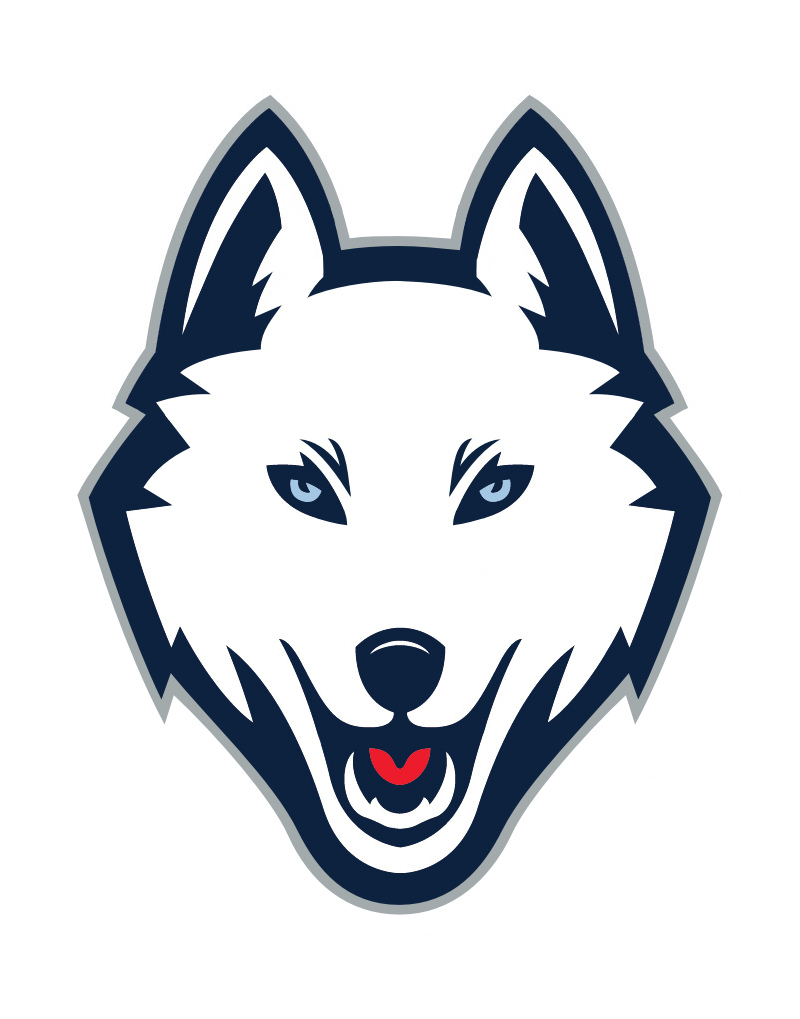
Topics
Replies
breakermind
17 Aug 2013, 13:51
RE:
kricka said:
Hi Spotware,
maybe you can give me the correct code on my question how to count and print total Sell positions to the log?
Thanks..
Hi
Tested count positions and print all positions number to log
Bye
// ------------------------------------------------------------------------------- // // This is a Template used as a guideline to build your own Robot. // // ------------------------------------------------------------------------------- using System; using cAlgo.API; using cAlgo.API.Indicators; using cAlgo.API.Requests; using cAlgo.Indicators; namespace cAlgo.Robots { [Robot(TimeZone = TimeZones.UTC)] public class Robo : Robot { //================================================================================ // Parametrs //================================================================================ private Position _position; [Parameter(DefaultValue = 10000, MinValue = 1000)] public int Volume { get; set; } //================================================================================ // OnStart //================================================================================ protected override void OnStart() { // delete if You dont need add positions on start Buy(); Buy(); Sell(); Sell(); Sell(); Buy(); Buy(); Buy(); Sell(); Sell(); Sell(); Sell(); Sell(); } //================================================================================ // OnTick //================================================================================ protected override void OnTick() { } //================================================================================ // OnBar //================================================================================ protected override void OnBar() { } //================================================================================ // OnPositionOpened //================================================================================ protected override void OnPositionOpened(Position openedPosition) { int BuyPos = 0; int SellPos = 0; foreach (var position in Account.Positions) { if (position.TradeType == TradeType.Buy) { BuyPos = BuyPos + 1; //or // BuyPos++; } if (position.TradeType == TradeType.Sell) { SellPos = SellPos + 1; //or // SellPos++; } } Print("All Buy positions: " + BuyPos); Print("All Sell positions: " + SellPos); } // end OnPositionOpened //================================================================================ // OnPositionClosed //================================================================================ protected override void OnPositionClosed(Position closedPosition) { } //================================================================================ // OnStop //================================================================================ protected override void OnStop() { } //================================================================================ // Function //================================================================================ private void Buy() { Trade.CreateBuyMarketOrder(Symbol, Volume); } private void Sell() { Trade.CreateSellMarketOrder(Symbol, Volume); } private void ClosePosition() { if (_position != null) { Trade.Close(_position); _position = null; } } //================================================================================ // Robot end //================================================================================ } }
@breakermind
breakermind
08 Aug 2013, 01:16
RE:
iRobot said:
Hi,
this is my stoploss/takeprofit condition. Stoploss must have Lower value for Long position and Upper value for Short position. I don't know how to implement that. In a given code stoploss has only one codition when position is opened.
protected override void OnPositionOpened(Position openedPosition)
{
_position = openedPosition;
double? stopLoss = LowerValue;
double? takeProfit = _position.EntryPrice + Symbol.PipSize*TakeProfit;
Trade.ModifyPosition(openedPosition, stopLoss, takeProfit);}
How could I have Upper and Lower stop loss depending if position is Short or Long.
Thanks.
Hi,
Prawdopodobnie co? takiego je?eli dobrze zrozumia?em i mo?na stawia? stopllos poni?ej warto?ci otwarcia pozycji,
je?eli nie to wtedy stop los zast?pi? trzeba takeprofitem
protected override void OnPositionOpened(Position openedPosition)
{
position = openedPosition;
foreach (var position in Account.Positions)
{
double LowerValue = 10;
double UpperValue = 20;
if(position.TradeType == TradeType.Buy){
double? stopLoss = position.EntryPrice - Symbol.PipSize*LowerValue;
double? takeProfit = position.EntryPrice + Symbol.PipSize*TakeProfit;
Trade.ModifyPosition(position, stopLoss, takeProfit);
}
if(position.TradeType == TradeType.Sell){
double? stopLoss = position.EntryPrice + Symbol.PipSize*UpperValue;
double? takeProfit = position.EntryPrice - Symbol.PipSize*TakeProfit;
Trade.ModifyPosition(position, stopLoss, takeProfit);
}
}
}
je?eli czego? nie pomyli?em nie testowa?em
Pozdrawiam
Ps. if You do not understant
http://translate.google.com/
language PL
@breakermind
breakermind
26 Jul 2013, 10:36
RE:
Hi,
tried to write the code to count and print only sell positions to the log, even if I have buy positions as well.
Hope you can help me with this.
Thanks..
Hi,
maybe something like this
public int sell;
sell = 0;
foreach (var position in Account.Positions)
{
if (position.TradeType == TradeType.Sell)
{
sell = sell +1;
}
}
Print("Sell positions: ");
Print(sell);
I have not tested this code.
if something you do not know to find search for answers in the finished robots
Regards
@breakermind
breakermind
22 Jul 2013, 18:31
RE:
What do you want OpenPrice to be set to? Most probably you are assigning a NaN value to it if the indexing is not right. This could result if lastIndex is smaller than backBars.
You may use Print statements throughout the code, to output the values assigned to variables that are used in the conditions that open/close trades, for instance. This way, you can identify what could be wrong.
Please be advised that the drawing of lines, has not been implemented for backtesting.
I would like to OpenPrice contain the value "Open" the first bar of the day (week).
Or simply how to get the value of the first bar of the Monday (Open High Low) when i start script on second day of week?
I use Print but sometimes shows some strange messages.
@breakermind
breakermind
19 Jul 2013, 17:33
RE:
On the page
breakermind.com
You can see how the strategy works on MT4 (video),
and how much earns,
but it is only tester.
In two months the testing strategy with $ 5,000 earned more than $ 100 000.
On demo account (MT4 vps server 24h) playing for 10 days earned more than 100%
( on two different accounts Fxpro.com MT4 (150% Start Position Volume 10000) one, the second (100%) FXcc.com MT4 Start Position Volume 1000)
Regards
@breakermind
breakermind
19 Jul 2013, 15:49
Hello,
I would like to convert MT4 strategy.
Is there somewhere a conwerter of Mt4 to cAlgo?
Or maybe someone is bored and would have the time and knowledge to do this?
MT4 Robot file here:
breakermind.com/breakermind-dayline.mq4
Regards
@breakermind
breakermind
17 Jul 2013, 20:35
RE:
Hello,
I need the equivalent function of MT4
robot operates a period M1 !!!//Openday price
double Dayline = iOpen (NULL, PERIOD_D1, 0);//Closeday price
double DaylineClose = iClose (NULL, PERIOD_D1, 0);//Create line
ObjectCreate ("Dayline" OBJ_HLINE, 0, Time [0], Dayline);// high bars
iHigh (NULL, PERIOD_D1, 0);
Hours ()
Minutes ()
Seconds ()
Surely the answer is trivial, but I can not be traced in the documentation.
Thank you in advance and
Regards
It is no longer valid.
/ / When M1 - first day bar open price
int minute = Server.Time.Minute;
int hour = Server.Time.Hour;
int backBars = hour * 60 + minute;
var lastIndex = MarketSeries.Open.Count;
double OpenPriceDay = MarketSeries.Open [lastIndex-backBars-1];
Regards and bye.
@breakermind
breakermind
17 Jul 2013, 19:47
RE:
so in need only this:
var netProfit = 0.0; foreach (var openedPosition in Account.Positions) { netProfit += openedPosition.NetProfit; } if(Account.Equity > Equity) { foreach (var openedPosition in Account.Positions) { Trade.Close(ALL THE POSITION; PLEASE DARLING ROBOT CLOSE ALL; PLEASE!!!!!); Stop(); }
but so don't work hahahaah
Welcome back,
I do not think you tested what I wrote :) because you knew It close all position !!!
First learn what these two characters / / do.
Regards and bye.
@breakermind
breakermind
17 Jul 2013, 17:50
RE:
Hi i will that my robot close all the opened position if the equity is XXX (parameter).
I have find this:
var netProfit = 0.0; foreach (var openedPosition in Account.Positions) { netProfit += openedPosition.NetProfit; } if(Account.Equity > Equity) { foreach (var openedPosition in Account.Positions) { Trade.Close(openedPosition); Stop(); }but so the robot close only one trade, the oldest, them shut down himself properly, but al trade are open.
I searched a lot on the site but did not find anything.Can someone help me?
Thanks and Regards
Hi
it works for me
protected override void OnPositionOpened(Position openedPosition)
{
if(Account.Equity > 1){
foreach (var position in Account.Positions)
{
//if (position.GrossProfit > 0)
//{
Trade.Close(position);
//}
}
}
}
Regards
@breakermind
breakermind
17 Aug 2013, 16:52 ( Updated at: 21 Dec 2023, 09:20 )
RE:
breakermind said:
Earn in one week
but is only test version.
Bye
@breakermind