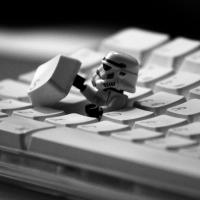
cAlgo Copy Orders with SocketServer
24 Jan 2015, 18:53
cAlgoMaster code example (send pending orders to SocketServer(localhost or vps):
in this example sever on vps (works onStart - You can test in weekend)
using System; using System.Text; using System.Linq; using System.Net; using System.Net.Sockets; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class cAlgoMaster : Robot { [Parameter(DefaultValue = 1, MaxValue = 10)] public int Slot { get; set; } public static string server = "92.222.7.98"; public static string responseData = ""; protected override void OnStart() { Connect(); } protected override void OnBar() { Connect(); } public void Connect() { ///=========================================================== string pos = "SET#" + Slot + "#"; pos = pos + "SPREAD:" + Symbol.Spread + "|"; foreach (var pendingOrder in PendingOrders) { pos = pos + pendingOrder.Id + "|" + pendingOrder.TradeType + "|" + pendingOrder.Volume + "|" + pendingOrder.TargetPrice + "|||"; } // end of stream pos = pos + '\0'; // Process the data sent by the client. Print("String " + pos); ////=========================================================== var name = "Up"; var text = "Send : " + pos.ToString(); var staticPos = StaticPosition.TopRight; var color = Colors.Yellow; ChartObjects.DrawText(name, text, staticPos, color); try { // Create a TcpClient to send and recive message from server. Int32 port = 8888; TcpClient client = new TcpClient(server, port); // Translate the passed message into ASCII and store it as a Byte array. Byte[] data = System.Text.Encoding.ASCII.GetBytes(pos); // Get a client stream for reading and writing. NetworkStream stream = client.GetStream(); // Send the message to the connected TcpServer. stream.Write(data, 0, data.Length); Print("Sent: " + pos); // Buffer to store the response bytes. data = new Byte[1024]; // Read the first batch of the TcpServer response bytes. Int32 bytes = stream.Read(data, 0, data.Length); responseData = System.Text.Encoding.ASCII.GetString(data, 0, bytes); Print("Received: {0}", responseData); // Close everything. stream.Close(); client.Close(); } catch (ArgumentNullException e) { Print("ArgumentNullException: {0}", e); } catch (SocketException e) { Print("SocketException: {0}", e); } } } }
cAlgoSlave get positions fron SocketServer (localhost or vps)
using System; using System.Text; using System.Linq; using System.Net; using System.Net.Sockets; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Internals; using cAlgo.Indicators; namespace cAlgo { [Robot(TimeZone = TimeZones.UTC, AccessRights = AccessRights.FullAccess)] public class cAlgoSlave : Robot { [Parameter(DefaultValue = 1, MaxValue = 10)] public int Slot { get; set; } public static string server = "92.222.7.98"; public static string responseData = ""; protected override void OnStart() { Connect(); } protected override void OnBar() { Connect(); } public void Connect() { ///=========================================================== string pos = "GET#" + Slot + "#"; // end of stream pos = pos + '\0'; try { // Create a TcpClient to send and recive message from server. Int32 port = 8888; TcpClient client = new TcpClient(server, port); // Translate the passed message into ASCII and store it as a Byte array. Byte[] data = System.Text.Encoding.ASCII.GetBytes(pos); // Get a client stream for reading and writing. NetworkStream stream = client.GetStream(); // Send the message to the connected TcpServer. stream.Write(data, 0, data.Length); Print("Sent: " + pos); // Buffer to store the response bytes. data = new Byte[1024]; // Read the first batch of the TcpServer response bytes. Int32 bytes = stream.Read(data, 0, data.Length); responseData = System.Text.Encoding.ASCII.GetString(data, 0, bytes); Print("Received: {0}", responseData); // Process the data sent by the client. var name = "Up"; var text = "Received: " + responseData.ToString(); var staticPos = StaticPosition.TopRight; var color = Colors.Yellow; ChartObjects.DrawText(name, text, staticPos, color); // Close everything. stream.Close(); client.Close(); } catch (ArgumentNullException e) { Print("ArgumentNullException: {0}", e); } catch (SocketException e) { Print("SocketException: {0}", e); } } } }
SocketServer is on vps it allow multi users(Slot = account),
cAlgo Master Accounts (You can send Positions from 10 account in same time)
cAlgo Slave Accounts unlimited (You can choose which account copy)
Simple version (send only text dont set orders)
mindbreaker
27 Jan 2015, 11:56
/algos/cbots/show/654
@mindbreaker