Help on Break Even
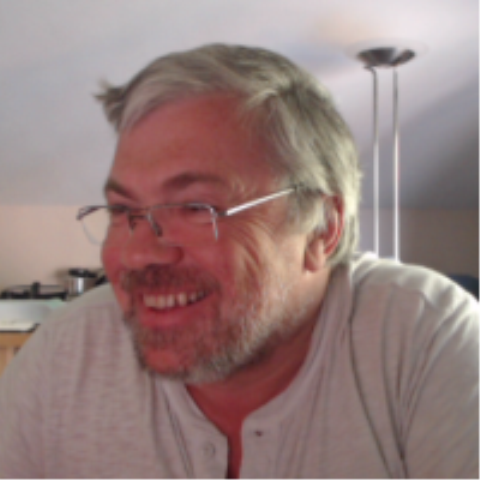
Help on Break Even
27 Jan 2018, 08:14
My bot is moving a Stop Loss to breakeven
The problem: it does fine for "Sell" orders, but shows an error for "Buy" orders.
Can anybody help?
here is the code:
using System.Linq;
using cAlgo.API;
using cAlgo.API.Indicators;
using cAlgo.API.Internals;
/// <summary>
/// This is a doubling the lot bot, with the stoploss halfed after the double
/// today the 20 th of Jan 2018 I am adding a breakeven to it
/// </summary>
namespace cAlgo
{
#region parameters
[Robot(TimeZone = TimeZones.RussianStandardTime, AccessRights = AccessRights.FullAccess)]
public class StartAChain : Robot
{
[Parameter(DefaultValue = 100000)]
public int LotSize { get; set; }
[Parameter(DefaultValue = 10.0)]
public double SL { get; set; }
[Parameter(DefaultValue = 10.0)]
public double TP { get; set; }
[Parameter("Instance Name", DefaultValue = "001")]
public string InstanceName { get; set; }
[Parameter("Source SMA #1")]
public DataSeries SourceSma1 { get; set; }
[Parameter("Period SMA #1", DefaultValue = 5, MinValue = 1, MaxValue = 100)]
public int PeriodsSma1 { get; set; }
[Parameter()]
private int currentLot { get; set; }
[Parameter()]
private double currentStop { get; set; }
[Parameter("Include Break-Even", DefaultValue = false)]
public bool IncludeBreakEven { get; set; }
[Parameter("Break-Even Trigger (pips)", DefaultValue = 10, MinValue = 1)]
public int Trigger { get; set; }
[Parameter("Break-Even Extra (pips)", DefaultValue = 2, MinValue = 0)]
public int BreakEvenExtraPips { get; set; }
private TradeType _tradeType;
#endregion
#region Indicator declarations
private SimpleMovingAverage _sma1 { get; set; }
public bool Buy { get; private set; }
#endregion
#region cTrader events
/// <summary>
/// This is called when the robot first starts, it is only called once.
/// </summary>
protected override void OnStart()
{
// construct the indicators
_sma1 = Indicators.SimpleMovingAverage(SourceSma1, PeriodsSma1);
currentLot = LotSize;
currentStop = SL;
// Positions.Closed += positionsOnClosed;
_tradeType = Buy ? TradeType.Buy : TradeType.Sell;
}
protected override void OnTick()
{
ManagePositions();
if (IncludeBreakEven == true)
GoToBreakEven();
}
protected override void OnStop()
{
// unused
}
#endregion
#region Manage Positions
private void ManagePositions()
{
if (!IsPositionOpenByType(TradeType.Buy) && !IsPositionOpenByType(TradeType.Sell))
{
if (_sma1.Result.LastValue < Symbol.Ask)
{
ExecuteMarketOrder(TradeType.Buy, Symbol, currentLot, InstanceName, currentStop, TP);
}
else
{
ExecuteMarketOrder(TradeType.Sell, Symbol, currentLot, InstanceName, currentStop, TP);
}
}
}
private bool IsPositionOpenByType(TradeType type)
{
var p = Positions.FindAll(InstanceName, Symbol, type);
if (p.Count() >= 1)
return true;
return false;
}
#endregion
#region Break Even
private void GoToBreakEven()
{
var position = Positions.Find(InstanceName, Symbol);
var entryPrice = position.EntryPrice;
var distance = _tradeType != TradeType.Sell ? Symbol.Bid - entryPrice : entryPrice - Symbol.Ask;
Print("distance {0} ", distance);
if (distance >= Trigger * Symbol.PipSize)
ModifyPosition(position, entryPrice, position.TakeProfit);
}
#endregion
}
}
Replies
PanagiotisCharalampous
29 Jan 2018, 12:35
Hi Alexander,
The problem lies in the following line of code
var distance = _tradeType != TradeType.Sell ? Symbol.Bid - entryPrice : entryPrice - Symbol.Ask;
The _tradeType is always Sell even if the open position is Buy. Can you explain to us what are you trying to achieve here?
Best Regards,
Panagiotis
@PanagiotisCharalampous
alexander.n.fedorov
29 Jan 2018, 13:43
I am new to C#. I was trying to use what I thought would be the normal shortcut for bunch of if.
Thank you very much for your reply. It is really a pleasure to recieve good service
I already figured out how to if (longer way)
Regards
@alexander.n.fedorov
alexander.n.fedorov
28 Jan 2018, 16:04
RE: I am still curious if anybody can help, please?
alexander.n.fedorov said:
@alexander.n.fedorov