Topics
Replies
firemyst
23 Jan 2024, 09:51
( Updated at: 23 Jan 2024, 12:55 )
Hello Team @Spotware / @PanagiotisCharalampous
Any further updates on a fix for this issue?
Thank you
@firemyst
firemyst
19 Jan 2024, 08:21
You can add the SL/TP columns in the “Trade watch” window under the chart if you're viewing that;
when you place an order, the SL and TP lines are on the chart, or can be dragged from the order's entry point to show on the chart.
So no idea what are you talking about???
@firemyst
firemyst
13 Jan 2024, 13:47
( Updated at: 15 Jan 2024, 07:11 )
My first suggestion is to post screen capture(s) showing the result of the build, because right now, for all we know you could be doing it in Visual Studio, VS Code, cTRader itself, or some other way.
How are people supposed to know to be able to assist with the little info you provided?
@firemyst
firemyst
13 Jan 2024, 13:41
( Updated at: 15 Jan 2024, 07:11 )
You need to post this in the Suggestions forum if you would like this to be considered:
https://ctrader.com/forum/suggestions
@firemyst
firemyst
13 Jan 2024, 13:40
( Updated at: 15 Jan 2024, 07:11 )
THis doesn't belong here.
You need to post it in the Suggestions forum:
https://ctrader.com/forum/suggestions
@firemyst
firemyst
11 Jan 2024, 09:03
RE: RE: RE: RE: RE: cTrader "pip scale" constantly goes flukey, and nowhere near being correct
PanagiotisCharalampous said:
firemyst said:
PanagiotisCharalampous said:
Hi firemyst,
Our team is still investigating this issue. Can you please send us the template you are using at 00:29 of the video?
Best regards,
Panagiotis
Sent as requested to the “community” address with subject ATTN PANAGIOTIS
Hopefully it'll help the team figure out what's going on.
Hi firemyst,
Thank you. Unfortunately we were not being able to reproduce this yet. But we will keep trying.
Best regards,
Panagiotis
I've done it again with another template file.
I've made a new video as well showing a bit more and talking through it so your team knows exactly what I'm doing and when.
When the video finishes uploading to YouTube, I'll email the “community” account with the new video link and template file that I used this time around.
@firemyst
firemyst
10 Jan 2024, 11:40
RE: RE: RE: cTrader "pip scale" constantly goes flukey, and nowhere near being correct
PanagiotisCharalampous said:
Hi firemyst,
Our team is still investigating this issue. Can you please send us the template you are using at 00:29 of the video?
Best regards,
Panagiotis
Sent as requested to the “community” address with subject ATTN PANAGIOTIS
Hopefully it'll help the team figure out what's going on.
@firemyst
firemyst
10 Jan 2024, 07:59
RE: cTrader "pip scale" constantly goes flukey, and nowhere near being correct
PanagiotisCharalampous said:
Hi firemyst,
Any chance you can provide us with more clear images? I cannot see the pip scale in these images.
Best regards,
Panagiotis
Any updates on this? Have they discovered the issue? It's getting to be real annoying.
Check out the GER40 today in multichart mode.
You can see the same numbers on the right side of both charts, but notice how one chart is screwed and says “100” pips while the other is correct and says “10” pips based on the values:

@firemyst
firemyst
09 Jan 2024, 08:11
RE: Does cTrader support algos built under .Net8.0 yet?
PanagiotisCharalampous said:
Hi firemyst,
We have no plans for introducing support for .Net 8.0 any time soon.
Best regards,
Panagiotis
Fair enough on that.
However, what about in regards to my other questions?
@firemyst
firemyst
07 Jan 2024, 02:52
RE: RE: Here are some tips to get you started
YesOrNot said:
Hi Hi! Thanks for the help. I just made it, but it doesn't deserve. I think the problem is plotting points, not the memory operations…
Great to see the big code improvements!
That's just the start as I said.
cTrader wasn't built well enough to handle all the custom drawn objects like other trading platforms.
What I've done as a work around is included parameters in my indicators to state how far back I want items drawn:
[Parameter("Num of Bars Back to Analyze", Group = "Misc", DefaultValue = 500, MinValue = 1)]
public int NumBarsBackToAnalyze { get; set; }
Then what I do is every time a new bar is formed, I programmatically remove all the custom drawn objects that are more than “NumBarsBackToAnalyze
”.
So in the example above, when a new bar is formed, if I have any custom drawn objects on the chart more than 500 bars ago, I delete them.
It's a rolling process, keeps cTrader somewhat responsive. However, because it's a heavy burden, I only do it once every time a new bar is formed – it's a waste of resources to do it every tick.
@firemyst
firemyst
06 Jan 2024, 11:00
I'm not sure you're referencing the symbol correct. None of the symbols I've ever seen in cTrader start with “#”.
Also, have you checked your broker's website to see what the symbol is?
Last, what's the symbol when you view the trading window in cTrader? You can search for all the symbols cTrader will let you trade.
@firemyst
firemyst
05 Jan 2024, 08:08
RE: cTrader "pip scale" constantly goes flukey, and nowhere near being correct
PanagiotisCharalampous said:
Hi firemyst,
Any chance you can provide us with more clear images? I cannot see the pip scale in these images.
Best regards,
Panagiotis
I've just managed to reproduce the issue again. I will submit the Youtube link using cTrader's “Report a technical” issue right now, while the scaling is messed up, referencing this forum discussion.
@firemyst
firemyst
05 Jan 2024, 07:24
RE: cTrader "pip scale" constantly goes flukey, and nowhere near being correct
PanagiotisCharalampous said:
Hi firemyst,
Any chance you can provide us with more clear images? I cannot see the pip scale in these images.
Best regards,
Panagiotis
How much more clear do you want? If you open the images in a “new tab” on your browser and/or download them and then zoom in with the Microsoft image viewer, the market value on the right is quite clear.
I've done just that now and here they are (first one is NAS, the last two are the FTSE100 before and after restarting cTrader) :
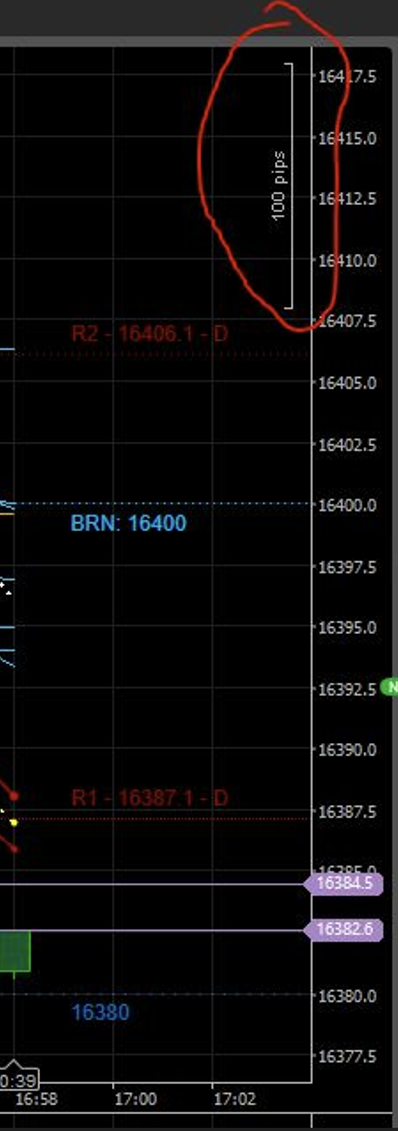
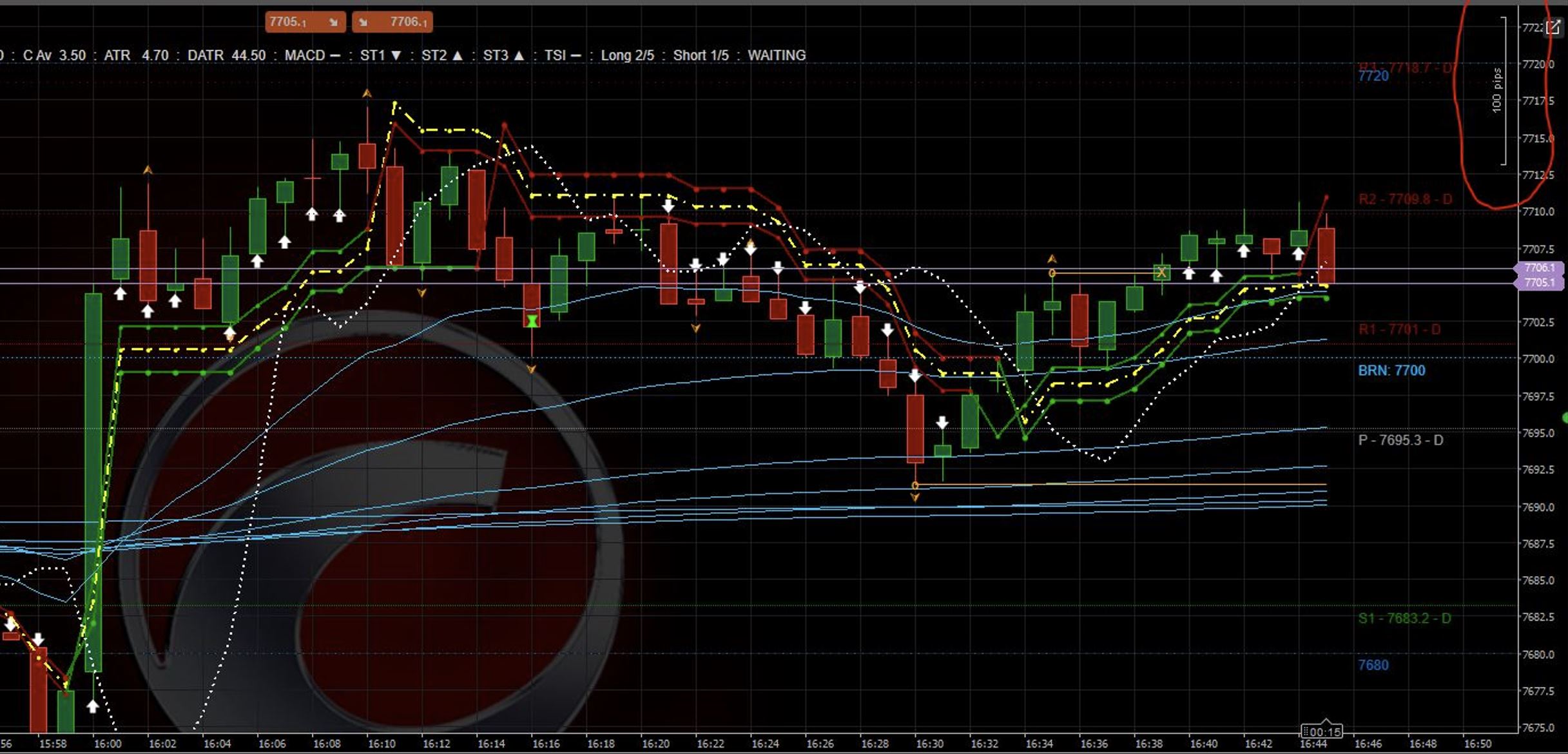
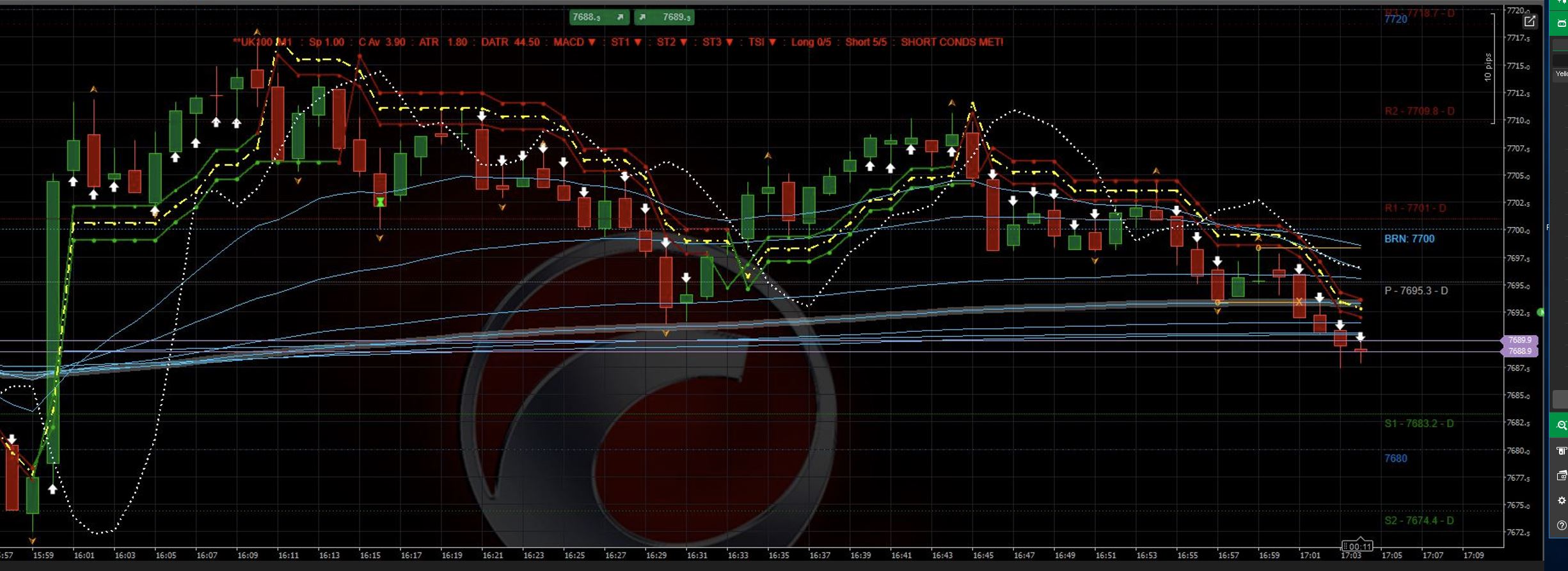
All times are UTC + 8
@firemyst
firemyst
30 Dec 2023, 12:00
( Updated at: 31 Dec 2023, 16:08 )
RE: RE: Multiple cbots and only one position at a time
The_Dark_Knight said:
firemyst said:
If you don't want positions of the same symbol to overlap, then you need to find all positions first of the current symbol, and then doing a Positions.Count on that.
Doing a generic “Positions.Count” will count all open positions, even if you have several not open on the current symbol your bot instance is running.
For example, you could have an AUDUSD position open, a EURUSD position open, and EURJPY position open. If you only want to limit your bot so that you don't open another EURJPY position, then you need to search all positions that are just EURJPY.
Example:
int numPositionsAlreadyOpen = Positions.FindAll(p.Label, Symbol.Name).Count();
Hope that helps you even more :-)
Thanks Firemyst, that's also very helpful. I found something like that in the forum.
For now, I will apply this logic by grouping the long and short positions separately, in order to decrease exposure at times where the pairs are correlated. According to the results of QuantAnalyzer, this would greatly soften the portfolio curve, but I don't know how much to trust those hypothetical results. I'll try it first in demo.
I added it like this in each cbot. Please correct me if that´s wrong:
protected override void OnBar()
{var longPositionsCount = Positions.Count(p => p.TradeType == TradeType.Buy);
var shortPositionsCount = Positions.Count(p => p.TradeType == TradeType.Sell);
if (longPositionsCount == 0 && ShouldEnterLong()) { logic }
if (ShouldExitLong()) {CloseLongPositions();}
if (shortPositionsCount == 0 && ShouldEnterShort()) { logic }
if (ShouldExitShort()) {CloseShortPositions();}
}
I can't tell you if it's right or wrong because I'm not 100% sure what you want to do.
What your code will do though is found the number of buy positions and sell positions open across all active bot instances and won't open a long position for any symbol if you have a long position open anywhere in any account; likewise your code won't open a short position for any symbol if you have a short position open anywhere.
@firemyst
firemyst
30 Dec 2023, 10:07
( Updated at: 31 Dec 2023, 16:08 )
You're doing nothing wrong. “Position” is defined as an abstract interface in cAlgo:
https://help.ctrader.com/ctrader-automate/references/Trading/Positions/Position/
In the process of Deserialization, it involves creating an instance, and you can't create instances of interfaces.
Here's a blog article with a long way around the issue if you really need to do that:
https://dotnetfalcon.com/deserialize-into-interface/
@firemyst
firemyst
30 Dec 2023, 09:54
If you don't want positions of the same symbol to overlap, then you need to find all positions first of the current symbol, and then doing a Positions.Count on that.
Doing a generic “Positions.Count” will count all open positions, even if you have several not open on the current symbol your bot instance is running.
For example, you could have an AUDUSD position open, a EURUSD position open, and EURJPY position open. If you only want to limit your bot so that you don't open another EURJPY position, then you need to search all positions that are just EURJPY.
Example:
int numPositionsAlreadyOpen = Positions.FindAll(p.Label, Symbol.Name).Count();
Hope that helps you even more :-)
@firemyst
firemyst
28 Jan 2024, 11:55
What kind of text?
You don't say what kind of text you're wanting to align.
If you mean from the Chart.DrawText method, here's an example for you:
@firemyst