandleWidth = 11; break; } case 5: { CandleWidth = 25; break; } } // This update de color of the color variable according to its condition var open = MarketSeries.Open[index]; var high = MarketSeries.High[index]; var low = MarketSeries.Low[index]; var close = MarketSeries.Close[index]; if (MacdHistogram.Histogram.IsRising() && Ema.Result.IsRising() && MacdHistogram.Histogram.LastValue < 0) { color = open > close ? _AboveDownColor : _AboveUpColor; } if (MacdHistogram.Histogram.IsRising() && Ema.Result.IsRising() && MacdHistogram.Histogram.LastValue > 0) { color = open > close ? _AboveDownColor2 : _AboveUpColor2; } if (MacdHistogram.Histogram.IsFalling() && Ema.Result.IsFalling() && MacdHistogram.Histogram.LastValue > 0) { color = open > close ? _BelowDownColor : _BelowUpColor; } if (MacdHistogram.Histogram.IsFalling() && Ema.Result.IsFalling() && MacdHistogram.Histogram.LastValue < 0) { color = open > close ? _BelowDownColor2 : _BelowUpColor2; } if (MacdHistogram.Histogram.IsRising() && Ema.Result.IsFalling() || MacdHistogram.Histogram.IsFalling() && Ema.Result.IsRising()) { color = open > close ? _NeutralDownColor : _NeutralUpColor; } //Here is printed on the Chart and the CandleWidth take the last value in the switch Chart.DrawTrendLine("candle" + index, index, open, index, close, (Chart.Objects.First(x => x.Name == "candle" + index) as ChartTrendLine).Color, CandleWidth, LineStyle.Solid); Chart.DrawTrendLine("line" + index, index, high, index, low, (Chart.Objects.First(x => x.Name == "line" + index) as ChartTrendLine).Color, 1, LineStyle.Solid); } public override void Calculate(int index) { // This update the Candlewidth Variable according to the ZoomChart Value int CandleWidth = 1; switch (Chart.Zoom) { case 0: { CandleWidth = 1; break; } case 1: { CandleWidth = 1; break; } case 2: { CandleWidth = 3; break; } case 3: { CandleWidth = 5; break; } case 4: { CandleWidth = 11; break; } case 5: { CandleWidth = 25; break; } } // This update de color of the color variable according to its condition var open = MarketSeries.Open[index]; var high = MarketSeries.High[index]; var low = MarketSeries.Low[index]; var close = MarketSeries.Close[index]; if (MacdHistogram.Histogram.IsRising() && Ema.Result.IsRising() && MacdHistogram.Histogram.LastValue < 0) { color = open > close ? _AboveDownColor : _AboveUpColor; } if (MacdHistogram.Histogram.IsRising() && Ema.Result.IsRising() && MacdHistogram.Histogram.LastValue > 0) { color = open > close ? _AboveDownColor2 : _AboveUpColor2; } if (MacdHistogram.Histogram.IsFalling() && Ema.Result.IsFalling() && MacdHistogram.Histogram.LastValue > 0) { color = open > close ? _BelowDownColor : _BelowUpColor; } if (MacdHistogram.Histogram.IsFalling() && Ema.Result.IsFalling() && MacdHistogram.Histogram.LastValue < 0) { color = open > close ? _BelowDownColor2 : _BelowUpColor2; } if (MacdHistogram.Histogram.IsRising() && Ema.Result.IsFalling() || MacdHistogram.Histogram.IsFalling() && Ema.Result.IsRising()) { color = open > close ? _NeutralDownColor : _NeutralUpColor; } //Here is printed on the Chart and the CandleWidth take the last value in the switch ChartObjects.DrawLine("candle" + index, index, open, index, close, color, CandleWidth, LineStyle.Solid); ChartObjects.DrawLine("line" + index, index, high, index, low, color, 1, LineStyle.Solid); } } }
Let me know if this works for you
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 14:45
Hi Glen,
Thanks I have seen the video. Your description was a bit confusing. The objects do not stay on the list for any chart and any symbol. The drawings stay on the list only when you change symbol on the same chart. The chart objects/indicators apply to the chart itself and not to a chart/symbol combination. If you need a clean chart and a separate set of chart objects/indicators, you can create a new chart.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 14:27
Hi Ben,
Not really :) Can we arrange a TeamViewer session so that our QA team can inspect your computer?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 14:14
Hi ryan.a.blake,
When you have time, please share backtesting parameters and results. I need to be able to reproduce exactly what you can see in cTrader.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 12:42
( Updated at: 19 Mar 2025, 08:57 )
Hi Glen,
Send it to support@ctrader.com
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 12:12
Hi Ton,
The issue is not very clear to me. Can you give me exact steps to reproduce it and understand what you mean?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 12:10
Hi Ton,
Immediatelly means on the existing liquidity. If the existing liquidity at the time of filling the order is not adequate to fill the order, then the order not filled is cancelled. It will not wait for new liquidity. Investopedia is a good resource for such definitions.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 11:34
Hi Ben,
When you say nothing happens, do you mean there are no errors or cTrader does not launch? If cTrader does not launch then this is because you probably try to run it from a short cut. To launch cTrader as an admininstrator, you need to find the actual folder and run it from there.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 11:19
Hi Jeziel,
Thanks for posting in our forum. To solve the problem, you need to handle the zoom changing event and redraw your indicator. See below
protected override void Initialize() { MacdHistogram = Indicators.MacdHistogram(Source, LongCycle, ShortCycle, Periods); Ema = Indicators.ExponentialMovingAverage(Source, EmaPeriod); ma200 = Indicators.SimpleMovingAverage(Source, Ema200); if (!Enum.TryParse<Colors>(AboveUpColor, out _AboveUpColor) || !Enum.TryParse<Colors>(AboveDownColor, out _AboveDownColor) || !Enum.TryParse<Colors>(BelowUpColor, out _BelowUpColor) || !Enum.TryParse<Colors>(BelowDownColor, out _BelowDownColor) || !Enum.TryParse<Colors>(NeutralUpColor, out _NeutralUpColor) || !Enum.TryParse<Colors>(NeutralDownColor, out _NeutralDownColor) || !Enum.TryParse<Colors>(AboveUpColor2, out _AboveUpColor2) || !Enum.TryParse<Colors>(AboveDownColor2, out _AboveDownColor2) || !Enum.TryParse<Colors>(BelowUpColor2, out _BelowUpColor2) || !Enum.TryParse<Colors>(BelowDownColor2, out _BelowDownColor2)) { _incorrectColors = true; } Chart.ZoomChanged += Chart_ZoomChanged; } private void Chart_ZoomChanged(ChartZoomEventArgs obj) { //Redraw your indicator }
Let me know if this helps
Best Regards.
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 10:36
Hi rooky06,
Can you explain what does this screener do?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 10:34
Hi Ton,
Server.Time is the time of your broker's server based on the timezone you set for the cBot.
Regarding the order types, both of the examples you posted are IOC orders. It means on triggering the order, fill whatever you can immediately and cancel the rest. On the other hand a FOK orders means fill the order immediately and completely or do not fill it at all. Since all orders in cTrader allow partial execution there is no way to satisfy the completely part of a FOK order.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 10:18
Hi ryan.a.blake,
Can you also provide us with backtesting parameters and backtesting results where we can see such trades? You can post a screenshot of a trade that you think is wrong and explain what would you expect to happen instead.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 09:59
Hi BamsiCBR,
Thank you for contacting Spotware. Subaccounts are visible only in cTrader Copy section. You should be able to see your sub accounts below the main account. Have you checked the cTrader Copy section?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
01 Mar 2019, 09:35
Hi Glen,
Should work the same way for both. I checked on cTrader Web but still seems ok. If you have exact steps to reproduce, please let us know to have a look at this.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
28 Feb 2019, 16:45
Hi Ryan,
Unfortunately the information you provide is too little. We need you to share with us your indicator and cBot and tell us how to reproduce the issue so that we can advise you.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
28 Feb 2019, 15:23
Hi Ben,
Both cAlgo folder and Templates folder are located in the Documents folder. So it seems cTrader does not have sufficient permissions to that folder. However I cannot give you further advise as I do not know what is happening on your pc. Can you please try running cTrader as an admininstrator and let me know if the problems disappear?
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
28 Feb 2019, 15:13
Hi ycomp,
Unfortunately I cannot advise you about Java, I am a .Net guy. However v2 is based on the same technology as v1. It just features more messages and changes to some existing ones. Also I don't think this this is an API issue but a matter of your application architecture. My proposal is to have a connection established all the time which will be used to send and receive messages. You will need to send heartbeats to the server to keep the connection open and have a listener which will listed constantly to incoming messages. If you are familiar with .Net, you can have a look at the .Net Sample.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
28 Feb 2019, 14:41
Hi Max T,
:) Here is what it is.
Best Regards,
Panagiotis
@PanagiotisCharalampous
PanagiotisCharalampous
04 Mar 2019, 10:36
Hi Sasha,
See below
Best Regards,
Panagiotis
@PanagiotisCharalampous